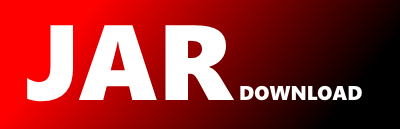
arrow.data.Reader.kt Maven / Gradle / Ivy
package arrow.data
import arrow.core.*
import arrow.typeclasses.*
/**
* Alias that represents a computation that has a dependency on [D].
*/
typealias ReaderFun = (D) -> A
/**
* Alias ReaderHK for [ReaderTHK]
*
* @see ReaderTHK
*/
typealias ReaderHK = ReaderTHK
/**
* Alias ReaderKind for [ReaderTKind]
*
* @see ReaderTKind
*/
typealias ReaderKind = ReaderTKind
/**
* Alias to partially apply type parameter [D] to [Reader].
*
* @see ReaderTKindPartial
*/
typealias ReaderKindPartial = ReaderTKindPartial
/**
* [Reader] represents a computation that has a dependency on [D].
* `Reader` is an alias for `ReaderT` and `Kleisli`.
*
* @param D the dependency or environment we depend on.
* @param A resulting type of the computation.
* @see ReaderT
*/
typealias Reader = ReaderT
/**
* Constructor for [Reader].
*
* @param run the dependency dependent computation.
*/
@Suppress("FunctionName")
fun Reader(run: ReaderFun): Reader = ReaderT(run.andThen { Id(it) })
/**
* Syntax for constructing a [Reader]
*
* @receiver [ReaderFun] a function that represents computation dependent on type [D].
*/
fun (ReaderFun).reader(): Reader = Reader(this)
/**
* Alias for [Kleisli.run]
*
* @param d dependency to runId the computation.
*/
fun Reader.runId(d: D): A = this.run(d).value()
/**
* Map the result of the computation [A] to [B] given a function [f].
*
* @param f the function to apply.
*/
fun Reader.map(f: (A) -> B): Reader = map(f, functor())
/**
* FlatMap the result of the computation [A] to another [Reader] for the same dependency [D] and flatten the structure.
*
* @param f the function to apply.
*/
fun Reader.flatMap(f: (A) -> Reader): Reader = flatMap(f, monad())
/**
* Apply a function `(A) -> B` that operates within the context of [Reader].
*
* @param ff function that maps [A] to [B] within the [Reader] context.
*/
fun Reader.ap(ff: ReaderKind B>): Reader = ap(ff, applicative())
/**
* Zip with another [Reader].
*
* @param o other [Reader] to zip with.
*/
fun Reader.zip(o: Reader): Reader> = zip(o, monad())
/**
* Compose with another [Reader] that has a dependency on the output of the computation.
*
* @param o other [Reader] to compose with.
*/
fun Reader.andThen(o: Reader): Reader = andThen(o, monad())
/**
* Map the result of the computation [A] to [B] given a function [f].
* Alias for [map]
*
* @param f the function to apply.
* @see map
*/
fun Reader.andThen(f: (A) -> B): Reader = map(f)
/**
* Set the result to [B] after running the computation.
*/
fun Reader.andThen(b: B): Reader = map { _ -> b }
@Suppress("FunctionName")
fun Reader(): ReaderApi = ReaderApi
object ReaderApi {
/**
* Alias for[ReaderT.Companion.applicative]
*/
fun applicative(): Applicative> = arrow.typeclasses.applicative()
/**
* Alias for [ReaderT.Companion.functor]
*/
fun functor(): Functor> = arrow.typeclasses.functor()
/**
* Alias for [ReaderT.Companion.monad]
*/
fun monad(): Monad> = arrow.typeclasses.monad()
fun pure(x: A): Reader = ReaderT.pure(x, arrow.typeclasses.monad())
fun ask(): Reader = ReaderT.ask(arrow.typeclasses.monad())
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy