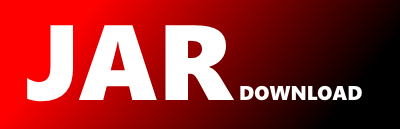
commonMain.arrow.optics.Iso.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arrow-optics-jvm Show documentation
Show all versions of arrow-optics-jvm Show documentation
Functional companion to Kotlin's Standard Library
package arrow.optics
import arrow.core.Either
import arrow.core.NonEmptyList
import arrow.core.None
import arrow.core.Option
import arrow.core.Either.Right
import arrow.core.Some
import arrow.core.Validated
import arrow.core.Validated.Invalid
import arrow.core.Validated.Valid
import arrow.core.compose
import arrow.core.identity
import arrow.typeclasses.Monoid
import kotlin.jvm.JvmStatic
/**
* [Iso] is a type alias for [PIso] which fixes the type arguments
* and restricts the [PIso] to monomorphic updates.
*/
public typealias Iso = PIso
private val stringToList: Iso> =
Iso(
get = CharSequence::toList,
reverseGet = { it.joinToString(separator = "") }
)
/**
* An [Iso] is a loss less invertible optic that defines an isomorphism between a type [S] and [A]
* i.e. a data class and its properties represented by TupleN
*
* A (polymorphic) [PIso] is useful when setting or modifying a value for a constructed type
* i.e. PIso
© 2015 - 2025 Weber Informatics LLC | Privacy Policy