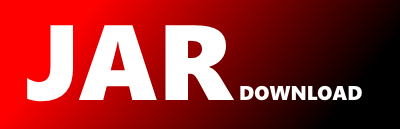
io.atlasmap.xml.test.v2.ObjectFactory Maven / Gradle / Ivy
package io.atlasmap.xml.test.v2;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the io.atlasmap.xml.test.v2 package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _XmlFPA_QNAME = new QName("http://atlasmap.io/xml/test/v2", "XmlFPA");
private final static QName _XmlFBPA_QNAME = new QName("http://atlasmap.io/xml/test/v2", "XmlFBPA");
private final static QName _XmlFPE_QNAME = new QName("http://atlasmap.io/xml/test/v2", "XmlFPE");
private final static QName _XmlFBPE_QNAME = new QName("http://atlasmap.io/xml/test/v2", "XmlFBPE");
private final static QName _XmlCA_QNAME = new QName("http://atlasmap.io/xml/test/v2", "XmlCA");
private final static QName _XmlCE_QNAME = new QName("http://atlasmap.io/xml/test/v2", "XmlCE");
private final static QName _XmlAA_QNAME = new QName("http://atlasmap.io/xml/test/v2", "XmlAA");
private final static QName _XmlAE_QNAME = new QName("http://atlasmap.io/xml/test/v2", "XmlAE");
private final static QName _XmlOA_QNAME = new QName("http://atlasmap.io/xml/test/v2", "XmlOA");
private final static QName _XmlOE_QNAME = new QName("http://atlasmap.io/xml/test/v2", "XmlOE");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: io.atlasmap.xml.test.v2
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link XmlFlatPrimitiveAttribute }
*
*/
public XmlFlatPrimitiveAttribute createXmlFlatPrimitiveAttribute() {
return new XmlFlatPrimitiveAttribute();
}
/**
* Create an instance of {@link XmlFlatBoxedPrimitiveAttribute }
*
*/
public XmlFlatBoxedPrimitiveAttribute createXmlFlatBoxedPrimitiveAttribute() {
return new XmlFlatBoxedPrimitiveAttribute();
}
/**
* Create an instance of {@link XmlFlatPrimitiveElement }
*
*/
public XmlFlatPrimitiveElement createXmlFlatPrimitiveElement() {
return new XmlFlatPrimitiveElement();
}
/**
* Create an instance of {@link XmlFlatBoxedPrimitiveElement }
*
*/
public XmlFlatBoxedPrimitiveElement createXmlFlatBoxedPrimitiveElement() {
return new XmlFlatBoxedPrimitiveElement();
}
/**
* Create an instance of {@link XmlContactAttribute }
*
*/
public XmlContactAttribute createXmlContactAttribute() {
return new XmlContactAttribute();
}
/**
* Create an instance of {@link XmlContactElement }
*
*/
public XmlContactElement createXmlContactElement() {
return new XmlContactElement();
}
/**
* Create an instance of {@link XmlAddressAttribute }
*
*/
public XmlAddressAttribute createXmlAddressAttribute() {
return new XmlAddressAttribute();
}
/**
* Create an instance of {@link XmlAddressElement }
*
*/
public XmlAddressElement createXmlAddressElement() {
return new XmlAddressElement();
}
/**
* Create an instance of {@link XmlOrderAttribute }
*
*/
public XmlOrderAttribute createXmlOrderAttribute() {
return new XmlOrderAttribute();
}
/**
* Create an instance of {@link XmlOrderElement }
*
*/
public XmlOrderElement createXmlOrderElement() {
return new XmlOrderElement();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link XmlFlatPrimitiveAttribute }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://atlasmap.io/xml/test/v2", name = "XmlFPA")
public JAXBElement createXmlFPA(XmlFlatPrimitiveAttribute value) {
return new JAXBElement(_XmlFPA_QNAME, XmlFlatPrimitiveAttribute.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link XmlFlatBoxedPrimitiveAttribute }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://atlasmap.io/xml/test/v2", name = "XmlFBPA")
public JAXBElement createXmlFBPA(XmlFlatBoxedPrimitiveAttribute value) {
return new JAXBElement(_XmlFBPA_QNAME, XmlFlatBoxedPrimitiveAttribute.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link XmlFlatPrimitiveElement }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://atlasmap.io/xml/test/v2", name = "XmlFPE")
public JAXBElement createXmlFPE(XmlFlatPrimitiveElement value) {
return new JAXBElement(_XmlFPE_QNAME, XmlFlatPrimitiveElement.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link XmlFlatBoxedPrimitiveElement }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://atlasmap.io/xml/test/v2", name = "XmlFBPE")
public JAXBElement createXmlFBPE(XmlFlatBoxedPrimitiveElement value) {
return new JAXBElement(_XmlFBPE_QNAME, XmlFlatBoxedPrimitiveElement.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link XmlContactAttribute }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://atlasmap.io/xml/test/v2", name = "XmlCA")
public JAXBElement createXmlCA(XmlContactAttribute value) {
return new JAXBElement(_XmlCA_QNAME, XmlContactAttribute.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link XmlContactElement }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://atlasmap.io/xml/test/v2", name = "XmlCE")
public JAXBElement createXmlCE(XmlContactElement value) {
return new JAXBElement(_XmlCE_QNAME, XmlContactElement.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link XmlAddressAttribute }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://atlasmap.io/xml/test/v2", name = "XmlAA")
public JAXBElement createXmlAA(XmlAddressAttribute value) {
return new JAXBElement(_XmlAA_QNAME, XmlAddressAttribute.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link XmlAddressElement }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://atlasmap.io/xml/test/v2", name = "XmlAE")
public JAXBElement createXmlAE(XmlAddressElement value) {
return new JAXBElement(_XmlAE_QNAME, XmlAddressElement.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link XmlOrderAttribute }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://atlasmap.io/xml/test/v2", name = "XmlOA")
public JAXBElement createXmlOA(XmlOrderAttribute value) {
return new JAXBElement(_XmlOA_QNAME, XmlOrderAttribute.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link XmlOrderElement }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://atlasmap.io/xml/test/v2", name = "XmlOE")
public JAXBElement createXmlOE(XmlOrderElement value) {
return new JAXBElement(_XmlOE_QNAME, XmlOrderElement.class, null, value);
}
}