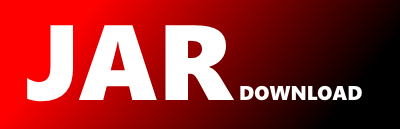
io.atlasmap.xml.test.v2.XmlFlatBoxedPrimitiveElement Maven / Gradle / Ivy
package io.atlasmap.xml.test.v2;
import java.io.Serializable;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for XmlFlatBoxedPrimitiveElement complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="XmlFlatBoxedPrimitiveElement">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="boxedBooleanField" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="boxedByteField" type="{http://www.w3.org/2001/XMLSchema}byte" minOccurs="0"/>
* <element name="boxedCharField" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="boxedDoubleField" type="{http://www.w3.org/2001/XMLSchema}double" minOccurs="0"/>
* <element name="boxedFloatField" type="{http://www.w3.org/2001/XMLSchema}float" minOccurs="0"/>
* <element name="boxedIntField" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="boxedLongField" type="{http://www.w3.org/2001/XMLSchema}long" minOccurs="0"/>
* <element name="boxedShortField" type="{http://www.w3.org/2001/XMLSchema}short" minOccurs="0"/>
* <element name="boxedStringField" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "XmlFlatBoxedPrimitiveElement", propOrder = {
"boxedBooleanField",
"boxedByteField",
"boxedCharField",
"boxedDoubleField",
"boxedFloatField",
"boxedIntField",
"boxedLongField",
"boxedShortField",
"boxedStringField"
})
@XmlRootElement(name = "XmlFlatBoxedPrimitiveElement")
public class XmlFlatBoxedPrimitiveElement
implements Serializable
{
private final static long serialVersionUID = 1L;
protected Boolean boxedBooleanField;
protected Byte boxedByteField;
protected String boxedCharField;
protected Double boxedDoubleField;
protected Float boxedFloatField;
protected Integer boxedIntField;
protected Long boxedLongField;
protected Short boxedShortField;
protected String boxedStringField;
/**
* Gets the value of the boxedBooleanField property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isBoxedBooleanField() {
return boxedBooleanField;
}
/**
* Sets the value of the boxedBooleanField property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setBoxedBooleanField(Boolean value) {
this.boxedBooleanField = value;
}
/**
* Gets the value of the boxedByteField property.
*
* @return
* possible object is
* {@link Byte }
*
*/
public Byte getBoxedByteField() {
return boxedByteField;
}
/**
* Sets the value of the boxedByteField property.
*
* @param value
* allowed object is
* {@link Byte }
*
*/
public void setBoxedByteField(Byte value) {
this.boxedByteField = value;
}
/**
* Gets the value of the boxedCharField property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBoxedCharField() {
return boxedCharField;
}
/**
* Sets the value of the boxedCharField property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBoxedCharField(String value) {
this.boxedCharField = value;
}
/**
* Gets the value of the boxedDoubleField property.
*
* @return
* possible object is
* {@link Double }
*
*/
public Double getBoxedDoubleField() {
return boxedDoubleField;
}
/**
* Sets the value of the boxedDoubleField property.
*
* @param value
* allowed object is
* {@link Double }
*
*/
public void setBoxedDoubleField(Double value) {
this.boxedDoubleField = value;
}
/**
* Gets the value of the boxedFloatField property.
*
* @return
* possible object is
* {@link Float }
*
*/
public Float getBoxedFloatField() {
return boxedFloatField;
}
/**
* Sets the value of the boxedFloatField property.
*
* @param value
* allowed object is
* {@link Float }
*
*/
public void setBoxedFloatField(Float value) {
this.boxedFloatField = value;
}
/**
* Gets the value of the boxedIntField property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getBoxedIntField() {
return boxedIntField;
}
/**
* Sets the value of the boxedIntField property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setBoxedIntField(Integer value) {
this.boxedIntField = value;
}
/**
* Gets the value of the boxedLongField property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getBoxedLongField() {
return boxedLongField;
}
/**
* Sets the value of the boxedLongField property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setBoxedLongField(Long value) {
this.boxedLongField = value;
}
/**
* Gets the value of the boxedShortField property.
*
* @return
* possible object is
* {@link Short }
*
*/
public Short getBoxedShortField() {
return boxedShortField;
}
/**
* Sets the value of the boxedShortField property.
*
* @param value
* allowed object is
* {@link Short }
*
*/
public void setBoxedShortField(Short value) {
this.boxedShortField = value;
}
/**
* Gets the value of the boxedStringField property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBoxedStringField() {
return boxedStringField;
}
/**
* Sets the value of the boxedStringField property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBoxedStringField(String value) {
this.boxedStringField = value;
}
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final XmlFlatBoxedPrimitiveElement that = ((XmlFlatBoxedPrimitiveElement) object);
{
Boolean leftBoxedBooleanField;
leftBoxedBooleanField = this.isBoxedBooleanField();
Boolean rightBoxedBooleanField;
rightBoxedBooleanField = that.isBoxedBooleanField();
if (this.boxedBooleanField!= null) {
if (that.boxedBooleanField!= null) {
if (!leftBoxedBooleanField.equals(rightBoxedBooleanField)) {
return false;
}
} else {
return false;
}
} else {
if (that.boxedBooleanField!= null) {
return false;
}
}
}
{
Byte leftBoxedByteField;
leftBoxedByteField = this.getBoxedByteField();
Byte rightBoxedByteField;
rightBoxedByteField = that.getBoxedByteField();
if (this.boxedByteField!= null) {
if (that.boxedByteField!= null) {
if (!leftBoxedByteField.equals(rightBoxedByteField)) {
return false;
}
} else {
return false;
}
} else {
if (that.boxedByteField!= null) {
return false;
}
}
}
{
String leftBoxedCharField;
leftBoxedCharField = this.getBoxedCharField();
String rightBoxedCharField;
rightBoxedCharField = that.getBoxedCharField();
if (this.boxedCharField!= null) {
if (that.boxedCharField!= null) {
if (!leftBoxedCharField.equals(rightBoxedCharField)) {
return false;
}
} else {
return false;
}
} else {
if (that.boxedCharField!= null) {
return false;
}
}
}
{
Double leftBoxedDoubleField;
leftBoxedDoubleField = this.getBoxedDoubleField();
Double rightBoxedDoubleField;
rightBoxedDoubleField = that.getBoxedDoubleField();
if (this.boxedDoubleField!= null) {
if (that.boxedDoubleField!= null) {
if (!leftBoxedDoubleField.equals(rightBoxedDoubleField)) {
return false;
}
} else {
return false;
}
} else {
if (that.boxedDoubleField!= null) {
return false;
}
}
}
{
Float leftBoxedFloatField;
leftBoxedFloatField = this.getBoxedFloatField();
Float rightBoxedFloatField;
rightBoxedFloatField = that.getBoxedFloatField();
if (this.boxedFloatField!= null) {
if (that.boxedFloatField!= null) {
if (!leftBoxedFloatField.equals(rightBoxedFloatField)) {
return false;
}
} else {
return false;
}
} else {
if (that.boxedFloatField!= null) {
return false;
}
}
}
{
Integer leftBoxedIntField;
leftBoxedIntField = this.getBoxedIntField();
Integer rightBoxedIntField;
rightBoxedIntField = that.getBoxedIntField();
if (this.boxedIntField!= null) {
if (that.boxedIntField!= null) {
if (!leftBoxedIntField.equals(rightBoxedIntField)) {
return false;
}
} else {
return false;
}
} else {
if (that.boxedIntField!= null) {
return false;
}
}
}
{
Long leftBoxedLongField;
leftBoxedLongField = this.getBoxedLongField();
Long rightBoxedLongField;
rightBoxedLongField = that.getBoxedLongField();
if (this.boxedLongField!= null) {
if (that.boxedLongField!= null) {
if (!leftBoxedLongField.equals(rightBoxedLongField)) {
return false;
}
} else {
return false;
}
} else {
if (that.boxedLongField!= null) {
return false;
}
}
}
{
Short leftBoxedShortField;
leftBoxedShortField = this.getBoxedShortField();
Short rightBoxedShortField;
rightBoxedShortField = that.getBoxedShortField();
if (this.boxedShortField!= null) {
if (that.boxedShortField!= null) {
if (!leftBoxedShortField.equals(rightBoxedShortField)) {
return false;
}
} else {
return false;
}
} else {
if (that.boxedShortField!= null) {
return false;
}
}
}
{
String leftBoxedStringField;
leftBoxedStringField = this.getBoxedStringField();
String rightBoxedStringField;
rightBoxedStringField = that.getBoxedStringField();
if (this.boxedStringField!= null) {
if (that.boxedStringField!= null) {
if (!leftBoxedStringField.equals(rightBoxedStringField)) {
return false;
}
} else {
return false;
}
} else {
if (that.boxedStringField!= null) {
return false;
}
}
}
return true;
}
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
Boolean theBoxedBooleanField;
theBoxedBooleanField = this.isBoxedBooleanField();
if (this.boxedBooleanField!= null) {
currentHashCode += theBoxedBooleanField.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Byte theBoxedByteField;
theBoxedByteField = this.getBoxedByteField();
if (this.boxedByteField!= null) {
currentHashCode += theBoxedByteField.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theBoxedCharField;
theBoxedCharField = this.getBoxedCharField();
if (this.boxedCharField!= null) {
currentHashCode += theBoxedCharField.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Double theBoxedDoubleField;
theBoxedDoubleField = this.getBoxedDoubleField();
if (this.boxedDoubleField!= null) {
currentHashCode += theBoxedDoubleField.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Float theBoxedFloatField;
theBoxedFloatField = this.getBoxedFloatField();
if (this.boxedFloatField!= null) {
currentHashCode += theBoxedFloatField.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Integer theBoxedIntField;
theBoxedIntField = this.getBoxedIntField();
if (this.boxedIntField!= null) {
currentHashCode += theBoxedIntField.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Long theBoxedLongField;
theBoxedLongField = this.getBoxedLongField();
if (this.boxedLongField!= null) {
currentHashCode += theBoxedLongField.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Short theBoxedShortField;
theBoxedShortField = this.getBoxedShortField();
if (this.boxedShortField!= null) {
currentHashCode += theBoxedShortField.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theBoxedStringField;
theBoxedStringField = this.getBoxedStringField();
if (this.boxedStringField!= null) {
currentHashCode += theBoxedStringField.hashCode();
}
}
return currentHashCode;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy