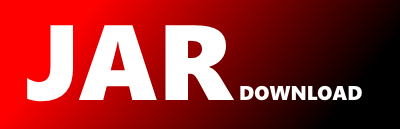
io.atlasmap.v2.ObjectFactory Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2018.05.02 at 12:29:58 PM EDT
//
package io.atlasmap.v2;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the io.atlasmap.v2 package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _AtlasMapping_QNAME = new QName("http://atlasmap.io/v2", "AtlasMapping");
private final static QName _Document_QNAME = new QName("http://atlasmap.io/v2", "Document");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: io.atlasmap.v2
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link AbsoluteValue }
*
*/
public AbsoluteValue createAbsoluteValue() {
return new AbsoluteValue();
}
/**
* Create an instance of {@link Add }
*
*/
public Add createAdd() {
return new Add();
}
/**
* Create an instance of {@link AddDays }
*
*/
public AddDays createAddDays() {
return new AddDays();
}
/**
* Create an instance of {@link AddSeconds }
*
*/
public AddSeconds createAddSeconds() {
return new AddSeconds();
}
/**
* Create an instance of {@link Append }
*
*/
public Append createAppend() {
return new Append();
}
/**
* Create an instance of {@link Average }
*
*/
public Average createAverage() {
return new Average();
}
/**
* Create an instance of {@link Camelize }
*
*/
public Camelize createCamelize() {
return new Camelize();
}
/**
* Create an instance of {@link Capitalize }
*
*/
public Capitalize createCapitalize() {
return new Capitalize();
}
/**
* Create an instance of {@link Ceiling }
*
*/
public Ceiling createCeiling() {
return new Ceiling();
}
/**
* Create an instance of {@link Concatenate }
*
*/
public Concatenate createConcatenate() {
return new Concatenate();
}
/**
* Create an instance of {@link Contains }
*
*/
public Contains createContains() {
return new Contains();
}
/**
* Create an instance of {@link ConvertAreaUnit }
*
*/
public ConvertAreaUnit createConvertAreaUnit() {
return new ConvertAreaUnit();
}
/**
* Create an instance of {@link ConvertDistanceUnit }
*
*/
public ConvertDistanceUnit createConvertDistanceUnit() {
return new ConvertDistanceUnit();
}
/**
* Create an instance of {@link ConvertMassUnit }
*
*/
public ConvertMassUnit createConvertMassUnit() {
return new ConvertMassUnit();
}
/**
* Create an instance of {@link ConvertVolumeUnit }
*
*/
public ConvertVolumeUnit createConvertVolumeUnit() {
return new ConvertVolumeUnit();
}
/**
* Create an instance of {@link CustomAction }
*
*/
public CustomAction createCustomAction() {
return new CustomAction();
}
/**
* Create an instance of {@link CurrentDate }
*
*/
public CurrentDate createCurrentDate() {
return new CurrentDate();
}
/**
* Create an instance of {@link CurrentDateTime }
*
*/
public CurrentDateTime createCurrentDateTime() {
return new CurrentDateTime();
}
/**
* Create an instance of {@link CurrentTime }
*
*/
public CurrentTime createCurrentTime() {
return new CurrentTime();
}
/**
* Create an instance of {@link DayOfWeek }
*
*/
public DayOfWeek createDayOfWeek() {
return new DayOfWeek();
}
/**
* Create an instance of {@link DayOfYear }
*
*/
public DayOfYear createDayOfYear() {
return new DayOfYear();
}
/**
* Create an instance of {@link Divide }
*
*/
public Divide createDivide() {
return new Divide();
}
/**
* Create an instance of {@link EndsWith }
*
*/
public EndsWith createEndsWith() {
return new EndsWith();
}
/**
* Create an instance of {@link Equals }
*
*/
public Equals createEquals() {
return new Equals();
}
/**
* Create an instance of {@link FileExtension }
*
*/
public FileExtension createFileExtension() {
return new FileExtension();
}
/**
* Create an instance of {@link Floor }
*
*/
public Floor createFloor() {
return new Floor();
}
/**
* Create an instance of {@link Format }
*
*/
public Format createFormat() {
return new Format();
}
/**
* Create an instance of {@link GenerateUUID }
*
*/
public GenerateUUID createGenerateUUID() {
return new GenerateUUID();
}
/**
* Create an instance of {@link IndexOf }
*
*/
public IndexOf createIndexOf() {
return new IndexOf();
}
/**
* Create an instance of {@link IsNull }
*
*/
public IsNull createIsNull() {
return new IsNull();
}
/**
* Create an instance of {@link LastIndexOf }
*
*/
public LastIndexOf createLastIndexOf() {
return new LastIndexOf();
}
/**
* Create an instance of {@link Length }
*
*/
public Length createLength() {
return new Length();
}
/**
* Create an instance of {@link Lowercase }
*
*/
public Lowercase createLowercase() {
return new Lowercase();
}
/**
* Create an instance of {@link LowercaseChar }
*
*/
public LowercaseChar createLowercaseChar() {
return new LowercaseChar();
}
/**
* Create an instance of {@link Maximum }
*
*/
public Maximum createMaximum() {
return new Maximum();
}
/**
* Create an instance of {@link Minimum }
*
*/
public Minimum createMinimum() {
return new Minimum();
}
/**
* Create an instance of {@link Multiply }
*
*/
public Multiply createMultiply() {
return new Multiply();
}
/**
* Create an instance of {@link Normalize }
*
*/
public Normalize createNormalize() {
return new Normalize();
}
/**
* Create an instance of {@link PadStringLeft }
*
*/
public PadStringLeft createPadStringLeft() {
return new PadStringLeft();
}
/**
* Create an instance of {@link PadStringRight }
*
*/
public PadStringRight createPadStringRight() {
return new PadStringRight();
}
/**
* Create an instance of {@link Prepend }
*
*/
public Prepend createPrepend() {
return new Prepend();
}
/**
* Create an instance of {@link RemoveFileExtension }
*
*/
public RemoveFileExtension createRemoveFileExtension() {
return new RemoveFileExtension();
}
/**
* Create an instance of {@link ReplaceAll }
*
*/
public ReplaceAll createReplaceAll() {
return new ReplaceAll();
}
/**
* Create an instance of {@link ReplaceFirst }
*
*/
public ReplaceFirst createReplaceFirst() {
return new ReplaceFirst();
}
/**
* Create an instance of {@link Round }
*
*/
public Round createRound() {
return new Round();
}
/**
* Create an instance of {@link SeparateByDash }
*
*/
public SeparateByDash createSeparateByDash() {
return new SeparateByDash();
}
/**
* Create an instance of {@link SeparateByUnderscore }
*
*/
public SeparateByUnderscore createSeparateByUnderscore() {
return new SeparateByUnderscore();
}
/**
* Create an instance of {@link StartsWith }
*
*/
public StartsWith createStartsWith() {
return new StartsWith();
}
/**
* Create an instance of {@link SubString }
*
*/
public SubString createSubString() {
return new SubString();
}
/**
* Create an instance of {@link SubStringAfter }
*
*/
public SubStringAfter createSubStringAfter() {
return new SubStringAfter();
}
/**
* Create an instance of {@link SubStringBefore }
*
*/
public SubStringBefore createSubStringBefore() {
return new SubStringBefore();
}
/**
* Create an instance of {@link Subtract }
*
*/
public Subtract createSubtract() {
return new Subtract();
}
/**
* Create an instance of {@link Trim }
*
*/
public Trim createTrim() {
return new Trim();
}
/**
* Create an instance of {@link TrimLeft }
*
*/
public TrimLeft createTrimLeft() {
return new TrimLeft();
}
/**
* Create an instance of {@link TrimRight }
*
*/
public TrimRight createTrimRight() {
return new TrimRight();
}
/**
* Create an instance of {@link Uppercase }
*
*/
public Uppercase createUppercase() {
return new Uppercase();
}
/**
* Create an instance of {@link UppercaseChar }
*
*/
public UppercaseChar createUppercaseChar() {
return new UppercaseChar();
}
/**
* Create an instance of {@link AtlasMapping }
*
*/
public AtlasMapping createAtlasMapping() {
return new AtlasMapping();
}
/**
* Create an instance of {@link Actions }
*
*/
public Actions createActions() {
return new Actions();
}
/**
* Create an instance of {@link DataSource }
*
*/
public DataSource createDataSource() {
return new DataSource();
}
/**
* Create an instance of {@link Constants }
*
*/
public Constants createConstants() {
return new Constants();
}
/**
* Create an instance of {@link Constant }
*
*/
public Constant createConstant() {
return new Constant();
}
/**
* Create an instance of {@link Properties }
*
*/
public Properties createProperties() {
return new Properties();
}
/**
* Create an instance of {@link Property }
*
*/
public Property createProperty() {
return new Property();
}
/**
* Create an instance of {@link Mappings }
*
*/
public Mappings createMappings() {
return new Mappings();
}
/**
* Create an instance of {@link Mapping }
*
*/
public Mapping createMapping() {
return new Mapping();
}
/**
* Create an instance of {@link Collection }
*
*/
public Collection createCollection() {
return new Collection();
}
/**
* Create an instance of {@link Fields }
*
*/
public Fields createFields() {
return new Fields();
}
/**
* Create an instance of {@link SimpleField }
*
*/
public SimpleField createSimpleField() {
return new SimpleField();
}
/**
* Create an instance of {@link PropertyField }
*
*/
public PropertyField createPropertyField() {
return new PropertyField();
}
/**
* Create an instance of {@link ConstantField }
*
*/
public ConstantField createConstantField() {
return new ConstantField();
}
/**
* Create an instance of {@link MockDocument }
*
*/
public MockDocument createMockDocument() {
return new MockDocument();
}
/**
* Create an instance of {@link MockField }
*
*/
public MockField createMockField() {
return new MockField();
}
/**
* Create an instance of {@link Validations }
*
*/
public Validations createValidations() {
return new Validations();
}
/**
* Create an instance of {@link Validation }
*
*/
public Validation createValidation() {
return new Validation();
}
/**
* Create an instance of {@link Audits }
*
*/
public Audits createAudits() {
return new Audits();
}
/**
* Create an instance of {@link Audit }
*
*/
public Audit createAudit() {
return new Audit();
}
/**
* Create an instance of {@link StringList }
*
*/
public StringList createStringList() {
return new StringList();
}
/**
* Create an instance of {@link StringMap }
*
*/
public StringMap createStringMap() {
return new StringMap();
}
/**
* Create an instance of {@link StringMapEntry }
*
*/
public StringMapEntry createStringMapEntry() {
return new StringMapEntry();
}
/**
* Create an instance of {@link LookupEntry }
*
*/
public LookupEntry createLookupEntry() {
return new LookupEntry();
}
/**
* Create an instance of {@link LookupTables }
*
*/
public LookupTables createLookupTables() {
return new LookupTables();
}
/**
* Create an instance of {@link LookupTable }
*
*/
public LookupTable createLookupTable() {
return new LookupTable();
}
/**
* Create an instance of {@link ActionDetail }
*
*/
public ActionDetail createActionDetail() {
return new ActionDetail();
}
/**
* Create an instance of {@link ActionParameters }
*
*/
public ActionParameters createActionParameters() {
return new ActionParameters();
}
/**
* Create an instance of {@link ActionParameter }
*
*/
public ActionParameter createActionParameter() {
return new ActionParameter();
}
/**
* Create an instance of {@link ActionDetails }
*
*/
public ActionDetails createActionDetails() {
return new ActionDetails();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AtlasMapping }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://atlasmap.io/v2", name = "AtlasMapping")
public JAXBElement createAtlasMapping(AtlasMapping value) {
return new JAXBElement(_AtlasMapping_QNAME, AtlasMapping.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Document }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://atlasmap.io/v2", name = "Document")
public JAXBElement createDocument(Document value) {
return new JAXBElement(_Document_QNAME, Document.class, null, value);
}
}