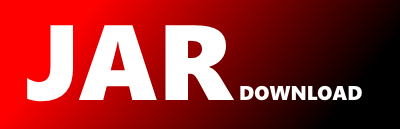
io.annot8.api.properties.Properties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of annot8-api Show documentation
Show all versions of annot8-api Show documentation
Core API interfaces for the Annot8 project
/* Annot8 (annot8.io) - Licensed under Apache-2.0. */
package io.annot8.api.properties;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Optional;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/** Base properties interface from which all other properties objects/interfaces extend. */
public interface Properties {
static boolean equals(Properties a, Properties b) {
return a.getAll().equals(b.getAll());
}
static int hashCode(Properties properties) {
return properties.getAll().hashCode();
}
/**
* Check if a key exists
*
* @param key the key
* @return true if a property with the given key exists
*/
default boolean has(final String key) {
return getAll().containsKey(key);
}
/**
* Check if key existing and its value is of a specific class
*
* @param key the key
* @param clazz class the value must be assignable to
* @return true if a property of the given class with the given key exists
*/
default boolean has(final String key, final Class> clazz) {
return getAll(clazz).containsKey(key);
}
/**
* Get the proprety value
*
* @param key the key
* @return the property value for the specified key, if it exists
*/
default Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy