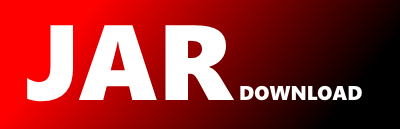
io.appwrite.models.File.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk-for-kotlin Show documentation
Show all versions of sdk-for-kotlin Show documentation
Appwrite is an open-source backend as a service server that abstract and simplify complex and repetitive development tasks behind a very simple to use REST API. Appwrite aims to help you develop your apps faster and in a more secure way. Use the Kotlin SDK to integrate your app with the Appwrite server to easily start interacting with all of Appwrite backend APIs and tools. For full API documentation and tutorials go to [https://appwrite.io/docs](https://appwrite.io/docs)
package io.appwrite.models
import com.google.gson.annotations.SerializedName
import io.appwrite.extensions.jsonCast
/**
* File
*/
data class File(
/**
* File ID.
*/
@SerializedName("\$id")
val id: String,
/**
* Bucket ID.
*/
@SerializedName("bucketId")
val bucketId: String,
/**
* File creation date in ISO 8601 format.
*/
@SerializedName("\$createdAt")
val createdAt: String,
/**
* File update date in ISO 8601 format.
*/
@SerializedName("\$updatedAt")
val updatedAt: String,
/**
* File permissions. [Learn more about permissions](/docs/permissions).
*/
@SerializedName("\$permissions")
val permissions: List,
/**
* File name.
*/
@SerializedName("name")
val name: String,
/**
* File MD5 signature.
*/
@SerializedName("signature")
val signature: String,
/**
* File mime type.
*/
@SerializedName("mimeType")
val mimeType: String,
/**
* File original size in bytes.
*/
@SerializedName("sizeOriginal")
val sizeOriginal: Long,
/**
* Total number of chunks available
*/
@SerializedName("chunksTotal")
val chunksTotal: Long,
/**
* Total number of chunks uploaded
*/
@SerializedName("chunksUploaded")
val chunksUploaded: Long,
) {
fun toMap(): Map = mapOf(
"\$id" to id as Any,
"bucketId" to bucketId as Any,
"\$createdAt" to createdAt as Any,
"\$updatedAt" to updatedAt as Any,
"\$permissions" to permissions as Any,
"name" to name as Any,
"signature" to signature as Any,
"mimeType" to mimeType as Any,
"sizeOriginal" to sizeOriginal as Any,
"chunksTotal" to chunksTotal as Any,
"chunksUploaded" to chunksUploaded as Any,
)
companion object {
@Suppress("UNCHECKED_CAST")
fun from(
map: Map,
) = File(
id = map["\$id"] as String,
bucketId = map["bucketId"] as String,
createdAt = map["\$createdAt"] as String,
updatedAt = map["\$updatedAt"] as String,
permissions = map["\$permissions"] as List,
name = map["name"] as String,
signature = map["signature"] as String,
mimeType = map["mimeType"] as String,
sizeOriginal = (map["sizeOriginal"] as Number).toLong(),
chunksTotal = (map["chunksTotal"] as Number).toLong(),
chunksUploaded = (map["chunksUploaded"] as Number).toLong(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy