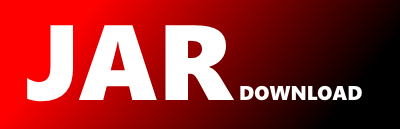
io.apicurio.registry.rest.client.models.BranchSearchResults Maven / Gradle / Ivy
package io.apicurio.registry.rest.client.models;
import com.microsoft.kiota.serialization.AdditionalDataHolder;
import com.microsoft.kiota.serialization.Parsable;
import com.microsoft.kiota.serialization.ParseNode;
import com.microsoft.kiota.serialization.SerializationWriter;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
/**
* Describes the response received when searching for branches.
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class BranchSearchResults implements AdditionalDataHolder, Parsable {
/**
* Stores additional data not described in the OpenAPI description found when deserializing. Can be used for serialization as well.
*/
private Map additionalData;
/**
* The branches returned in the result set.
*/
private java.util.List branches;
/**
* The total number of branches that matched the query that produced the result set (may be more than the number of branches in the result set).
*/
private Integer count;
/**
* Instantiates a new {@link BranchSearchResults} and sets the default values.
*/
public BranchSearchResults() {
this.setAdditionalData(new HashMap<>());
}
/**
* Creates a new instance of the appropriate class based on discriminator value
* @param parseNode The parse node to use to read the discriminator value and create the object
* @return a {@link BranchSearchResults}
*/
@jakarta.annotation.Nonnull
public static BranchSearchResults createFromDiscriminatorValue(@jakarta.annotation.Nonnull final ParseNode parseNode) {
Objects.requireNonNull(parseNode);
return new BranchSearchResults();
}
/**
* Gets the AdditionalData property value. Stores additional data not described in the OpenAPI description found when deserializing. Can be used for serialization as well.
* @return a {@link Map}
*/
@jakarta.annotation.Nonnull
public Map getAdditionalData() {
return this.additionalData;
}
/**
* Gets the branches property value. The branches returned in the result set.
* @return a {@link java.util.List}
*/
@jakarta.annotation.Nullable
public java.util.List getBranches() {
return this.branches;
}
/**
* Gets the count property value. The total number of branches that matched the query that produced the result set (may be more than the number of branches in the result set).
* @return a {@link Integer}
*/
@jakarta.annotation.Nullable
public Integer getCount() {
return this.count;
}
/**
* The deserialization information for the current model
* @return a {@link Map>}
*/
@jakarta.annotation.Nonnull
public Map> getFieldDeserializers() {
final HashMap> deserializerMap = new HashMap>(2);
deserializerMap.put("branches", (n) -> { this.setBranches(n.getCollectionOfObjectValues(SearchedBranch::createFromDiscriminatorValue)); });
deserializerMap.put("count", (n) -> { this.setCount(n.getIntegerValue()); });
return deserializerMap;
}
/**
* Serializes information the current object
* @param writer Serialization writer to use to serialize this model
*/
public void serialize(@jakarta.annotation.Nonnull final SerializationWriter writer) {
Objects.requireNonNull(writer);
writer.writeCollectionOfObjectValues("branches", this.getBranches());
writer.writeIntegerValue("count", this.getCount());
writer.writeAdditionalData(this.getAdditionalData());
}
/**
* Sets the AdditionalData property value. Stores additional data not described in the OpenAPI description found when deserializing. Can be used for serialization as well.
* @param value Value to set for the AdditionalData property.
*/
public void setAdditionalData(@jakarta.annotation.Nullable final Map value) {
this.additionalData = value;
}
/**
* Sets the branches property value. The branches returned in the result set.
* @param value Value to set for the branches property.
*/
public void setBranches(@jakarta.annotation.Nullable final java.util.List value) {
this.branches = value;
}
/**
* Sets the count property value. The total number of branches that matched the query that produced the result set (may be more than the number of branches in the result set).
* @param value Value to set for the count property.
*/
public void setCount(@jakarta.annotation.Nullable final Integer value) {
this.count = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy