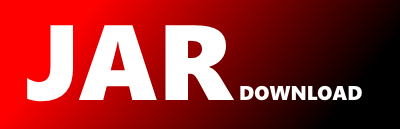
commonMain.arrow.core.MemoizedDeepRecursiveFunction.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arrow-core Show documentation
Show all versions of arrow-core Show documentation
Functional companion to Kotlin's Standard Library
package arrow.core
import arrow.atomic.Atomic
import arrow.atomic.updateAndGet
import kotlin.jvm.JvmInline
import kotlin.jvm.JvmOverloads
public interface MemoizationCache {
public fun get(key: K): V?
public fun set(key: K, value: V): V
}
@JvmInline
public value class AtomicMemoizationCache(
private val cache: Atomic
© 2015 - 2025 Weber Informatics LLC | Privacy Policy