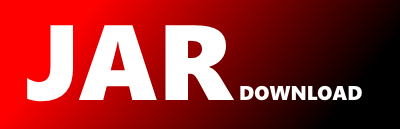
io.atlasmap.v2.Actions Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2018.05.02 at 12:29:58 PM EDT
//
package io.atlasmap.v2;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElements;
import javax.xml.bind.annotation.XmlType;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
/**
* Java class for Actions complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Actions">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <choice maxOccurs="unbounded" minOccurs="0">
* <element ref="{http://atlasmap.io/v2}AbsoluteValue"/>
* <element ref="{http://atlasmap.io/v2}Add"/>
* <element ref="{http://atlasmap.io/v2}AddDays"/>
* <element ref="{http://atlasmap.io/v2}AddSeconds"/>
* <element ref="{http://atlasmap.io/v2}Append"/>
* <element ref="{http://atlasmap.io/v2}Average"/>
* <element ref="{http://atlasmap.io/v2}Camelize"/>
* <element ref="{http://atlasmap.io/v2}Capitalize"/>
* <element ref="{http://atlasmap.io/v2}Ceiling"/>
* <element ref="{http://atlasmap.io/v2}Concatenate"/>
* <element ref="{http://atlasmap.io/v2}Contains"/>
* <element ref="{http://atlasmap.io/v2}ConvertAreaUnit"/>
* <element ref="{http://atlasmap.io/v2}ConvertDistanceUnit"/>
* <element ref="{http://atlasmap.io/v2}ConvertMassUnit"/>
* <element ref="{http://atlasmap.io/v2}ConvertVolumeUnit"/>
* <element ref="{http://atlasmap.io/v2}CurrentDate"/>
* <element ref="{http://atlasmap.io/v2}CurrentDateTime"/>
* <element ref="{http://atlasmap.io/v2}CurrentTime"/>
* <element ref="{http://atlasmap.io/v2}DayOfWeek"/>
* <element ref="{http://atlasmap.io/v2}DayOfYear"/>
* <element ref="{http://atlasmap.io/v2}Divide"/>
* <element ref="{http://atlasmap.io/v2}EndsWith"/>
* <element ref="{http://atlasmap.io/v2}Equals"/>
* <element ref="{http://atlasmap.io/v2}FileExtension"/>
* <element ref="{http://atlasmap.io/v2}Floor"/>
* <element ref="{http://atlasmap.io/v2}Format"/>
* <element ref="{http://atlasmap.io/v2}GenerateUUID"/>
* <element ref="{http://atlasmap.io/v2}IndexOf"/>
* <element ref="{http://atlasmap.io/v2}IsNull"/>
* <element ref="{http://atlasmap.io/v2}LastIndexOf"/>
* <element ref="{http://atlasmap.io/v2}Length"/>
* <element ref="{http://atlasmap.io/v2}LowercaseChar"/>
* <element ref="{http://atlasmap.io/v2}Lowercase"/>
* <element ref="{http://atlasmap.io/v2}Maximum"/>
* <element ref="{http://atlasmap.io/v2}Minimum"/>
* <element ref="{http://atlasmap.io/v2}Multiply"/>
* <element ref="{http://atlasmap.io/v2}Normalize"/>
* <element ref="{http://atlasmap.io/v2}PadStringLeft"/>
* <element ref="{http://atlasmap.io/v2}PadStringRight"/>
* <element ref="{http://atlasmap.io/v2}Prepend"/>
* <element ref="{http://atlasmap.io/v2}RemoveFileExtension"/>
* <element ref="{http://atlasmap.io/v2}ReplaceAll"/>
* <element ref="{http://atlasmap.io/v2}ReplaceFirst"/>
* <element ref="{http://atlasmap.io/v2}Round"/>
* <element ref="{http://atlasmap.io/v2}SeparateByDash"/>
* <element ref="{http://atlasmap.io/v2}SeparateByUnderscore"/>
* <element ref="{http://atlasmap.io/v2}StartsWith"/>
* <element ref="{http://atlasmap.io/v2}SubString"/>
* <element ref="{http://atlasmap.io/v2}SubStringAfter"/>
* <element ref="{http://atlasmap.io/v2}SubStringBefore"/>
* <element ref="{http://atlasmap.io/v2}Subtract"/>
* <element ref="{http://atlasmap.io/v2}Trim"/>
* <element ref="{http://atlasmap.io/v2}TrimLeft"/>
* <element ref="{http://atlasmap.io/v2}TrimRight"/>
* <element ref="{http://atlasmap.io/v2}Uppercase"/>
* <element ref="{http://atlasmap.io/v2}UppercaseChar"/>
* </choice>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Actions", propOrder = {
"actions"
})
@JsonSerialize(using = ActionsJsonSerializer.class)
@JsonDeserialize(using = ActionsJsonDeserializer.class)
public class Actions
implements Serializable
{
private final static long serialVersionUID = 1L;
@XmlElements({
@XmlElement(name = "AbsoluteValue", type = AbsoluteValue.class),
@XmlElement(name = "Add", type = Add.class),
@XmlElement(name = "AddDays", type = AddDays.class),
@XmlElement(name = "AddSeconds", type = AddSeconds.class),
@XmlElement(name = "Append", type = Append.class),
@XmlElement(name = "Average", type = Average.class),
@XmlElement(name = "Camelize", type = Camelize.class),
@XmlElement(name = "Capitalize", type = Capitalize.class),
@XmlElement(name = "Ceiling", type = Ceiling.class),
@XmlElement(name = "Concatenate", type = Concatenate.class),
@XmlElement(name = "Contains", type = Contains.class),
@XmlElement(name = "ConvertAreaUnit", type = ConvertAreaUnit.class),
@XmlElement(name = "ConvertDistanceUnit", type = ConvertDistanceUnit.class),
@XmlElement(name = "ConvertMassUnit", type = ConvertMassUnit.class),
@XmlElement(name = "ConvertVolumeUnit", type = ConvertVolumeUnit.class),
@XmlElement(name = "CurrentDate", type = CurrentDate.class),
@XmlElement(name = "CurrentDateTime", type = CurrentDateTime.class),
@XmlElement(name = "CurrentTime", type = CurrentTime.class),
@XmlElement(name = "DayOfWeek", type = DayOfWeek.class),
@XmlElement(name = "DayOfYear", type = DayOfYear.class),
@XmlElement(name = "Divide", type = Divide.class),
@XmlElement(name = "EndsWith", type = EndsWith.class),
@XmlElement(name = "Equals", type = Equals.class),
@XmlElement(name = "FileExtension", type = FileExtension.class),
@XmlElement(name = "Floor", type = Floor.class),
@XmlElement(name = "Format", type = Format.class),
@XmlElement(name = "GenerateUUID", type = GenerateUUID.class),
@XmlElement(name = "IndexOf", type = IndexOf.class),
@XmlElement(name = "IsNull", type = IsNull.class),
@XmlElement(name = "LastIndexOf", type = LastIndexOf.class),
@XmlElement(name = "Length", type = Length.class),
@XmlElement(name = "LowercaseChar", type = LowercaseChar.class),
@XmlElement(name = "Lowercase", type = Lowercase.class),
@XmlElement(name = "Maximum", type = Maximum.class),
@XmlElement(name = "Minimum", type = Minimum.class),
@XmlElement(name = "Multiply", type = Multiply.class),
@XmlElement(name = "Normalize", type = Normalize.class),
@XmlElement(name = "PadStringLeft", type = PadStringLeft.class),
@XmlElement(name = "PadStringRight", type = PadStringRight.class),
@XmlElement(name = "Prepend", type = Prepend.class),
@XmlElement(name = "RemoveFileExtension", type = RemoveFileExtension.class),
@XmlElement(name = "ReplaceAll", type = ReplaceAll.class),
@XmlElement(name = "ReplaceFirst", type = ReplaceFirst.class),
@XmlElement(name = "Round", type = Round.class),
@XmlElement(name = "SeparateByDash", type = SeparateByDash.class),
@XmlElement(name = "SeparateByUnderscore", type = SeparateByUnderscore.class),
@XmlElement(name = "StartsWith", type = StartsWith.class),
@XmlElement(name = "SubString", type = SubString.class),
@XmlElement(name = "SubStringAfter", type = SubStringAfter.class),
@XmlElement(name = "SubStringBefore", type = SubStringBefore.class),
@XmlElement(name = "Subtract", type = Subtract.class),
@XmlElement(name = "Trim", type = Trim.class),
@XmlElement(name = "TrimLeft", type = TrimLeft.class),
@XmlElement(name = "TrimRight", type = TrimRight.class),
@XmlElement(name = "Uppercase", type = Uppercase.class),
@XmlElement(name = "UppercaseChar", type = UppercaseChar.class)
})
protected List actions;
/**
* Gets the value of the actions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the actions property.
*
*
* For example, to add a new item, do as follows:
*
* getActions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AbsoluteValue }
* {@link Add }
* {@link AddDays }
* {@link AddSeconds }
* {@link Append }
* {@link Average }
* {@link Camelize }
* {@link Capitalize }
* {@link Ceiling }
* {@link Concatenate }
* {@link Contains }
* {@link ConvertAreaUnit }
* {@link ConvertDistanceUnit }
* {@link ConvertMassUnit }
* {@link ConvertVolumeUnit }
* {@link CurrentDate }
* {@link CurrentDateTime }
* {@link CurrentTime }
* {@link DayOfWeek }
* {@link DayOfYear }
* {@link Divide }
* {@link EndsWith }
* {@link Equals }
* {@link FileExtension }
* {@link Floor }
* {@link Format }
* {@link GenerateUUID }
* {@link IndexOf }
* {@link IsNull }
* {@link LastIndexOf }
* {@link Length }
* {@link LowercaseChar }
* {@link Lowercase }
* {@link Maximum }
* {@link Minimum }
* {@link Multiply }
* {@link Normalize }
* {@link PadStringLeft }
* {@link PadStringRight }
* {@link Prepend }
* {@link RemoveFileExtension }
* {@link ReplaceAll }
* {@link ReplaceFirst }
* {@link Round }
* {@link SeparateByDash }
* {@link SeparateByUnderscore }
* {@link StartsWith }
* {@link SubString }
* {@link SubStringAfter }
* {@link SubStringBefore }
* {@link Subtract }
* {@link Trim }
* {@link TrimLeft }
* {@link TrimRight }
* {@link Uppercase }
* {@link UppercaseChar }
*
*
*/
public List getActions() {
if (actions == null) {
actions = new ArrayList();
}
return this.actions;
}
}