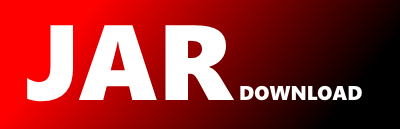
io.appwrite.services.Locale.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk-for-kotlin Show documentation
Show all versions of sdk-for-kotlin Show documentation
Appwrite is an open-source backend as a service server that abstract and simplify complex and repetitive development tasks behind a very simple to use REST API. Appwrite aims to help you develop your apps faster and in a more secure way. Use the Kotlin SDK to integrate your app with the Appwrite server to easily start interacting with all of Appwrite backend APIs and tools. For full API documentation and tutorials go to [https://appwrite.io/docs](https://appwrite.io/docs)
package io.appwrite.services
import io.appwrite.Client
import io.appwrite.models.*
import io.appwrite.exceptions.AppwriteException
import io.appwrite.extensions.classOf
import okhttp3.Cookie
import java.io.File
/**
* The Locale service allows you to customize your app based on your users' location.
**/
class Locale : Service {
public constructor (client: Client) : super(client) { }
/**
* Get User Locale
*
* Get the current user location based on IP. Returns an object with user country code, country name, continent name, continent code, ip address and suggested currency. You can use the locale header to get the data in a supported language.([IP Geolocation by DB-IP](https://db-ip.com))
*
* @return [io.appwrite.models.Locale]
*/
@Throws(AppwriteException::class)
suspend fun get(
): io.appwrite.models.Locale {
val apiPath = "/locale"
val apiParams = mutableMapOf(
)
val apiHeaders = mutableMapOf(
"content-type" to "application/json",
)
val converter: (Any) -> io.appwrite.models.Locale = {
io.appwrite.models.Locale.from(map = it as Map)
}
return client.call(
"GET",
apiPath,
apiHeaders,
apiParams,
responseType = io.appwrite.models.Locale::class.java,
converter,
)
}
/**
* List Locale Codes
*
* List of all locale codes in [ISO 639-1](https://en.wikipedia.org/wiki/List_of_ISO_639-1_codes).
*
* @return [io.appwrite.models.LocaleCodeList]
*/
@Throws(AppwriteException::class)
suspend fun listCodes(
): io.appwrite.models.LocaleCodeList {
val apiPath = "/locale/codes"
val apiParams = mutableMapOf(
)
val apiHeaders = mutableMapOf(
"content-type" to "application/json",
)
val converter: (Any) -> io.appwrite.models.LocaleCodeList = {
io.appwrite.models.LocaleCodeList.from(map = it as Map)
}
return client.call(
"GET",
apiPath,
apiHeaders,
apiParams,
responseType = io.appwrite.models.LocaleCodeList::class.java,
converter,
)
}
/**
* List Continents
*
* List of all continents. You can use the locale header to get the data in a supported language.
*
* @return [io.appwrite.models.ContinentList]
*/
@Throws(AppwriteException::class)
suspend fun listContinents(
): io.appwrite.models.ContinentList {
val apiPath = "/locale/continents"
val apiParams = mutableMapOf(
)
val apiHeaders = mutableMapOf(
"content-type" to "application/json",
)
val converter: (Any) -> io.appwrite.models.ContinentList = {
io.appwrite.models.ContinentList.from(map = it as Map)
}
return client.call(
"GET",
apiPath,
apiHeaders,
apiParams,
responseType = io.appwrite.models.ContinentList::class.java,
converter,
)
}
/**
* List Countries
*
* List of all countries. You can use the locale header to get the data in a supported language.
*
* @return [io.appwrite.models.CountryList]
*/
@Throws(AppwriteException::class)
suspend fun listCountries(
): io.appwrite.models.CountryList {
val apiPath = "/locale/countries"
val apiParams = mutableMapOf(
)
val apiHeaders = mutableMapOf(
"content-type" to "application/json",
)
val converter: (Any) -> io.appwrite.models.CountryList = {
io.appwrite.models.CountryList.from(map = it as Map)
}
return client.call(
"GET",
apiPath,
apiHeaders,
apiParams,
responseType = io.appwrite.models.CountryList::class.java,
converter,
)
}
/**
* List EU Countries
*
* List of all countries that are currently members of the EU. You can use the locale header to get the data in a supported language.
*
* @return [io.appwrite.models.CountryList]
*/
@Throws(AppwriteException::class)
suspend fun listCountriesEU(
): io.appwrite.models.CountryList {
val apiPath = "/locale/countries/eu"
val apiParams = mutableMapOf(
)
val apiHeaders = mutableMapOf(
"content-type" to "application/json",
)
val converter: (Any) -> io.appwrite.models.CountryList = {
io.appwrite.models.CountryList.from(map = it as Map)
}
return client.call(
"GET",
apiPath,
apiHeaders,
apiParams,
responseType = io.appwrite.models.CountryList::class.java,
converter,
)
}
/**
* List Countries Phone Codes
*
* List of all countries phone codes. You can use the locale header to get the data in a supported language.
*
* @return [io.appwrite.models.PhoneList]
*/
@Throws(AppwriteException::class)
suspend fun listCountriesPhones(
): io.appwrite.models.PhoneList {
val apiPath = "/locale/countries/phones"
val apiParams = mutableMapOf(
)
val apiHeaders = mutableMapOf(
"content-type" to "application/json",
)
val converter: (Any) -> io.appwrite.models.PhoneList = {
io.appwrite.models.PhoneList.from(map = it as Map)
}
return client.call(
"GET",
apiPath,
apiHeaders,
apiParams,
responseType = io.appwrite.models.PhoneList::class.java,
converter,
)
}
/**
* List Currencies
*
* List of all currencies, including currency symbol, name, plural, and decimal digits for all major and minor currencies. You can use the locale header to get the data in a supported language.
*
* @return [io.appwrite.models.CurrencyList]
*/
@Throws(AppwriteException::class)
suspend fun listCurrencies(
): io.appwrite.models.CurrencyList {
val apiPath = "/locale/currencies"
val apiParams = mutableMapOf(
)
val apiHeaders = mutableMapOf(
"content-type" to "application/json",
)
val converter: (Any) -> io.appwrite.models.CurrencyList = {
io.appwrite.models.CurrencyList.from(map = it as Map)
}
return client.call(
"GET",
apiPath,
apiHeaders,
apiParams,
responseType = io.appwrite.models.CurrencyList::class.java,
converter,
)
}
/**
* List Languages
*
* List of all languages classified by ISO 639-1 including 2-letter code, name in English, and name in the respective language.
*
* @return [io.appwrite.models.LanguageList]
*/
@Throws(AppwriteException::class)
suspend fun listLanguages(
): io.appwrite.models.LanguageList {
val apiPath = "/locale/languages"
val apiParams = mutableMapOf(
)
val apiHeaders = mutableMapOf(
"content-type" to "application/json",
)
val converter: (Any) -> io.appwrite.models.LanguageList = {
io.appwrite.models.LanguageList.from(map = it as Map)
}
return client.call(
"GET",
apiPath,
apiHeaders,
apiParams,
responseType = io.appwrite.models.LanguageList::class.java,
converter,
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy