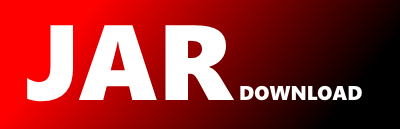
commonMain.arrow.optics.Every.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arrow-optics-jvm Show documentation
Show all versions of arrow-optics-jvm Show documentation
Functional companion to Kotlin's Standard Library
package arrow.optics
import arrow.core.Either
import arrow.core.NonEmptyList
import arrow.core.Option
import arrow.core.Tuple10
import arrow.core.Tuple4
import arrow.core.Tuple5
import arrow.core.Tuple6
import arrow.core.Tuple7
import arrow.core.Tuple8
import arrow.core.Tuple9
import arrow.core.fold
import arrow.core.foldMap
import arrow.typeclasses.Monoid
import kotlin.jvm.JvmStatic
public typealias Every = PEvery
/**
* Composition of Fold and Traversal
* It combines their powers
*/
public interface PEvery : PTraversal, Fold, PSetter {
/**
* Map each target to a type R and use a Monoid to fold the results
*/
override fun foldMap(M: Monoid, source: S, map: (focus: A) -> R): R
override fun modify(source: S, map: (focus: A) -> B): T
/**
* Compose a [PEvery] with a [PEvery]
*/
public infix fun compose(other: PEvery): PEvery =
object : PEvery {
override fun foldMap(M: Monoid, source: S, map: (C) -> R): R =
[email protected](M, source) { c -> other.foldMap(M, c, map) }
override fun modify(source: S, map: (focus: C) -> D): T =
[email protected](source) { b -> other.modify(b, map) }
}
public operator fun plus(other: PEvery): PEvery =
this compose other
public companion object {
public fun from(T: Traversal, F: Fold): Every =
object : Every {
override fun foldMap(M: Monoid, source: S, map: (A) -> R): R = F.foldMap(M, source, map)
override fun modify(source: S, map: (focus: A) -> A): S = T.modify(source, map)
}
/**
* [Traversal] for [List] that focuses in each [A] of the source [List].
*/
@JvmStatic
public fun list(): Every, A> =
object : Every, A> {
override fun modify(source: List, map: (focus: A) -> A): List =
source.map(map)
override fun foldMap(M: Monoid, source: List, map: (focus: A) -> R): R =
source.foldMap(M, map)
}
/**
* [Traversal] for [Either] that has focus in each [Either.Right].
*
* @return [Traversal] with source [Either] and focus every [Either.Right] of the source.
*/
@JvmStatic
public fun either(): Every, R> =
object : Every, R> {
override fun modify(source: Either, map: (focus: R) -> R): Either =
source.map(map)
override fun foldMap(M: Monoid, source: Either, map: (focus: R) -> A): A =
source.fold({ M.empty() }, map)
}
@JvmStatic
public fun map(): Every
© 2015 - 2025 Weber Informatics LLC | Privacy Policy