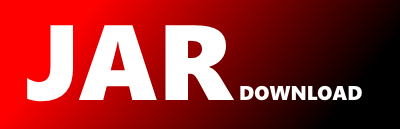
io.annot8.common.implementations.content.AbstractContentBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of annot8-common-implementations Show documentation
Show all versions of annot8-common-implementations Show documentation
Common functionality used by Annot8 implementations
/* Annot8 (annot8.io) - Licensed under Apache-2.0. */
package io.annot8.common.implementations.content;
import java.util.UUID;
import java.util.function.Supplier;
import io.annot8.common.implementations.properties.MapImmutableProperties;
import io.annot8.common.implementations.stores.SaveCallback;
import io.annot8.core.data.Content;
import io.annot8.core.exceptions.IncompleteException;
import io.annot8.core.properties.ImmutableProperties;
import io.annot8.core.properties.Properties;
public abstract class AbstractContentBuilder>
implements Content.Builder {
private final SaveCallback saver;
private final ImmutableProperties.Builder properties = new MapImmutableProperties.Builder();
private String name;
private String id;
private Supplier data;
public AbstractContentBuilder(SaveCallback saver) {
this.saver = saver;
}
@Override
public Content.Builder withId(String id) {
this.id = id;
return this;
}
@Override
public Content.Builder withName(String name) {
this.name = name;
return this;
}
@Override
public Content.Builder withData(Supplier data) {
this.data = data;
return this;
}
@Override
public Content.Builder from(C from) {
return this;
}
@Override
public Content.Builder withProperty(String key, Object value) {
properties.withProperty(key, value);
return this;
}
@Override
public Content.Builder withProperties(Properties properties) {
this.properties.withProperties(properties);
return this;
}
@Override
public Content.Builder withoutProperty(String key, Object value) {
properties.withoutProperty(key, value);
return this;
}
@Override
public Content.Builder withoutProperty(String key) {
properties.withoutProperty(key);
return this;
}
@Override
public C save() throws IncompleteException {
if (id == null) {
id = UUID.randomUUID().toString();
}
if (name == null) {
throw new IncompleteException("Name is required");
}
if (data == null) {
throw new IncompleteException("Data supplier is required");
}
C content = create(id, name, properties.save(), data);
if (saver == null) {
return content;
}
return saver.save(content);
}
protected abstract C create(
String id, String name, ImmutableProperties properties, Supplier data);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy