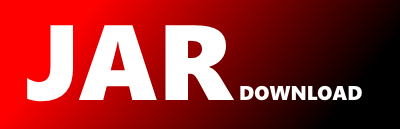
commonMain.arrow.optics.Prism.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arrow-optics-jvm Show documentation
Show all versions of arrow-optics-jvm Show documentation
Functional companion to Kotlin's Standard Library
package arrow.optics
import arrow.core.Either
import arrow.core.None
import arrow.core.Option
import arrow.core.Some
import arrow.core.compose
import arrow.core.flatMap
import arrow.core.identity
import arrow.core.left
import arrow.core.right
import arrow.typeclasses.Monoid
import kotlin.jvm.JvmName
import kotlin.jvm.JvmStatic
/**
* [Prism] is a type alias for [PPrism] which fixes the type arguments
* and restricts the [PPrism] to monomorphic updates.
*/
public typealias Prism = PPrism
/**
* A [Prism] is a loss less invertible optic that can look into a structure and optionally find its focus.
* Mostly used for finding a focus that is only present under certain conditions i.e. list head Prism, Int>
*
* A (polymorphic) [PPrism] is useful when setting or modifying a value for a polymorphic sum type
* i.e. PPrism
© 2015 - 2025 Weber Informatics LLC | Privacy Policy