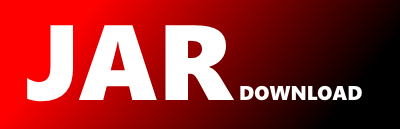
io.apicurio.registry.rest.client.search.versions.VersionsRequestBuilder Maven / Gradle / Ivy
package io.apicurio.registry.rest.client.search.versions;
import com.microsoft.kiota.BaseRequestBuilder;
import com.microsoft.kiota.BaseRequestConfiguration;
import com.microsoft.kiota.HttpMethod;
import com.microsoft.kiota.QueryParameters;
import com.microsoft.kiota.RequestAdapter;
import com.microsoft.kiota.RequestInformation;
import com.microsoft.kiota.RequestOption;
import com.microsoft.kiota.serialization.Parsable;
import com.microsoft.kiota.serialization.ParsableFactory;
import io.apicurio.registry.rest.client.models.ProblemDetails;
import io.apicurio.registry.rest.client.models.SortOrder;
import io.apicurio.registry.rest.client.models.VersionSearchResults;
import io.apicurio.registry.rest.client.models.VersionSortBy;
import io.apicurio.registry.rest.client.models.VersionState;
import java.io.InputStream;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
/**
* Search for versions in the registry.
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class VersionsRequestBuilder extends BaseRequestBuilder {
/**
* Instantiates a new {@link VersionsRequestBuilder} and sets the default values.
* @param pathParameters Path parameters for the request
* @param requestAdapter The request adapter to use to execute the requests.
*/
public VersionsRequestBuilder(@jakarta.annotation.Nonnull final HashMap pathParameters, @jakarta.annotation.Nonnull final RequestAdapter requestAdapter) {
super(requestAdapter, "{+baseurl}/search/versions{?artifactId*,artifactType*,canonical*,contentId*,description*,globalId*,groupId*,labels*,limit*,name*,offset*,order*,orderby*,state*,version*}", pathParameters);
}
/**
* Instantiates a new {@link VersionsRequestBuilder} and sets the default values.
* @param rawUrl The raw URL to use for the request builder.
* @param requestAdapter The request adapter to use to execute the requests.
*/
public VersionsRequestBuilder(@jakarta.annotation.Nonnull final String rawUrl, @jakarta.annotation.Nonnull final RequestAdapter requestAdapter) {
super(requestAdapter, "{+baseurl}/search/versions{?artifactId*,artifactType*,canonical*,contentId*,description*,globalId*,groupId*,labels*,limit*,name*,offset*,order*,orderby*,state*,version*}", rawUrl);
}
/**
* Returns a paginated list of all versions that match the provided filter criteria.This operation can fail for the following reasons:* A server error occurred (HTTP error `500`)
* @return a {@link VersionSearchResults}
* @throws ProblemDetails When receiving a 500 status code
*/
@jakarta.annotation.Nullable
public VersionSearchResults get() {
return get(null);
}
/**
* Returns a paginated list of all versions that match the provided filter criteria.This operation can fail for the following reasons:* A server error occurred (HTTP error `500`)
* @param requestConfiguration Configuration for the request such as headers, query parameters, and middleware options.
* @return a {@link VersionSearchResults}
* @throws ProblemDetails When receiving a 500 status code
*/
@jakarta.annotation.Nullable
public VersionSearchResults get(@jakarta.annotation.Nullable final java.util.function.Consumer requestConfiguration) {
final RequestInformation requestInfo = toGetRequestInformation(requestConfiguration);
final HashMap> errorMapping = new HashMap>();
errorMapping.put("500", ProblemDetails::createFromDiscriminatorValue);
return this.requestAdapter.send(requestInfo, errorMapping, VersionSearchResults::createFromDiscriminatorValue);
}
/**
* Returns a paginated list of all versions that match the posted content.This operation can fail for the following reasons:* Provided content (request body) was empty (HTTP error `400`)* A server error occurred (HTTP error `500`)
* @param body Binary request body
* @param contentType The request body content type.
* @return a {@link VersionSearchResults}
* @throws ProblemDetails When receiving a 400 status code
* @throws ProblemDetails When receiving a 500 status code
*/
@jakarta.annotation.Nullable
public VersionSearchResults post(@jakarta.annotation.Nonnull final InputStream body, @jakarta.annotation.Nonnull final String contentType) {
return post(body, contentType, null);
}
/**
* Returns a paginated list of all versions that match the posted content.This operation can fail for the following reasons:* Provided content (request body) was empty (HTTP error `400`)* A server error occurred (HTTP error `500`)
* @param body Binary request body
* @param contentType The request body content type.
* @param requestConfiguration Configuration for the request such as headers, query parameters, and middleware options.
* @return a {@link VersionSearchResults}
* @throws ProblemDetails When receiving a 400 status code
* @throws ProblemDetails When receiving a 500 status code
*/
@jakarta.annotation.Nullable
public VersionSearchResults post(@jakarta.annotation.Nonnull final InputStream body, @jakarta.annotation.Nonnull final String contentType, @jakarta.annotation.Nullable final java.util.function.Consumer requestConfiguration) {
Objects.requireNonNull(body);
Objects.requireNonNull(contentType);
final RequestInformation requestInfo = toPostRequestInformation(body, contentType, requestConfiguration);
final HashMap> errorMapping = new HashMap>();
errorMapping.put("400", ProblemDetails::createFromDiscriminatorValue);
errorMapping.put("500", ProblemDetails::createFromDiscriminatorValue);
return this.requestAdapter.send(requestInfo, errorMapping, VersionSearchResults::createFromDiscriminatorValue);
}
/**
* Returns a paginated list of all versions that match the provided filter criteria.This operation can fail for the following reasons:* A server error occurred (HTTP error `500`)
* @return a {@link RequestInformation}
*/
@jakarta.annotation.Nonnull
public RequestInformation toGetRequestInformation() {
return toGetRequestInformation(null);
}
/**
* Returns a paginated list of all versions that match the provided filter criteria.This operation can fail for the following reasons:* A server error occurred (HTTP error `500`)
* @param requestConfiguration Configuration for the request such as headers, query parameters, and middleware options.
* @return a {@link RequestInformation}
*/
@jakarta.annotation.Nonnull
public RequestInformation toGetRequestInformation(@jakarta.annotation.Nullable final java.util.function.Consumer requestConfiguration) {
final RequestInformation requestInfo = new RequestInformation(HttpMethod.GET, urlTemplate, pathParameters);
requestInfo.configure(requestConfiguration, GetRequestConfiguration::new, x -> x.queryParameters);
requestInfo.headers.tryAdd("Accept", "application/json");
return requestInfo;
}
/**
* Returns a paginated list of all versions that match the posted content.This operation can fail for the following reasons:* Provided content (request body) was empty (HTTP error `400`)* A server error occurred (HTTP error `500`)
* @param body Binary request body
* @param contentType The request body content type.
* @return a {@link RequestInformation}
*/
@jakarta.annotation.Nonnull
public RequestInformation toPostRequestInformation(@jakarta.annotation.Nonnull final InputStream body, @jakarta.annotation.Nonnull final String contentType) {
return toPostRequestInformation(body, contentType, null);
}
/**
* Returns a paginated list of all versions that match the posted content.This operation can fail for the following reasons:* Provided content (request body) was empty (HTTP error `400`)* A server error occurred (HTTP error `500`)
* @param body Binary request body
* @param contentType The request body content type.
* @param requestConfiguration Configuration for the request such as headers, query parameters, and middleware options.
* @return a {@link RequestInformation}
*/
@jakarta.annotation.Nonnull
public RequestInformation toPostRequestInformation(@jakarta.annotation.Nonnull final InputStream body, @jakarta.annotation.Nonnull final String contentType, @jakarta.annotation.Nullable final java.util.function.Consumer requestConfiguration) {
Objects.requireNonNull(body);
Objects.requireNonNull(contentType);
final RequestInformation requestInfo = new RequestInformation(HttpMethod.POST, urlTemplate, pathParameters);
requestInfo.configure(requestConfiguration, PostRequestConfiguration::new, x -> x.queryParameters);
requestInfo.headers.tryAdd("Accept", "application/json");
requestInfo.setStreamContent(body, contentType);
return requestInfo;
}
/**
* Returns a request builder with the provided arbitrary URL. Using this method means any other path or query parameters are ignored.
* @param rawUrl The raw URL to use for the request builder.
* @return a {@link VersionsRequestBuilder}
*/
@jakarta.annotation.Nonnull
public VersionsRequestBuilder withUrl(@jakarta.annotation.Nonnull final String rawUrl) {
Objects.requireNonNull(rawUrl);
return new VersionsRequestBuilder(rawUrl, requestAdapter);
}
/**
* Returns a paginated list of all versions that match the provided filter criteria.This operation can fail for the following reasons:* A server error occurred (HTTP error `500`)
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class GetQueryParameters implements QueryParameters {
/**
* Filter by artifactId.
*/
@jakarta.annotation.Nullable
public String artifactId;
/**
* Filter by contentId.
*/
@jakarta.annotation.Nullable
public Long contentId;
/**
* Filter by description.
*/
@jakarta.annotation.Nullable
public String description;
/**
* Filter by globalId.
*/
@jakarta.annotation.Nullable
public Long globalId;
/**
* Filter by artifact group.
*/
@jakarta.annotation.Nullable
public String groupId;
/**
* Filter by one or more name/value label. Separate each name/value pair using a colon. Forexample `labels=foo:bar` will return only artifacts with a label named `foo`and value `bar`.
*/
@jakarta.annotation.Nullable
public String[] labels;
/**
* The number of versions to return. Defaults to 20.
*/
@jakarta.annotation.Nullable
public Integer limit;
/**
* Filter by name.
*/
@jakarta.annotation.Nullable
public String name;
/**
* The number of versions to skip before starting to collect the result set. Defaults to 0.
*/
@jakarta.annotation.Nullable
public Integer offset;
/**
* Sort order, ascending (`asc`) or descending (`desc`).
*/
@jakarta.annotation.Nullable
public SortOrder order;
/**
* The field to sort by. Can be one of:* `name`* `createdOn`
*/
@jakarta.annotation.Nullable
public VersionSortBy orderby;
/**
* Filter by version state.
*/
@jakarta.annotation.Nullable
public VersionState state;
/**
* Filter by version number.
*/
@jakarta.annotation.Nullable
public String version;
/**
* Extracts the query parameters into a map for the URI template parsing.
* @return a {@link Map}
*/
@jakarta.annotation.Nonnull
public Map toQueryParameters() {
final Map allQueryParams = new HashMap();
allQueryParams.put("order", order);
allQueryParams.put("orderby", orderby);
allQueryParams.put("state", state);
allQueryParams.put("artifactId", artifactId);
allQueryParams.put("contentId", contentId);
allQueryParams.put("description", description);
allQueryParams.put("globalId", globalId);
allQueryParams.put("groupId", groupId);
allQueryParams.put("limit", limit);
allQueryParams.put("name", name);
allQueryParams.put("offset", offset);
allQueryParams.put("version", version);
allQueryParams.put("labels", labels);
return allQueryParams;
}
}
/**
* Configuration for the request such as headers, query parameters, and middleware options.
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class GetRequestConfiguration extends BaseRequestConfiguration {
/**
* Request query parameters
*/
@jakarta.annotation.Nullable
public GetQueryParameters queryParameters = new GetQueryParameters();
}
/**
* Returns a paginated list of all versions that match the posted content.This operation can fail for the following reasons:* Provided content (request body) was empty (HTTP error `400`)* A server error occurred (HTTP error `500`)
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class PostQueryParameters implements QueryParameters {
/**
* Filter by artifact Id.
*/
@jakarta.annotation.Nullable
public String artifactId;
/**
* Indicates the type of artifact represented by the content being used for the search. This is only needed when using the `canonical` query parameter, so that the server knows how to canonicalize the content prior to searching for matching versions.
*/
@jakarta.annotation.Nullable
public String artifactType;
/**
* Parameter that can be set to `true` to indicate that the server should "canonicalize" the content when searching for matching artifacts. Canonicalization is unique to each artifact type, but typically involves removing any extra whitespace and formatting the content in a consistent manner. Must be used along with the `artifactType` query parameter.
*/
@jakarta.annotation.Nullable
public Boolean canonical;
/**
* Filter by group Id.
*/
@jakarta.annotation.Nullable
public String groupId;
/**
* The number of versions to return. Defaults to 20.
*/
@jakarta.annotation.Nullable
public Integer limit;
/**
* The number of versions to skip before starting to collect the result set. Defaults to 0.
*/
@jakarta.annotation.Nullable
public Integer offset;
/**
* Sort order, ascending (`asc`) or descending (`desc`).
*/
@jakarta.annotation.Nullable
public SortOrder order;
/**
* The field to sort by. Can be one of:* `name`* `createdOn`
*/
@jakarta.annotation.Nullable
public VersionSortBy orderby;
/**
* Extracts the query parameters into a map for the URI template parsing.
* @return a {@link Map}
*/
@jakarta.annotation.Nonnull
public Map toQueryParameters() {
final Map allQueryParams = new HashMap();
allQueryParams.put("order", order);
allQueryParams.put("orderby", orderby);
allQueryParams.put("artifactId", artifactId);
allQueryParams.put("artifactType", artifactType);
allQueryParams.put("canonical", canonical);
allQueryParams.put("groupId", groupId);
allQueryParams.put("limit", limit);
allQueryParams.put("offset", offset);
return allQueryParams;
}
}
/**
* Configuration for the request such as headers, query parameters, and middleware options.
*/
@jakarta.annotation.Generated("com.microsoft.kiota")
public class PostRequestConfiguration extends BaseRequestConfiguration {
/**
* Request query parameters
*/
@jakarta.annotation.Nullable
public PostQueryParameters queryParameters = new PostQueryParameters();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy