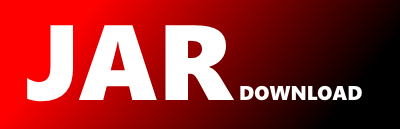
arrow.data.Coproduct.kt Maven / Gradle / Ivy
package arrow.data
import arrow.*
import arrow.core.*
import arrow.typeclasses.*
@higherkind data class Coproduct(val run: Either, HK>) : CoproductKind, CoproductKindedJ {
fun map(CF: Functor, CG: Functor, f: (A) -> B): Coproduct = Coproduct(run.bimap(CF.lift(f), CG.lift(f)))
fun coflatMap(CF: Comonad, CG: Comonad, f: (Coproduct) -> B): Coproduct =
Coproduct(run.bimap(
{ CF.coflatMap(it, { f(Coproduct(Left(it))) }) },
{ CG.coflatMap(it, { f(Coproduct(Right(it))) }) }
))
fun extract(CF: Comonad, CG: Comonad): A = run.fold({ CF.extract(it) }, { CG.extract(it) })
fun fold(f: FunctionK, g: FunctionK): HK = run.fold({ f(it) }, { g(it) })
fun foldLeft(b: B, f: (B, A) -> B, FF: Foldable, FG: Foldable): B = run.fold({ FF.foldLeft(it, b, f) }, { FG.foldLeft(it, b, f) })
fun foldRight(lb: Eval, f: (A, Eval) -> Eval, FF: Foldable, FG: Foldable): Eval =
run.fold({ FF.foldRight(it, lb, f) }, { FG.foldRight(it, lb, f) })
fun traverse(f: (A) -> HK, GA: Applicative, FT: Traverse, GT: Traverse): HK> =
run.fold({
GA.map(FT.traverse(it, f, GA), { Coproduct(Left(it)) })
}, {
GA.map(GT.traverse(it, f, GA), { Coproduct(Right(it)) })
})
companion object {
inline operator fun invoke(run: Either, HK>): Coproduct = Coproduct(run)
}
}
inline fun Either, HK>.coproduct(): Coproduct =
Coproduct(this)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy