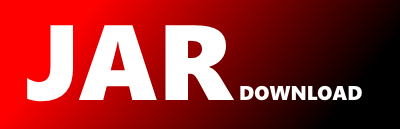
io.annot8.common.data.bounds.PositionBounds Maven / Gradle / Ivy
Show all versions of annot8-common-data Show documentation
/* Annot8 (annot8.io) - Licensed under Apache-2.0. */
package io.annot8.common.data.bounds;
import io.annot8.api.bounds.Bounds;
import io.annot8.api.data.Content;
import io.annot8.api.exceptions.InvalidBoundsException;
import jakarta.json.bind.annotation.JsonbCreator;
import jakarta.json.bind.annotation.JsonbProperty;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.sound.sampled.AudioInputStream;
/**
* A position marker within a content.
*
* Currently supports Content type of String, Object[] or List<Object> (or their subtypes)
*/
public class PositionBounds implements Bounds {
private final int position;
/**
* New position
*
* @param position offset at least 0
*/
@JsonbCreator
public PositionBounds(@JsonbProperty("position") int position) {
if (position < 0) {
throw new InvalidBoundsException("Position must be greater than or equal to 0");
}
this.position = position;
}
/**
* Get the position offset
*
* @return the offset
*/
public int getPosition() {
return position;
}
@Override
@SuppressWarnings("unchecked") // All casts are checked below
public , R> Optional getData(C content, Class requiredClass) {
if (position < 0) {
return Optional.empty();
}
D data = content.getData();
if (data.getClass().isArray()) {
Object[] objArr = (Object[]) data;
if (position < objArr.length && requiredClass.isAssignableFrom(objArr[position].getClass())) {
return Optional.of((R) objArr[position]);
}
} else if (data instanceof List) {
List