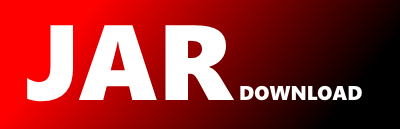
commonMain.arrow.optics.Copy.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arrow-optics-jvm Show documentation
Show all versions of arrow-optics-jvm Show documentation
Functional companion to Kotlin's Standard Library
package arrow.optics
import kotlin.experimental.ExperimentalTypeInference
@DslMarker
public annotation class OpticsCopyMarker
@OpticsCopyMarker
public interface Copy {
/**
* Changes the value of the element(s) pointed by the [Setter].
*/
public infix fun Setter.set(b: B)
/**
* Transforms the value of the element(s) pointed by the [Traversal].
*/
public infix fun Traversal.transform(f: (B) -> B)
/**
* Declares a block in which all optics are nested within
* the given [field]. Instead of:
*
* ```
* x.copy {
* X.a.this set "A"
* X.a.that set "B"
* }
* ```
*
* you can write:
*
* ```
* x.copy {
* inside(X.a) {
* A.this set "A"
* A.that set "B"
* }
* }
* ```
*/
@OptIn(ExperimentalTypeInference::class)
public fun inside(field: Traversal, @BuilderInference f: Copy.() -> Unit): Unit =
field.transform { it.copy(f) }
}
// mutable builder of copies
private class CopyImpl(var current: A): Copy {
override fun Setter.set(b: B) {
current = this.set(current, b)
}
override fun Traversal.transform(f: (B) -> B) {
current = this.modify(current, f)
}
}
/**
* Small DSL which parallel Kotlin's built-in `copy`,
* but using optics instead of field names. See [Copy]
* for the operations allowed inside the block.
*
* This allows declaring changes on nested elements,
* preventing the "nested `copy` problem". Instead of:
*
* ```
* person.copy(address = person.address.copy(city = "Madrid"))
* ```
*
* you can write:
*
* ```
* person.copy {
* Person.address.city set "Madrid"
* }
* ```
*/
@OptIn(ExperimentalTypeInference::class)
public fun A.copy(@BuilderInference f: Copy.() -> Unit): A =
CopyImpl(this).also(f).current
© 2015 - 2025 Weber Informatics LLC | Privacy Policy