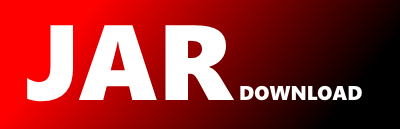
io.ap4k.option.config.JvmConfigFluentImpl Maven / Gradle / Ivy
The newest version!
package io.ap4k.option.config;
import io.ap4k.kubernetes.config.ConfigurationFluentImpl;
import java.lang.Object;
import io.ap4k.option.annotation.SecureRandomSource;
import java.lang.Boolean;
import io.ap4k.option.annotation.GarbageCollector;
public class JvmConfigFluentImpl> extends ConfigurationFluentImpl implements JvmConfigFluent{
private int xms = 0;
private int xmx = 0;
private boolean server = false;
private boolean useStringDeduplication = false;
private boolean preferIPv4Stack = false;
private boolean heapDumpOnOutOfMemoryError = false;
private boolean useGCOverheadLimit = false;
private GarbageCollector gc = io.ap4k.option.annotation.GarbageCollector.Undefined;
private SecureRandomSource secureRandom = io.ap4k.option.annotation.SecureRandomSource.Undefined;
public JvmConfigFluentImpl(){
}
public JvmConfigFluentImpl(JvmConfig instance){
this.withProject(instance.getProject());
this.withAttributes(instance.getAttributes());
this.withXms(instance.getXms());
this.withXmx(instance.getXmx());
this.withServer(instance.isServer());
this.withUseStringDeduplication(instance.isUseStringDeduplication());
this.withPreferIPv4Stack(instance.isPreferIPv4Stack());
this.withHeapDumpOnOutOfMemoryError(instance.isHeapDumpOnOutOfMemoryError());
this.withUseGCOverheadLimit(instance.isUseGCOverheadLimit());
this.withGc(instance.getGc());
this.withSecureRandom(instance.getSecureRandom());
}
public int getXms(){
return this.xms;
}
public A withXms(int xms){
this.xms=xms; return (A) this;
}
public Boolean hasXms(){
return true;
}
public int getXmx(){
return this.xmx;
}
public A withXmx(int xmx){
this.xmx=xmx; return (A) this;
}
public Boolean hasXmx(){
return true;
}
public boolean isServer(){
return this.server;
}
public A withServer(boolean server){
this.server=server; return (A) this;
}
public Boolean hasServer(){
return true;
}
public boolean isUseStringDeduplication(){
return this.useStringDeduplication;
}
public A withUseStringDeduplication(boolean useStringDeduplication){
this.useStringDeduplication=useStringDeduplication; return (A) this;
}
public Boolean hasUseStringDeduplication(){
return true;
}
public boolean isPreferIPv4Stack(){
return this.preferIPv4Stack;
}
public A withPreferIPv4Stack(boolean preferIPv4Stack){
this.preferIPv4Stack=preferIPv4Stack; return (A) this;
}
public Boolean hasPreferIPv4Stack(){
return true;
}
public boolean isHeapDumpOnOutOfMemoryError(){
return this.heapDumpOnOutOfMemoryError;
}
public A withHeapDumpOnOutOfMemoryError(boolean heapDumpOnOutOfMemoryError){
this.heapDumpOnOutOfMemoryError=heapDumpOnOutOfMemoryError; return (A) this;
}
public Boolean hasHeapDumpOnOutOfMemoryError(){
return true;
}
public boolean isUseGCOverheadLimit(){
return this.useGCOverheadLimit;
}
public A withUseGCOverheadLimit(boolean useGCOverheadLimit){
this.useGCOverheadLimit=useGCOverheadLimit; return (A) this;
}
public Boolean hasUseGCOverheadLimit(){
return true;
}
public GarbageCollector getGc(){
return this.gc;
}
public A withGc(GarbageCollector gc){
this.gc=gc; return (A) this;
}
public Boolean hasGc(){
return this.gc != null;
}
public SecureRandomSource getSecureRandom(){
return this.secureRandom;
}
public A withSecureRandom(SecureRandomSource secureRandom){
this.secureRandom=secureRandom; return (A) this;
}
public Boolean hasSecureRandom(){
return this.secureRandom != null;
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
JvmConfigFluentImpl that = (JvmConfigFluentImpl) o;
if (xms != that.xms) return false;
if (xmx != that.xmx) return false;
if (server != that.server) return false;
if (useStringDeduplication != that.useStringDeduplication) return false;
if (preferIPv4Stack != that.preferIPv4Stack) return false;
if (heapDumpOnOutOfMemoryError != that.heapDumpOnOutOfMemoryError) return false;
if (useGCOverheadLimit != that.useGCOverheadLimit) return false;
if (gc != null ? !gc.equals(that.gc) :that.gc != null) return false;
if (secureRandom != null ? !secureRandom.equals(that.secureRandom) :that.secureRandom != null) return false;
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy