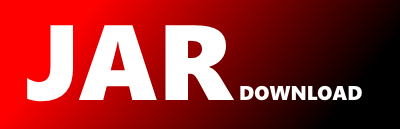
io.antmedia.rest.model.AppSettingsModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ant-media-server Show documentation
Show all versions of ant-media-server Show documentation
Ant Media Server supports RTMP, RTSP, MP4, HLS, WebRTC, Adaptive Streaming, etc.
package io.antmedia.rest.model;
import java.util.List;
import io.antmedia.EncoderSettings;
public class AppSettingsModel {
private boolean mp4MuxingEnabled;
private boolean addDateTimeToMp4FileName;
private boolean hlsMuxingEnabled;
private boolean objectDetectionEnabled;
private int hlsListSize;
private int hlsTime;
private String hlsPlayListType;
private String facebookClientId;
private String facebookClientSecret;
private String youtubeClientId;
private String youtubeClientSecret;
private String periscopeClientId;
private String periscopeClientSecret;
private boolean acceptOnlyStreamsInDataStore;
private List encoderSettings;
private String vodFolder;
private boolean previewOverwrite;
public boolean isMp4MuxingEnabled() {
return mp4MuxingEnabled;
}
public void setMp4MuxingEnabled(boolean mp4MuxingEnabled) {
this.mp4MuxingEnabled = mp4MuxingEnabled;
}
public boolean isAddDateTimeToMp4FileName() {
return addDateTimeToMp4FileName;
}
public void setAddDateTimeToMp4FileName(boolean addDateTimeToMp4FileName) {
this.addDateTimeToMp4FileName = addDateTimeToMp4FileName;
}
public boolean isHlsMuxingEnabled() {
return hlsMuxingEnabled;
}
public void setHlsMuxingEnabled(boolean hlsMuxingEnabled) {
this.hlsMuxingEnabled = hlsMuxingEnabled;
}
public boolean isObjectDetectionEnabled() {
return objectDetectionEnabled;
}
public void setObjectDetectionEnabled(boolean objectDetectionEnabled) {
this.objectDetectionEnabled = objectDetectionEnabled;
}
public int getHlsListSize() {
return hlsListSize;
}
public void setHlsListSize(int hlsListSize) {
this.hlsListSize = hlsListSize;
}
public int getHlsTime() {
return hlsTime;
}
public void setHlsTime(int hlsTime) {
this.hlsTime = hlsTime;
}
public String getHlsPlayListType() {
return hlsPlayListType;
}
public void setHlsPlayListType(String hlsPlayListType) {
this.hlsPlayListType = hlsPlayListType;
}
public String getFacebookClientId() {
return facebookClientId;
}
public void setFacebookClientId(String facebookClientId) {
this.facebookClientId = facebookClientId;
}
public String getFacebookClientSecret() {
return facebookClientSecret;
}
public void setFacebookClientSecret(String facebookClientSecret) {
this.facebookClientSecret = facebookClientSecret;
}
public String getYoutubeClientId() {
return youtubeClientId;
}
public void setYoutubeClientId(String youtubeClientId) {
this.youtubeClientId = youtubeClientId;
}
public String getYoutubeClientSecret() {
return youtubeClientSecret;
}
public void setYoutubeClientSecret(String youtubeClientSecret) {
this.youtubeClientSecret = youtubeClientSecret;
}
public String getPeriscopeClientId() {
return periscopeClientId;
}
public void setPeriscopeClientId(String periscopeClientId) {
this.periscopeClientId = periscopeClientId;
}
public String getPeriscopeClientSecret() {
return periscopeClientSecret;
}
public void setPeriscopeClientSecret(String periscopeClientSecret) {
this.periscopeClientSecret = periscopeClientSecret;
}
public boolean isAcceptOnlyStreamsInDataStore() {
return acceptOnlyStreamsInDataStore;
}
public void setAcceptOnlyStreamsInDataStore(boolean acceptOnlyStreamsInDataStore) {
this.acceptOnlyStreamsInDataStore = acceptOnlyStreamsInDataStore;
}
public List getEncoderSettings() {
return encoderSettings;
}
public void setEncoderSettings(List encoderSettings) {
this.encoderSettings = encoderSettings;
}
public String getVodFolder() {
return vodFolder;
}
public void setVodFolder(String vodFolder) {
this.vodFolder = vodFolder;
}
public boolean isPreviewOverwrite() {
return previewOverwrite;
}
public void setPreviewOverwrite(boolean previewOverwrite) {
this.previewOverwrite = previewOverwrite;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy