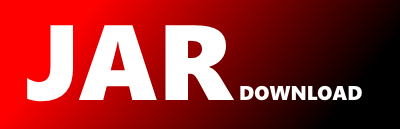
io.apicurio.datamodels.models.asyncapi.v26.io.AsyncApi26ModelReader Maven / Gradle / Ivy
package io.apicurio.datamodels.models.asyncapi.v26.io;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.node.ObjectNode;
import io.apicurio.datamodels.models.RootNode;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26Binding;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26ChannelBindings;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26ChannelItem;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26Channels;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26Components;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26Contact;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26CorrelationID;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26Document;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26DocumentImpl;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26ExternalDocumentation;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26Info;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26License;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26Message;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26MessageBindings;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26MessageExample;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26MessageTrait;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26OAuthFlow;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26OAuthFlows;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26OneOfMessages;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26Operation;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26OperationBindings;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26OperationTrait;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26Parameter;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26Parameters;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26Schema;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26SecurityRequirement;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26SecurityScheme;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26Server;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26ServerBindings;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26ServerVariable;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26Servers;
import io.apicurio.datamodels.models.asyncapi.v26.AsyncApi26Tag;
import io.apicurio.datamodels.models.io.ModelReader;
import io.apicurio.datamodels.models.union.BooleanUnionValue;
import io.apicurio.datamodels.models.union.BooleanUnionValueImpl;
import io.apicurio.datamodels.models.union.SchemaListUnionValue;
import io.apicurio.datamodels.models.union.SchemaListUnionValueImpl;
import io.apicurio.datamodels.models.util.JsonUtil;
import io.apicurio.datamodels.models.util.ReaderUtil;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
public class AsyncApi26ModelReader implements ModelReader {
public void readDocument(ObjectNode json, AsyncApi26Document node) {
{
String value = JsonUtil.consumeStringProperty(json, "asyncapi");
node.setAsyncapi(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "id");
node.setId(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "info");
if (object != null) {
node.setInfo(node.createInfo());
readInfo(object, (AsyncApi26Info) node.getInfo());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "servers");
if (object != null) {
node.setServers(node.createServers());
readServers(object, (AsyncApi26Servers) node.getServers());
}
}
{
String value = JsonUtil.consumeStringProperty(json, "defaultContentType");
node.setDefaultContentType(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "channels");
if (object != null) {
node.setChannels(node.createChannels());
readChannels(object, (AsyncApi26Channels) node.getChannels());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "components");
if (object != null) {
node.setComponents(node.createComponents());
readComponents(object, (AsyncApi26Components) node.getComponents());
}
}
{
List objects = JsonUtil.consumeObjectArrayProperty(json, "tags");
if (objects != null) {
objects.forEach(object -> {
AsyncApi26Tag model = (AsyncApi26Tag) node.createTag();
this.readTag(object, model);
node.addTag(model);
});
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "externalDocs");
if (object != null) {
node.setExternalDocs(node.createExternalDocumentation());
readExternalDocumentation(object, (AsyncApi26ExternalDocumentation) node.getExternalDocs());
}
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
@Override
public RootNode readRoot(ObjectNode json) {
AsyncApi26Document rootModel = new AsyncApi26DocumentImpl();
this.readDocument(json, rootModel);
return rootModel;
}
public void readInfo(ObjectNode json, AsyncApi26Info node) {
{
String value = JsonUtil.consumeStringProperty(json, "title");
node.setTitle(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "version");
node.setVersion(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "description");
node.setDescription(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "termsOfService");
node.setTermsOfService(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "contact");
if (object != null) {
node.setContact(node.createContact());
readContact(object, (AsyncApi26Contact) node.getContact());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "license");
if (object != null) {
node.setLicense(node.createLicense());
readLicense(object, (AsyncApi26License) node.getLicense());
}
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readContact(ObjectNode json, AsyncApi26Contact node) {
{
String value = JsonUtil.consumeStringProperty(json, "name");
node.setName(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "url");
node.setUrl(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "email");
node.setEmail(value);
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readLicense(ObjectNode json, AsyncApi26License node) {
{
String value = JsonUtil.consumeStringProperty(json, "name");
node.setName(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "url");
node.setUrl(value);
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readServers(ObjectNode json, AsyncApi26Servers node) {
{
List propertyNames = JsonUtil.keys(json);
propertyNames.forEach(name -> {
ObjectNode object = JsonUtil.consumeObjectProperty(json, name);
if (object != null) {
AsyncApi26Server model = (AsyncApi26Server) node.createServer();
this.readServer(object, model);
node.addItem(name, model);
}
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readServer(ObjectNode json, AsyncApi26Server node) {
{
String value = JsonUtil.consumeStringProperty(json, "$ref");
node.set$ref(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "url");
node.setUrl(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "protocol");
node.setProtocol(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "protocolVersion");
node.setProtocolVersion(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "description");
node.setDescription(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "variables");
JsonUtil.keys(object).forEach(name -> {
ObjectNode mapValue = JsonUtil.consumeObjectProperty(object, name);
if (mapValue != null) {
AsyncApi26ServerVariable model = (AsyncApi26ServerVariable) node.createServerVariable();
this.readServerVariable(mapValue, model);
node.addVariable(name, model);
}
});
}
{
List objects = JsonUtil.consumeObjectArrayProperty(json, "security");
if (objects != null) {
objects.forEach(object -> {
AsyncApi26SecurityRequirement model = (AsyncApi26SecurityRequirement) node
.createSecurityRequirement();
this.readSecurityRequirement(object, model);
node.addSecurity(model);
});
}
}
{
List objects = JsonUtil.consumeObjectArrayProperty(json, "tags");
if (objects != null) {
objects.forEach(object -> {
AsyncApi26Tag model = (AsyncApi26Tag) node.createTag();
this.readTag(object, model);
node.addTag(model);
});
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "bindings");
if (object != null) {
node.setBindings(node.createServerBindings());
readServerBindings(object, (AsyncApi26ServerBindings) node.getBindings());
}
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readServerVariable(ObjectNode json, AsyncApi26ServerVariable node) {
{
String value = JsonUtil.consumeStringProperty(json, "$ref");
node.set$ref(value);
}
{
List value = JsonUtil.consumeStringArrayProperty(json, "enum");
node.setEnum(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "default");
node.setDefault(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "description");
node.setDescription(value);
}
{
List value = JsonUtil.consumeStringArrayProperty(json, "examples");
node.setExamples(value);
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readChannels(ObjectNode json, AsyncApi26Channels node) {
{
List propertyNames = JsonUtil.keys(json);
propertyNames.forEach(name -> {
ObjectNode object = JsonUtil.consumeObjectProperty(json, name);
if (object != null) {
AsyncApi26ChannelItem model = (AsyncApi26ChannelItem) node.createChannelItem();
this.readChannelItem(object, model);
node.addItem(name, model);
}
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readChannelItem(ObjectNode json, AsyncApi26ChannelItem node) {
{
String value = JsonUtil.consumeStringProperty(json, "$ref");
node.set$ref(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "description");
node.setDescription(value);
}
{
List value = JsonUtil.consumeStringArrayProperty(json, "servers");
node.setServers(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "subscribe");
if (object != null) {
node.setSubscribe(node.createOperation());
readOperation(object, (AsyncApi26Operation) node.getSubscribe());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "publish");
if (object != null) {
node.setPublish(node.createOperation());
readOperation(object, (AsyncApi26Operation) node.getPublish());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "parameters");
if (object != null) {
node.setParameters(node.createParameters());
readParameters(object, (AsyncApi26Parameters) node.getParameters());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "bindings");
if (object != null) {
node.setBindings(node.createChannelBindings());
readChannelBindings(object, (AsyncApi26ChannelBindings) node.getBindings());
}
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readOperation(ObjectNode json, AsyncApi26Operation node) {
{
String value = JsonUtil.consumeStringProperty(json, "operationId");
node.setOperationId(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "summary");
node.setSummary(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "description");
node.setDescription(value);
}
{
List objects = JsonUtil.consumeObjectArrayProperty(json, "security");
if (objects != null) {
objects.forEach(object -> {
AsyncApi26SecurityRequirement model = (AsyncApi26SecurityRequirement) node
.createSecurityRequirement();
this.readSecurityRequirement(object, model);
node.addSecurity(model);
});
}
}
{
List objects = JsonUtil.consumeObjectArrayProperty(json, "tags");
if (objects != null) {
objects.forEach(object -> {
AsyncApi26Tag model = (AsyncApi26Tag) node.createTag();
this.readTag(object, model);
node.addTag(model);
});
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "externalDocs");
if (object != null) {
node.setExternalDocs(node.createExternalDocumentation());
readExternalDocumentation(object, (AsyncApi26ExternalDocumentation) node.getExternalDocs());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "bindings");
if (object != null) {
node.setBindings(node.createOperationBindings());
readOperationBindings(object, (AsyncApi26OperationBindings) node.getBindings());
}
}
{
List objects = JsonUtil.consumeObjectArrayProperty(json, "traits");
if (objects != null) {
objects.forEach(object -> {
AsyncApi26OperationTrait model = (AsyncApi26OperationTrait) node.createOperationTrait();
this.readOperationTrait(object, model);
node.addTrait(model);
});
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "message");
if (object != null) {
node.setMessage(node.createMessage());
readMessage(object, (AsyncApi26Message) node.getMessage());
}
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readOperationTrait(ObjectNode json, AsyncApi26OperationTrait node) {
{
String value = JsonUtil.consumeStringProperty(json, "$ref");
node.set$ref(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "operationId");
node.setOperationId(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "summary");
node.setSummary(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "description");
node.setDescription(value);
}
{
List objects = JsonUtil.consumeObjectArrayProperty(json, "security");
if (objects != null) {
objects.forEach(object -> {
AsyncApi26SecurityRequirement model = (AsyncApi26SecurityRequirement) node
.createSecurityRequirement();
this.readSecurityRequirement(object, model);
node.addSecurity(model);
});
}
}
{
List objects = JsonUtil.consumeObjectArrayProperty(json, "tags");
if (objects != null) {
objects.forEach(object -> {
AsyncApi26Tag model = (AsyncApi26Tag) node.createTag();
this.readTag(object, model);
node.addTag(model);
});
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "externalDocs");
if (object != null) {
node.setExternalDocs(node.createExternalDocumentation());
readExternalDocumentation(object, (AsyncApi26ExternalDocumentation) node.getExternalDocs());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "bindings");
if (object != null) {
node.setBindings(node.createOperationBindings());
readOperationBindings(object, (AsyncApi26OperationBindings) node.getBindings());
}
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readParameters(ObjectNode json, AsyncApi26Parameters node) {
{
List propertyNames = JsonUtil.keys(json);
propertyNames.forEach(name -> {
ObjectNode object = JsonUtil.consumeObjectProperty(json, name);
if (object != null) {
AsyncApi26Parameter model = (AsyncApi26Parameter) node.createParameter();
this.readParameter(object, model);
node.addItem(name, model);
}
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readParameter(ObjectNode json, AsyncApi26Parameter node) {
{
String value = JsonUtil.consumeStringProperty(json, "$ref");
node.set$ref(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "description");
node.setDescription(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "schema");
if (object != null) {
node.setSchema(node.createSchema());
readSchema(object, (AsyncApi26Schema) node.getSchema());
}
}
{
String value = JsonUtil.consumeStringProperty(json, "location");
node.setLocation(value);
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readServerBindings(ObjectNode json, AsyncApi26ServerBindings node) {
{
String value = JsonUtil.consumeStringProperty(json, "$ref");
node.set$ref(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "http");
if (object != null) {
node.setHttp(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getHttp());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "ws");
if (object != null) {
node.setWs(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getWs());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "kafka");
if (object != null) {
node.setKafka(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getKafka());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "anypointmq");
if (object != null) {
node.setAnypointmq(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getAnypointmq());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "amqp");
if (object != null) {
node.setAmqp(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getAmqp());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "amqp1");
if (object != null) {
node.setAmqp1(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getAmqp1());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "mqtt");
if (object != null) {
node.setMqtt(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getMqtt());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "mqtt5");
if (object != null) {
node.setMqtt5(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getMqtt5());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "nats");
if (object != null) {
node.setNats(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getNats());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "jms");
if (object != null) {
node.setJms(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getJms());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "sns");
if (object != null) {
node.setSns(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getSns());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "solace");
if (object != null) {
node.setSolace(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getSolace());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "sqs");
if (object != null) {
node.setSqs(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getSqs());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "stomp");
if (object != null) {
node.setStomp(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getStomp());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "redis");
if (object != null) {
node.setRedis(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getRedis());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "mercure");
if (object != null) {
node.setMercure(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getMercure());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "ibmmq");
if (object != null) {
node.setIbmmq(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getIbmmq());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "googlepubsub");
if (object != null) {
node.setGooglepubsub(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getGooglepubsub());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "pulsar");
if (object != null) {
node.setPulsar(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getPulsar());
}
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readChannelBindings(ObjectNode json, AsyncApi26ChannelBindings node) {
{
String value = JsonUtil.consumeStringProperty(json, "$ref");
node.set$ref(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "http");
if (object != null) {
node.setHttp(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getHttp());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "ws");
if (object != null) {
node.setWs(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getWs());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "kafka");
if (object != null) {
node.setKafka(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getKafka());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "anypointmq");
if (object != null) {
node.setAnypointmq(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getAnypointmq());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "amqp");
if (object != null) {
node.setAmqp(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getAmqp());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "amqp1");
if (object != null) {
node.setAmqp1(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getAmqp1());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "mqtt");
if (object != null) {
node.setMqtt(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getMqtt());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "mqtt5");
if (object != null) {
node.setMqtt5(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getMqtt5());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "nats");
if (object != null) {
node.setNats(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getNats());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "jms");
if (object != null) {
node.setJms(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getJms());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "sns");
if (object != null) {
node.setSns(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getSns());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "solace");
if (object != null) {
node.setSolace(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getSolace());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "sqs");
if (object != null) {
node.setSqs(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getSqs());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "stomp");
if (object != null) {
node.setStomp(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getStomp());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "redis");
if (object != null) {
node.setRedis(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getRedis());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "mercure");
if (object != null) {
node.setMercure(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getMercure());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "ibmmq");
if (object != null) {
node.setIbmmq(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getIbmmq());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "googlepubsub");
if (object != null) {
node.setGooglepubsub(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getGooglepubsub());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "pulsar");
if (object != null) {
node.setPulsar(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getPulsar());
}
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readOperationBindings(ObjectNode json, AsyncApi26OperationBindings node) {
{
String value = JsonUtil.consumeStringProperty(json, "$ref");
node.set$ref(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "http");
if (object != null) {
node.setHttp(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getHttp());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "ws");
if (object != null) {
node.setWs(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getWs());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "kafka");
if (object != null) {
node.setKafka(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getKafka());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "anypointmq");
if (object != null) {
node.setAnypointmq(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getAnypointmq());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "amqp");
if (object != null) {
node.setAmqp(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getAmqp());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "amqp1");
if (object != null) {
node.setAmqp1(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getAmqp1());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "mqtt");
if (object != null) {
node.setMqtt(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getMqtt());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "mqtt5");
if (object != null) {
node.setMqtt5(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getMqtt5());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "nats");
if (object != null) {
node.setNats(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getNats());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "jms");
if (object != null) {
node.setJms(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getJms());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "sns");
if (object != null) {
node.setSns(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getSns());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "solace");
if (object != null) {
node.setSolace(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getSolace());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "sqs");
if (object != null) {
node.setSqs(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getSqs());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "stomp");
if (object != null) {
node.setStomp(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getStomp());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "redis");
if (object != null) {
node.setRedis(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getRedis());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "mercure");
if (object != null) {
node.setMercure(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getMercure());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "ibmmq");
if (object != null) {
node.setIbmmq(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getIbmmq());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "googlepubsub");
if (object != null) {
node.setGooglepubsub(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getGooglepubsub());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "pulsar");
if (object != null) {
node.setPulsar(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getPulsar());
}
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readMessageBindings(ObjectNode json, AsyncApi26MessageBindings node) {
{
String value = JsonUtil.consumeStringProperty(json, "$ref");
node.set$ref(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "http");
if (object != null) {
node.setHttp(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getHttp());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "ws");
if (object != null) {
node.setWs(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getWs());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "kafka");
if (object != null) {
node.setKafka(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getKafka());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "anypointmq");
if (object != null) {
node.setAnypointmq(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getAnypointmq());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "amqp");
if (object != null) {
node.setAmqp(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getAmqp());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "amqp1");
if (object != null) {
node.setAmqp1(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getAmqp1());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "mqtt");
if (object != null) {
node.setMqtt(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getMqtt());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "mqtt5");
if (object != null) {
node.setMqtt5(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getMqtt5());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "nats");
if (object != null) {
node.setNats(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getNats());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "jms");
if (object != null) {
node.setJms(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getJms());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "sns");
if (object != null) {
node.setSns(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getSns());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "solace");
if (object != null) {
node.setSolace(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getSolace());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "sqs");
if (object != null) {
node.setSqs(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getSqs());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "stomp");
if (object != null) {
node.setStomp(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getStomp());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "redis");
if (object != null) {
node.setRedis(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getRedis());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "mercure");
if (object != null) {
node.setMercure(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getMercure());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "ibmmq");
if (object != null) {
node.setIbmmq(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getIbmmq());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "googlepubsub");
if (object != null) {
node.setGooglepubsub(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getGooglepubsub());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "pulsar");
if (object != null) {
node.setPulsar(node.createBinding());
readBinding(object, (AsyncApi26Binding) node.getPulsar());
}
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readOneOfMessages(ObjectNode json, AsyncApi26OneOfMessages node) {
{
List objects = JsonUtil.consumeObjectArrayProperty(json, "oneOf");
if (objects != null) {
objects.forEach(object -> {
AsyncApi26Message model = (AsyncApi26Message) node.createMessage();
this.readMessage(object, model);
node.addOneOf(model);
});
}
}
ReaderUtil.readExtraProperties(json, node);
}
public void readMessage(ObjectNode json, AsyncApi26Message node) {
{
String value = JsonUtil.consumeStringProperty(json, "$ref");
node.set$ref(value);
}
{
List objects = JsonUtil.consumeObjectArrayProperty(json, "oneOf");
if (objects != null) {
objects.forEach(object -> {
AsyncApi26Message model = (AsyncApi26Message) node.createMessage();
this.readMessage(object, model);
node.addOneOf(model);
});
}
}
{
String value = JsonUtil.consumeStringProperty(json, "messageId");
node.setMessageId(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "headers");
if (object != null) {
node.setHeaders(node.createSchema());
readSchema(object, (AsyncApi26Schema) node.getHeaders());
}
}
{
JsonNode value = JsonUtil.consumeAnyProperty(json, "payload");
node.setPayload(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "correlationId");
if (object != null) {
node.setCorrelationId(node.createCorrelationID());
readCorrelationID(object, (AsyncApi26CorrelationID) node.getCorrelationId());
}
}
{
String value = JsonUtil.consumeStringProperty(json, "schemaFormat");
node.setSchemaFormat(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "contentType");
node.setContentType(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "name");
node.setName(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "title");
node.setTitle(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "summary");
node.setSummary(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "description");
node.setDescription(value);
}
{
List objects = JsonUtil.consumeObjectArrayProperty(json, "tags");
if (objects != null) {
objects.forEach(object -> {
AsyncApi26Tag model = (AsyncApi26Tag) node.createTag();
this.readTag(object, model);
node.addTag(model);
});
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "externalDocs");
if (object != null) {
node.setExternalDocs(node.createExternalDocumentation());
readExternalDocumentation(object, (AsyncApi26ExternalDocumentation) node.getExternalDocs());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "bindings");
if (object != null) {
node.setBindings(node.createMessageBindings());
readMessageBindings(object, (AsyncApi26MessageBindings) node.getBindings());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "examples");
if (object != null) {
node.setExamples(node.createMessageExample());
readMessageExample(object, (AsyncApi26MessageExample) node.getExamples());
}
}
{
List objects = JsonUtil.consumeObjectArrayProperty(json, "traits");
if (objects != null) {
objects.forEach(object -> {
AsyncApi26MessageTrait model = (AsyncApi26MessageTrait) node.createMessageTrait();
this.readMessageTrait(object, model);
node.addTrait(model);
});
}
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readMessageTrait(ObjectNode json, AsyncApi26MessageTrait node) {
{
String value = JsonUtil.consumeStringProperty(json, "$ref");
node.set$ref(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "messageId");
node.setMessageId(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "headers");
if (object != null) {
node.setHeaders(node.createSchema());
readSchema(object, (AsyncApi26Schema) node.getHeaders());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "correlationId");
if (object != null) {
node.setCorrelationId(node.createCorrelationID());
readCorrelationID(object, (AsyncApi26CorrelationID) node.getCorrelationId());
}
}
{
String value = JsonUtil.consumeStringProperty(json, "schemaFormat");
node.setSchemaFormat(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "contentType");
node.setContentType(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "name");
node.setName(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "title");
node.setTitle(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "summary");
node.setSummary(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "description");
node.setDescription(value);
}
{
List objects = JsonUtil.consumeObjectArrayProperty(json, "tags");
if (objects != null) {
objects.forEach(object -> {
AsyncApi26Tag model = (AsyncApi26Tag) node.createTag();
this.readTag(object, model);
node.addTag(model);
});
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "externalDocs");
if (object != null) {
node.setExternalDocs(node.createExternalDocumentation());
readExternalDocumentation(object, (AsyncApi26ExternalDocumentation) node.getExternalDocs());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "bindings");
if (object != null) {
node.setBindings(node.createMessageBindings());
readMessageBindings(object, (AsyncApi26MessageBindings) node.getBindings());
}
}
{
Map value = JsonUtil.consumeAnyMapProperty(json, "examples");
node.setExamples(value);
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readMessageExample(ObjectNode json, AsyncApi26MessageExample node) {
{
Map value = JsonUtil.consumeAnyMapProperty(json, "headers");
node.setHeaders(value);
}
{
JsonNode value = JsonUtil.consumeAnyProperty(json, "payload");
node.setPayload(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "name");
node.setName(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "summary");
node.setSummary(value);
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readTag(ObjectNode json, AsyncApi26Tag node) {
{
String value = JsonUtil.consumeStringProperty(json, "name");
node.setName(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "description");
node.setDescription(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "externalDocs");
if (object != null) {
node.setExternalDocs(node.createExternalDocumentation());
readExternalDocumentation(object, (AsyncApi26ExternalDocumentation) node.getExternalDocs());
}
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readExternalDocumentation(ObjectNode json, AsyncApi26ExternalDocumentation node) {
{
String value = JsonUtil.consumeStringProperty(json, "description");
node.setDescription(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "url");
node.setUrl(value);
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readComponents(ObjectNode json, AsyncApi26Components node) {
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "schemas");
JsonUtil.keys(object).forEach(name -> {
ObjectNode mapValue = JsonUtil.consumeObjectProperty(object, name);
if (mapValue != null) {
AsyncApi26Schema model = (AsyncApi26Schema) node.createSchema();
this.readSchema(mapValue, model);
node.addSchema(name, model);
}
});
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "servers");
JsonUtil.keys(object).forEach(name -> {
ObjectNode mapValue = JsonUtil.consumeObjectProperty(object, name);
if (mapValue != null) {
AsyncApi26Server model = (AsyncApi26Server) node.createServer();
this.readServer(mapValue, model);
node.addServer(name, model);
}
});
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "serverVariables");
JsonUtil.keys(object).forEach(name -> {
ObjectNode mapValue = JsonUtil.consumeObjectProperty(object, name);
if (mapValue != null) {
AsyncApi26ServerVariable model = (AsyncApi26ServerVariable) node.createServerVariable();
this.readServerVariable(mapValue, model);
node.addServerVariable(name, model);
}
});
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "channels");
JsonUtil.keys(object).forEach(name -> {
ObjectNode mapValue = JsonUtil.consumeObjectProperty(object, name);
if (mapValue != null) {
AsyncApi26ChannelItem model = (AsyncApi26ChannelItem) node.createChannelItem();
this.readChannelItem(mapValue, model);
node.addChannel(name, model);
}
});
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "messages");
JsonUtil.keys(object).forEach(name -> {
ObjectNode mapValue = JsonUtil.consumeObjectProperty(object, name);
if (mapValue != null) {
AsyncApi26Message model = (AsyncApi26Message) node.createMessage();
this.readMessage(mapValue, model);
node.addMessage(name, model);
}
});
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "securitySchemes");
JsonUtil.keys(object).forEach(name -> {
ObjectNode mapValue = JsonUtil.consumeObjectProperty(object, name);
if (mapValue != null) {
AsyncApi26SecurityScheme model = (AsyncApi26SecurityScheme) node.createSecurityScheme();
this.readSecurityScheme(mapValue, model);
node.addSecurityScheme(name, model);
}
});
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "parameters");
JsonUtil.keys(object).forEach(name -> {
ObjectNode mapValue = JsonUtil.consumeObjectProperty(object, name);
if (mapValue != null) {
AsyncApi26Parameter model = (AsyncApi26Parameter) node.createParameter();
this.readParameter(mapValue, model);
node.addParameter(name, model);
}
});
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "correlationIds");
JsonUtil.keys(object).forEach(name -> {
ObjectNode mapValue = JsonUtil.consumeObjectProperty(object, name);
if (mapValue != null) {
AsyncApi26CorrelationID model = (AsyncApi26CorrelationID) node.createCorrelationID();
this.readCorrelationID(mapValue, model);
node.addCorrelationId(name, model);
}
});
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "operationTraits");
JsonUtil.keys(object).forEach(name -> {
ObjectNode mapValue = JsonUtil.consumeObjectProperty(object, name);
if (mapValue != null) {
AsyncApi26OperationTrait model = (AsyncApi26OperationTrait) node.createOperationTrait();
this.readOperationTrait(mapValue, model);
node.addOperationTrait(name, model);
}
});
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "messageTraits");
JsonUtil.keys(object).forEach(name -> {
ObjectNode mapValue = JsonUtil.consumeObjectProperty(object, name);
if (mapValue != null) {
AsyncApi26MessageTrait model = (AsyncApi26MessageTrait) node.createMessageTrait();
this.readMessageTrait(mapValue, model);
node.addMessageTrait(name, model);
}
});
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "serverBindings");
JsonUtil.keys(object).forEach(name -> {
ObjectNode mapValue = JsonUtil.consumeObjectProperty(object, name);
if (mapValue != null) {
AsyncApi26ServerBindings model = (AsyncApi26ServerBindings) node.createServerBindings();
this.readServerBindings(mapValue, model);
node.addServerBinding(name, model);
}
});
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "channelBindings");
JsonUtil.keys(object).forEach(name -> {
ObjectNode mapValue = JsonUtil.consumeObjectProperty(object, name);
if (mapValue != null) {
AsyncApi26ChannelBindings model = (AsyncApi26ChannelBindings) node.createChannelBindings();
this.readChannelBindings(mapValue, model);
node.addChannelBinding(name, model);
}
});
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "operationBindings");
JsonUtil.keys(object).forEach(name -> {
ObjectNode mapValue = JsonUtil.consumeObjectProperty(object, name);
if (mapValue != null) {
AsyncApi26OperationBindings model = (AsyncApi26OperationBindings) node.createOperationBindings();
this.readOperationBindings(mapValue, model);
node.addOperationBinding(name, model);
}
});
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "messageBindings");
JsonUtil.keys(object).forEach(name -> {
ObjectNode mapValue = JsonUtil.consumeObjectProperty(object, name);
if (mapValue != null) {
AsyncApi26MessageBindings model = (AsyncApi26MessageBindings) node.createMessageBindings();
this.readMessageBindings(mapValue, model);
node.addMessageBinding(name, model);
}
});
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readSchema(ObjectNode json, AsyncApi26Schema node) {
{
String value = JsonUtil.consumeStringProperty(json, "$ref");
node.set$ref(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "title");
node.setTitle(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "type");
node.setType(value);
}
{
List value = JsonUtil.consumeStringArrayProperty(json, "required");
node.setRequired(value);
}
{
Number value = JsonUtil.consumeNumberProperty(json, "multipleOf");
node.setMultipleOf(value);
}
{
Number value = JsonUtil.consumeNumberProperty(json, "maximum");
node.setMaximum(value);
}
{
Number value = JsonUtil.consumeNumberProperty(json, "exclusiveMaximum");
node.setExclusiveMaximum(value);
}
{
Number value = JsonUtil.consumeNumberProperty(json, "minimum");
node.setMinimum(value);
}
{
Number value = JsonUtil.consumeNumberProperty(json, "exclusiveMinimum");
node.setExclusiveMinimum(value);
}
{
Integer value = JsonUtil.consumeIntegerProperty(json, "maxLength");
node.setMaxLength(value);
}
{
Integer value = JsonUtil.consumeIntegerProperty(json, "minLength");
node.setMinLength(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "pattern");
node.setPattern(value);
}
{
Integer value = JsonUtil.consumeIntegerProperty(json, "maxItems");
node.setMaxItems(value);
}
{
Integer value = JsonUtil.consumeIntegerProperty(json, "minItems");
node.setMinItems(value);
}
{
Boolean value = JsonUtil.consumeBooleanProperty(json, "uniqueItems");
node.setUniqueItems(value);
}
{
Integer value = JsonUtil.consumeIntegerProperty(json, "maxProperties");
node.setMaxProperties(value);
}
{
Integer value = JsonUtil.consumeIntegerProperty(json, "minProperties");
node.setMinProperties(value);
}
{
List value = JsonUtil.consumeAnyArrayProperty(json, "enum");
node.setEnum(value);
}
{
JsonNode value = JsonUtil.consumeAnyProperty(json, "const");
node.setConst(value);
}
{
List value = JsonUtil.consumeAnyArrayProperty(json, "examples");
node.setExamples(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "if");
if (object != null) {
node.setIf(node.createSchema());
readSchema(object, (AsyncApi26Schema) node.getIf());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "then");
if (object != null) {
node.setThen(node.createSchema());
readSchema(object, (AsyncApi26Schema) node.getThen());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "else");
if (object != null) {
node.setElse(node.createSchema());
readSchema(object, (AsyncApi26Schema) node.getElse());
}
}
{
Boolean value = JsonUtil.consumeBooleanProperty(json, "readOnly");
node.setReadOnly(value);
}
{
Boolean value = JsonUtil.consumeBooleanProperty(json, "writeOnly");
node.setWriteOnly(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "properties");
JsonUtil.keys(object).forEach(name -> {
ObjectNode mapValue = JsonUtil.consumeObjectProperty(object, name);
if (mapValue != null) {
AsyncApi26Schema model = (AsyncApi26Schema) node.createSchema();
this.readSchema(mapValue, model);
node.addProperty(name, model);
}
});
}
{
Map value = JsonUtil.consumeStringMapProperty(json, "patternProperties");
node.setPatternProperties(value);
}
{
JsonNode value = JsonUtil.consumeAnyProperty(json, "additionalProperties");
if (value != null) {
if (JsonUtil.isObject(value)) {
ObjectNode object = JsonUtil.toObject(value);
node.setAdditionalProperties(node.createSchema());
readSchema(object, (AsyncApi26Schema) node.getAdditionalProperties());
} else if (JsonUtil.isBoolean(value)) {
Boolean pValue = JsonUtil.toBoolean(value);
BooleanUnionValue unionValue = new BooleanUnionValueImpl(pValue);
node.setAdditionalProperties(unionValue);
} else {
node.addExtraProperty("additionalProperties", value);
}
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "additionalItems");
if (object != null) {
node.setAdditionalItems(node.createSchema());
readSchema(object, (AsyncApi26Schema) node.getAdditionalItems());
}
}
{
JsonNode value = JsonUtil.consumeAnyProperty(json, "items");
if (value != null) {
if (JsonUtil.isObject(value)) {
ObjectNode object = JsonUtil.toObject(value);
node.setItems(node.createSchema());
readSchema(object, (AsyncApi26Schema) node.getItems());
} else if (JsonUtil.isArray(value)) {
List array = JsonUtil.toList(value);
List models = new ArrayList<>();
array.forEach(item -> {
ObjectNode object = JsonUtil.toObject(item);
AsyncApi26Schema model = (AsyncApi26Schema) node.createSchema();
this.readSchema(object, model);
models.add(model);
});
@SuppressWarnings({"unchecked", "rawtypes"})
SchemaListUnionValue unionValue = new SchemaListUnionValueImpl((List) models);
node.setItems(unionValue);
} else {
node.addExtraProperty("items", value);
}
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "propertyNames");
if (object != null) {
node.setPropertyNames(node.createSchema());
readSchema(object, (AsyncApi26Schema) node.getPropertyNames());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "contains");
if (object != null) {
node.setContains(node.createSchema());
readSchema(object, (AsyncApi26Schema) node.getContains());
}
}
{
List objects = JsonUtil.consumeObjectArrayProperty(json, "allOf");
if (objects != null) {
objects.forEach(object -> {
AsyncApi26Schema model = (AsyncApi26Schema) node.createSchema();
this.readSchema(object, model);
node.addAllOf(model);
});
}
}
{
List objects = JsonUtil.consumeObjectArrayProperty(json, "oneOf");
if (objects != null) {
objects.forEach(object -> {
AsyncApi26Schema model = (AsyncApi26Schema) node.createSchema();
this.readSchema(object, model);
node.addOneOf(model);
});
}
}
{
List objects = JsonUtil.consumeObjectArrayProperty(json, "anyOf");
if (objects != null) {
objects.forEach(object -> {
AsyncApi26Schema model = (AsyncApi26Schema) node.createSchema();
this.readSchema(object, model);
node.addAnyOf(model);
});
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "not");
if (object != null) {
node.setNot(node.createSchema());
readSchema(object, (AsyncApi26Schema) node.getNot());
}
}
{
String value = JsonUtil.consumeStringProperty(json, "description");
node.setDescription(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "format");
node.setFormat(value);
}
{
JsonNode value = JsonUtil.consumeAnyProperty(json, "default");
node.setDefault(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "discriminator");
node.setDiscriminator(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "externalDocs");
if (object != null) {
node.setExternalDocs(node.createExternalDocumentation());
readExternalDocumentation(object, (AsyncApi26ExternalDocumentation) node.getExternalDocs());
}
}
{
Boolean value = JsonUtil.consumeBooleanProperty(json, "deprecated");
node.setDeprecated(value);
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readSecurityScheme(ObjectNode json, AsyncApi26SecurityScheme node) {
{
String value = JsonUtil.consumeStringProperty(json, "$ref");
node.set$ref(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "type");
node.setType(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "description");
node.setDescription(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "name");
node.setName(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "in");
node.setIn(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "scheme");
node.setScheme(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "bearerFormat");
node.setBearerFormat(value);
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "flows");
if (object != null) {
node.setFlows(node.createOAuthFlows());
readOAuthFlows(object, (AsyncApi26OAuthFlows) node.getFlows());
}
}
{
String value = JsonUtil.consumeStringProperty(json, "openIdConnectUrl");
node.setOpenIdConnectUrl(value);
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readOAuthFlows(ObjectNode json, AsyncApi26OAuthFlows node) {
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "implicit");
if (object != null) {
node.setImplicit(node.createOAuthFlow());
readOAuthFlow(object, (AsyncApi26OAuthFlow) node.getImplicit());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "password");
if (object != null) {
node.setPassword(node.createOAuthFlow());
readOAuthFlow(object, (AsyncApi26OAuthFlow) node.getPassword());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "clientCredentials");
if (object != null) {
node.setClientCredentials(node.createOAuthFlow());
readOAuthFlow(object, (AsyncApi26OAuthFlow) node.getClientCredentials());
}
}
{
ObjectNode object = JsonUtil.consumeObjectProperty(json, "authorizationCode");
if (object != null) {
node.setAuthorizationCode(node.createOAuthFlow());
readOAuthFlow(object, (AsyncApi26OAuthFlow) node.getAuthorizationCode());
}
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readOAuthFlow(ObjectNode json, AsyncApi26OAuthFlow node) {
{
String value = JsonUtil.consumeStringProperty(json, "authorizationUrl");
node.setAuthorizationUrl(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "tokenUrl");
node.setTokenUrl(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "refreshUrl");
node.setRefreshUrl(value);
}
{
Map value = JsonUtil.consumeStringMapProperty(json, "scopes");
node.setScopes(value);
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readSecurityRequirement(ObjectNode json, AsyncApi26SecurityRequirement node) {
{
List propertyNames = JsonUtil.keys(json);
propertyNames.forEach(name -> {
List value = JsonUtil.consumeStringArrayProperty(json, name);
node.addItem(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readCorrelationID(ObjectNode json, AsyncApi26CorrelationID node) {
{
String value = JsonUtil.consumeStringProperty(json, "$ref");
node.set$ref(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "description");
node.setDescription(value);
}
{
String value = JsonUtil.consumeStringProperty(json, "location");
node.setLocation(value);
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
public void readBinding(ObjectNode json, AsyncApi26Binding node) {
{
List propertyNames = JsonUtil.keys(json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addItem(name, value);
});
}
{
List propertyNames = JsonUtil.matchingKeys("^x-.+$", json);
propertyNames.forEach(name -> {
JsonNode value = JsonUtil.consumeAnyProperty(json, name);
node.addExtension(name, value);
});
}
ReaderUtil.readExtraProperties(json, node);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy