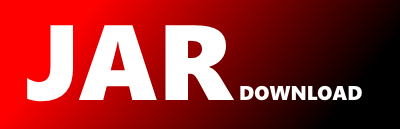
io.apicurio.registry.rest.client.models.CreateArtifact Maven / Gradle / Ivy
package io.apicurio.registry.rest.client.models;
import com.microsoft.kiota.serialization.AdditionalDataHolder;
import com.microsoft.kiota.serialization.Parsable;
import com.microsoft.kiota.serialization.ParseNode;
import com.microsoft.kiota.serialization.SerializationWriter;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
/**
* Data sent when creating a new artifact.
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class CreateArtifact implements AdditionalDataHolder, Parsable {
/**
* Stores additional data not described in the OpenAPI description found when deserializing. Can be used for serialization as well.
*/
private Map additionalData;
/**
* The ID of a single artifact.
*/
private String artifactId;
/**
* The artifactType property
*/
private String artifactType;
/**
* The description property
*/
private String description;
/**
* The firstVersion property
*/
private CreateVersion firstVersion;
/**
* User-defined name-value pairs. Name and value must be strings.
*/
private Labels labels;
/**
* The name property
*/
private String name;
/**
* Instantiates a new {@link CreateArtifact} and sets the default values.
*/
public CreateArtifact() {
this.setAdditionalData(new HashMap<>());
}
/**
* Creates a new instance of the appropriate class based on discriminator value
* @param parseNode The parse node to use to read the discriminator value and create the object
* @return a {@link CreateArtifact}
*/
@jakarta.annotation.Nonnull
public static CreateArtifact createFromDiscriminatorValue(@jakarta.annotation.Nonnull final ParseNode parseNode) {
Objects.requireNonNull(parseNode);
return new CreateArtifact();
}
/**
* Gets the AdditionalData property value. Stores additional data not described in the OpenAPI description found when deserializing. Can be used for serialization as well.
* @return a {@link Map}
*/
@jakarta.annotation.Nonnull
public Map getAdditionalData() {
return this.additionalData;
}
/**
* Gets the artifactId property value. The ID of a single artifact.
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getArtifactId() {
return this.artifactId;
}
/**
* Gets the artifactType property value. The artifactType property
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getArtifactType() {
return this.artifactType;
}
/**
* Gets the description property value. The description property
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getDescription() {
return this.description;
}
/**
* The deserialization information for the current model
* @return a {@link Map>}
*/
@jakarta.annotation.Nonnull
public Map> getFieldDeserializers() {
final HashMap> deserializerMap = new HashMap>(6);
deserializerMap.put("artifactId", (n) -> { this.setArtifactId(n.getStringValue()); });
deserializerMap.put("artifactType", (n) -> { this.setArtifactType(n.getStringValue()); });
deserializerMap.put("description", (n) -> { this.setDescription(n.getStringValue()); });
deserializerMap.put("firstVersion", (n) -> { this.setFirstVersion(n.getObjectValue(CreateVersion::createFromDiscriminatorValue)); });
deserializerMap.put("labels", (n) -> { this.setLabels(n.getObjectValue(Labels::createFromDiscriminatorValue)); });
deserializerMap.put("name", (n) -> { this.setName(n.getStringValue()); });
return deserializerMap;
}
/**
* Gets the firstVersion property value. The firstVersion property
* @return a {@link CreateVersion}
*/
@jakarta.annotation.Nullable
public CreateVersion getFirstVersion() {
return this.firstVersion;
}
/**
* Gets the labels property value. User-defined name-value pairs. Name and value must be strings.
* @return a {@link Labels}
*/
@jakarta.annotation.Nullable
public Labels getLabels() {
return this.labels;
}
/**
* Gets the name property value. The name property
* @return a {@link String}
*/
@jakarta.annotation.Nullable
public String getName() {
return this.name;
}
/**
* Serializes information the current object
* @param writer Serialization writer to use to serialize this model
*/
public void serialize(@jakarta.annotation.Nonnull final SerializationWriter writer) {
Objects.requireNonNull(writer);
writer.writeStringValue("artifactId", this.getArtifactId());
writer.writeStringValue("artifactType", this.getArtifactType());
writer.writeStringValue("description", this.getDescription());
writer.writeObjectValue("firstVersion", this.getFirstVersion());
writer.writeObjectValue("labels", this.getLabels());
writer.writeStringValue("name", this.getName());
writer.writeAdditionalData(this.getAdditionalData());
}
/**
* Sets the AdditionalData property value. Stores additional data not described in the OpenAPI description found when deserializing. Can be used for serialization as well.
* @param value Value to set for the AdditionalData property.
*/
public void setAdditionalData(@jakarta.annotation.Nullable final Map value) {
this.additionalData = value;
}
/**
* Sets the artifactId property value. The ID of a single artifact.
* @param value Value to set for the artifactId property.
*/
public void setArtifactId(@jakarta.annotation.Nullable final String value) {
this.artifactId = value;
}
/**
* Sets the artifactType property value. The artifactType property
* @param value Value to set for the artifactType property.
*/
public void setArtifactType(@jakarta.annotation.Nullable final String value) {
this.artifactType = value;
}
/**
* Sets the description property value. The description property
* @param value Value to set for the description property.
*/
public void setDescription(@jakarta.annotation.Nullable final String value) {
this.description = value;
}
/**
* Sets the firstVersion property value. The firstVersion property
* @param value Value to set for the firstVersion property.
*/
public void setFirstVersion(@jakarta.annotation.Nullable final CreateVersion value) {
this.firstVersion = value;
}
/**
* Sets the labels property value. User-defined name-value pairs. Name and value must be strings.
* @param value Value to set for the labels property.
*/
public void setLabels(@jakarta.annotation.Nullable final Labels value) {
this.labels = value;
}
/**
* Sets the name property value. The name property
* @param value Value to set for the name property.
*/
public void setName(@jakarta.annotation.Nullable final String value) {
this.name = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy