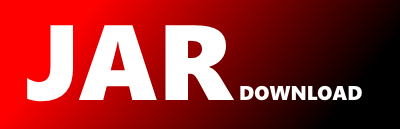
com.apigee.mgmtapi.sdk.client.MgmtAPIClient Maven / Gradle / Ivy
package com.apigee.mgmtapi.sdk.client;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.nio.charset.Charset;
import org.apache.log4j.Logger;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import org.springframework.context.support.AbstractApplicationContext;
import org.springframework.core.env.Environment;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.security.crypto.codec.Base64;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestTemplate;
import com.apigee.mgmtapi.sdk.core.AppConfig;
import com.apigee.mgmtapi.sdk.model.AccessToken;
import com.apigee.mgmtapi.sdk.service.FileService;
import com.google.auth.oauth2.GoogleCredentials;
import com.google.gson.Gson;
public class MgmtAPIClient {
private static final Logger logger = Logger.getLogger(MgmtAPIClient.class);
/**
* To get the Access Token Management URL, client_id and client_secret needs
* to be passed through a config file whose full path is passed as system
* property like -DconfigFile.path="/to/dir/config.properties"
*
* @param username
* @param password
* @return
* @throws Exception
*/
public AccessToken getAccessToken(String username, String password) throws Exception {
Environment env = this.getConfigProperties();
if (env == null) {
logger.error("Config file missing");
throw new Exception("Config file missing");
}
return getAccessToken(env.getProperty("mgmt.login.url"), env.getProperty("mgmt.login.client.id"),
env.getProperty("mgmt.login.client.secret"), username, password);
}
/**
* To get the Access Token Management URL, client_id and client_secret needs
* to be passed through a config file whose full path is passed as system
* property like -DconfigFile.path="/to/dir/config.properties"
*
* @param username
* @param password
* @param mfa
* @return
* @throws Exception
*/
public AccessToken getAccessToken(String username, String password, String mfa) throws Exception {
Environment env = this.getConfigProperties();
if (env == null) {
logger.error("Config file missing");
throw new Exception("Config file missing");
}
if (mfa == null || mfa.equals("")) {
logger.error("mfa cannot be empty");
throw new Exception("mfa cannot be empty");
}
return getAccessToken(env.getProperty("mgmt.login.mfa.url")+mfa, env.getProperty("mgmt.login.client.id"),
env.getProperty("mgmt.login.client.secret"), username, password);
}
/**
* To get Access Token
* @param url
* @param clientId
* @param client_secret
* @param username
* @param password
* @param mfa
* @return
* @throws Exception
*/
public AccessToken getAccessToken(String url, String clientId, String client_secret, String username,
String password, String mfa) throws Exception {
return getAccessToken(url+"?mfa_token="+mfa, clientId, client_secret, username, password);
}
/**
* To get the Access Token
*
* @param url
* @param clientId
* @param client_secret
* @param username
* @param password
* @return
* @throws Exception
*/
public AccessToken getAccessToken(String url, String clientId, String client_secret, String username,
String password) throws Exception {
RestTemplate restTemplate = new RestTemplate();
HttpHeaders headers = new HttpHeaders();
AccessToken token = new AccessToken();
ResponseEntity result = null;
try {
headers.add("Authorization", "Basic "
+ new String(Base64.encode((clientId + ":" + client_secret).getBytes()), Charset.forName("UTF-8")));
headers.add("Content-Type", "application/x-www-form-urlencoded");
MultiValueMap map = new LinkedMultiValueMap();
map.add("username", username);
map.add("password", password);
map.add("grant_type", "password");
HttpEntity
© 2015 - 2025 Weber Informatics LLC | Privacy Policy