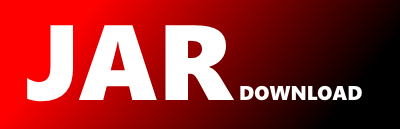
io.appwrite.models.User.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk-for-kotlin Show documentation
Show all versions of sdk-for-kotlin Show documentation
Appwrite is an open-source backend as a service server that abstract and simplify complex and repetitive development tasks behind a very simple to use REST API. Appwrite aims to help you develop your apps faster and in a more secure way. Use the Kotlin SDK to integrate your app with the Appwrite server to easily start interacting with all of Appwrite backend APIs and tools. For full API documentation and tutorials go to [https://appwrite.io/docs](https://appwrite.io/docs)
package io.appwrite.models
import com.google.gson.annotations.SerializedName
import io.appwrite.extensions.jsonCast
/**
* User
*/
data class User(
/**
* User ID.
*/
@SerializedName("\$id")
val id: String,
/**
* User creation date in ISO 8601 format.
*/
@SerializedName("\$createdAt")
val createdAt: String,
/**
* User update date in ISO 8601 format.
*/
@SerializedName("\$updatedAt")
val updatedAt: String,
/**
* User name.
*/
@SerializedName("name")
val name: String,
/**
* Hashed user password.
*/
@SerializedName("password")
var password: String?,
/**
* Password hashing algorithm.
*/
@SerializedName("hash")
var hash: String?,
/**
* Password hashing algorithm configuration.
*/
@SerializedName("hashOptions")
var hashOptions: Any?,
/**
* User registration date in ISO 8601 format.
*/
@SerializedName("registration")
val registration: String,
/**
* User status. Pass `true` for enabled and `false` for disabled.
*/
@SerializedName("status")
val status: Boolean,
/**
* Labels for the user.
*/
@SerializedName("labels")
val labels: List,
/**
* Password update time in ISO 8601 format.
*/
@SerializedName("passwordUpdate")
val passwordUpdate: String,
/**
* User email address.
*/
@SerializedName("email")
val email: String,
/**
* User phone number in E.164 format.
*/
@SerializedName("phone")
val phone: String,
/**
* Email verification status.
*/
@SerializedName("emailVerification")
val emailVerification: Boolean,
/**
* Phone verification status.
*/
@SerializedName("phoneVerification")
val phoneVerification: Boolean,
/**
* User preferences as a key-value object
*/
@SerializedName("prefs")
val prefs: Preferences,
/**
* Most recent access date in ISO 8601 format. This attribute is only updated again after 24 hours.
*/
@SerializedName("accessedAt")
val accessedAt: String,
) {
fun toMap(): Map = mapOf(
"\$id" to id as Any,
"\$createdAt" to createdAt as Any,
"\$updatedAt" to updatedAt as Any,
"name" to name as Any,
"password" to password as Any,
"hash" to hash as Any,
"hashOptions" to hashOptions as Any,
"registration" to registration as Any,
"status" to status as Any,
"labels" to labels as Any,
"passwordUpdate" to passwordUpdate as Any,
"email" to email as Any,
"phone" to phone as Any,
"emailVerification" to emailVerification as Any,
"phoneVerification" to phoneVerification as Any,
"prefs" to prefs.toMap() as Any,
"accessedAt" to accessedAt as Any,
)
companion object {
operator fun invoke(
id: String,
createdAt: String,
updatedAt: String,
name: String,
password: String?,
hash: String?,
hashOptions: Any?,
registration: String,
status: Boolean,
labels: List,
passwordUpdate: String,
email: String,
phone: String,
emailVerification: Boolean,
phoneVerification: Boolean,
prefs: Preferences
© 2015 - 2024 Weber Informatics LLC | Privacy Policy