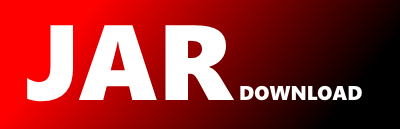
io.appwrite.services.Graphql.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk-for-kotlin Show documentation
Show all versions of sdk-for-kotlin Show documentation
Appwrite is an open-source backend as a service server that abstract and simplify complex and repetitive development tasks behind a very simple to use REST API. Appwrite aims to help you develop your apps faster and in a more secure way. Use the Kotlin SDK to integrate your app with the Appwrite server to easily start interacting with all of Appwrite backend APIs and tools. For full API documentation and tutorials go to [https://appwrite.io/docs](https://appwrite.io/docs)
package io.appwrite.services
import io.appwrite.Client
import io.appwrite.models.*
import io.appwrite.exceptions.AppwriteException
import io.appwrite.extensions.classOf
import okhttp3.Cookie
import java.io.File
/**
* The GraphQL API allows you to query and mutate your Appwrite server using GraphQL.
**/
class Graphql : Service {
public constructor (client: Client) : super(client) { }
/**
* GraphQL Endpoint
*
* Execute a GraphQL mutation.
*
* @param query The query or queries to execute.
* @return [Any]
*/
@Throws(AppwriteException::class)
suspend fun query(
query: Any,
): Any {
val apiPath = "/graphql"
val apiParams = mutableMapOf(
"query" to query,
)
val apiHeaders = mutableMapOf(
"x-sdk-graphql" to "true",
"content-type" to "application/json",
)
val converter: (Any) -> Any = {
it
}
return client.call(
"POST",
apiPath,
apiHeaders,
apiParams,
responseType = Any::class.java,
converter,
)
}
/**
* GraphQL Endpoint
*
* Execute a GraphQL mutation.
*
* @param query The query or queries to execute.
* @return [Any]
*/
@Throws(AppwriteException::class)
suspend fun mutation(
query: Any,
): Any {
val apiPath = "/graphql/mutation"
val apiParams = mutableMapOf(
"query" to query,
)
val apiHeaders = mutableMapOf(
"x-sdk-graphql" to "true",
"content-type" to "application/json",
)
val converter: (Any) -> Any = {
it
}
return client.call(
"POST",
apiPath,
apiHeaders,
apiParams,
responseType = Any::class.java,
converter,
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy