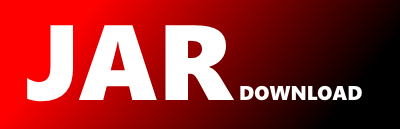
commonMain.arrow.optics.Traversal.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arrow-optics-jvm Show documentation
Show all versions of arrow-optics-jvm Show documentation
Functional companion to Kotlin's Standard Library
package arrow.optics
import arrow.core.Either
import arrow.core.NonEmptyList
import arrow.core.Option
import arrow.core.Tuple10
import arrow.core.Tuple4
import arrow.core.Tuple5
import arrow.core.Tuple6
import arrow.core.Tuple7
import arrow.core.Tuple8
import arrow.core.Tuple9
import kotlin.jvm.JvmStatic
/**
* [Traversal] is a type alias for [PTraversal] which fixes the type arguments
* and restricts the [PTraversal] to monomorphic updates.
*/
public typealias Traversal = PTraversal
/**
* A [Traversal] is an optic that allows to see into a structure with 0 to N foci.
*
* [Traversal] is a generalisation of [kotlin.collections.map] and can be seen as a representation of modify.
* all methods are written in terms of modify
*
* @param S the source of a [PTraversal]
* @param T the modified source of a [PTraversal]
* @param A the target of a [PTraversal]
* @param B the modified target of a [PTraversal]
*/
public fun interface PTraversal : PSetter {
override fun modify(source: S, map: (focus: A) -> B): T
public fun choice(other: PTraversal): PTraversal, Either, A, B> =
PTraversal { s, f ->
s.fold(
{ a -> Either.Left(this.modify(a, f)) },
{ u -> Either.Right(other.modify(u, f)) }
)
}
/**
* Compose a [PTraversal] with a [PTraversal]
*/
public infix fun compose(other: PTraversal): PTraversal =
PTraversal { s, f -> this.modify(s) { b -> other.modify(b, f) } }
public operator fun plus(other: PTraversal): PTraversal =
this compose other
public companion object {
public fun id(): PTraversal =
PIso.id()
public fun codiagonal(): Traversal, S> =
Traversal { s, f -> s.mapLeft(f).map(f) }
/**
* [PTraversal] that points to nothing
*/
public fun void(): Traversal =
POptional.void()
/**
* [PTraversal] constructor from multiple getters of the same source.
*/
public operator fun invoke(get1: (S) -> A, get2: (S) -> A, set: (B, B, S) -> T): PTraversal =
PTraversal { s, f -> set(f(get1(s)), f(get2(s)), s) }
public operator fun invoke(
get1: (S) -> A,
get2: (S) -> A,
get3: (S) -> A,
set: (B, B, B, S) -> T
): PTraversal =
PTraversal { s, f -> set(f(get1(s)), f(get2(s)), f(get3(s)), s) }
public operator fun invoke(
get1: (S) -> A,
get2: (S) -> A,
get3: (S) -> A,
get4: (S) -> A,
set: (B, B, B, B, S) -> T
): PTraversal =
PTraversal { s, f -> set(f(get1(s)), f(get2(s)), f(get3(s)), f(get4(s)), s) }
public operator fun invoke(
get1: (S) -> A,
get2: (S) -> A,
get3: (S) -> A,
get4: (S) -> A,
get5: (S) -> A,
set: (B, B, B, B, B, S) -> T
): PTraversal =
PTraversal { s, f -> set(f(get1(s)), f(get2(s)), f(get3(s)), f(get4(s)), f(get5(s)), s) }
public operator fun invoke(
get1: (S) -> A,
get2: (S) -> A,
get3: (S) -> A,
get4: (S) -> A,
get5: (S) -> A,
get6: (S) -> A,
set: (B, B, B, B, B, B, S) -> T
): PTraversal =
PTraversal { s, f -> set(f(get1(s)), f(get2(s)), f(get3(s)), f(get4(s)), f(get5(s)), f(get6(s)), s) }
public operator fun invoke(
get1: (S) -> A,
get2: (S) -> A,
get3: (S) -> A,
get4: (S) -> A,
get5: (S) -> A,
get6: (S) -> A,
get7: (S) -> A,
set: (B, B, B, B, B, B, B, S) -> T
): PTraversal =
PTraversal { s, f -> set(f(get1(s)), f(get2(s)), f(get3(s)), f(get4(s)), f(get5(s)), f(get6(s)), f(get7(s)), s) }
public operator fun invoke(
get1: (S) -> A,
get2: (S) -> A,
get3: (S) -> A,
get4: (S) -> A,
get5: (S) -> A,
get6: (S) -> A,
get7: (S) -> A,
get8: (S) -> A,
set: (B, B, B, B, B, B, B, B, S) -> T
): PTraversal =
PTraversal { s, f ->
set(
f(get1(s)),
f(get2(s)),
f(get3(s)),
f(get4(s)),
f(get5(s)),
f(get6(s)),
f(get7(s)),
f(get8(s)),
s
)
}
public operator fun invoke(
get1: (S) -> A,
get2: (S) -> A,
get3: (S) -> A,
get4: (S) -> A,
get5: (S) -> A,
get6: (S) -> A,
get7: (S) -> A,
get8: (S) -> A,
get9: (S) -> A,
set: (B, B, B, B, B, B, B, B, B, S) -> T
): PTraversal =
PTraversal { s, f ->
set(
f(get1(s)),
f(get2(s)),
f(get3(s)),
f(get4(s)),
f(get5(s)),
f(get6(s)),
f(get7(s)),
f(get8(s)),
f(get9(s)),
s
)
}
public operator fun invoke(
get1: (S) -> A,
get2: (S) -> A,
get3: (S) -> A,
get4: (S) -> A,
get5: (S) -> A,
get6: (S) -> A,
get7: (S) -> A,
get8: (S) -> A,
get9: (S) -> A,
get10: (S) -> A,
set: (B, B, B, B, B, B, B, B, B, B, S) -> T
): PTraversal =
PTraversal { s, f ->
set(
f(get1(s)),
f(get2(s)),
f(get3(s)),
f(get4(s)),
f(get5(s)),
f(get6(s)),
f(get7(s)),
f(get8(s)),
f(get9(s)),
f(get10(s)),
s
)
}
/**
* [Traversal] for [List] that focuses in each [A] of the source [List].
*/
@JvmStatic
public fun list(): Traversal, A> =
Every.list()
/**
* [Traversal] for [Either] that has focus in each [Either.Right].
*
* @return [Traversal] with source [Either] and focus every [Either.Right] of the source.
*/
@JvmStatic
public fun either(): Traversal, R> =
Every.either()
@JvmStatic
public fun map(): Traversal
© 2015 - 2025 Weber Informatics LLC | Privacy Policy