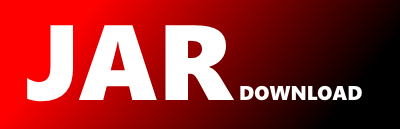
io.annot8.api.stores.GroupStore Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of annot8-api Show documentation
Show all versions of annot8-api Show documentation
Core API interfaces for the Annot8 project
/* Annot8 (annot8.io) - Licensed under Apache-2.0. */
package io.annot8.api.stores;
import io.annot8.api.annotations.Group;
import io.annot8.api.data.Item;
import io.annot8.api.filters.Filter;
import io.annot8.api.helpers.WithFilter;
import java.util.Collection;
import java.util.Optional;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/** Base groups interface from which all other group stores extend. */
public interface GroupStore extends WithFilter {
/**
* Get the item to which the groups relate.
*
* @return item
*/
Item getItem();
/**
* Create a new group builder
*
* @return a builder object for the supported group
*/
Group.Builder getBuilder();
/**
* Create a new group
*
* @return a builder to createContent a new group
*/
default Group.Builder create() {
return getBuilder();
}
/**
* Clone an existing group to createContent a new group
*
* @param existing the existing group
* @return a builder to based on the an existing group, but don't overwrite that group on save.
*/
default Group.Builder copy(Group existing) {
return getBuilder().from(existing).newId();
}
/**
* Edit an existing group, saving will replace it
*
* @param existing the existing group
* @return a builder to edit an existing group
*/
default Group.Builder edit(Group existing) {
return getBuilder().from(existing);
}
/**
* Delete a group from the store
*
* @param group to delete
*/
void delete(final Group group);
/**
* Delete a collection of groups from the store
*
* @param groups the groups to delete
*/
default void delete(final Collection groups) {
groups.forEach(this::delete);
}
/** Delete all groups from the store */
default void deleteAll() {
delete(getAll().collect(Collectors.toList()));
}
/**
* Get all groups
*
* @return all groups currently held in this store
*/
Stream getAll();
/**
* Get all groups of a given type currently held in this store
*
* @param type the type to filter on
* @return the groups of that types
*/
default Stream getByType(final String type) {
return getAll().filter(a -> type.equals(a.getType()));
}
/**
* Get the group with the given ID, if it is currently held in this store
*
* @param groupId the id
* @return the group
*/
Optional getById(final String groupId);
/**
* Filter annotations to match the test.
*
* @param filter the test to filter with
* @return stream of matching annotations
*/
default Stream filter(Filter filter) {
return getAll().filter(filter::test);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy