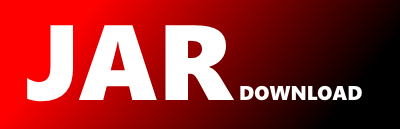
io.atlasmap.xml.test.v2.XmlFlatPrimitiveAttribute Maven / Gradle / Ivy
package io.atlasmap.xml.test.v2;
import java.io.Serializable;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for XmlFlatPrimitiveAttribute complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="XmlFlatPrimitiveAttribute">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="booleanField" use="required" type="{http://www.w3.org/2001/XMLSchema}boolean" />
* <attribute name="byteField" use="required" type="{http://www.w3.org/2001/XMLSchema}byte" />
* <attribute name="charField" use="required" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="doubleField" use="required" type="{http://www.w3.org/2001/XMLSchema}double" />
* <attribute name="floatField" use="required" type="{http://www.w3.org/2001/XMLSchema}float" />
* <attribute name="intField" use="required" type="{http://www.w3.org/2001/XMLSchema}int" />
* <attribute name="longField" use="required" type="{http://www.w3.org/2001/XMLSchema}long" />
* <attribute name="shortField" use="required" type="{http://www.w3.org/2001/XMLSchema}short" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "XmlFlatPrimitiveAttribute")
@XmlRootElement(name = "XmlFlatPrimitiveAttribute")
public class XmlFlatPrimitiveAttribute
implements Serializable
{
private final static long serialVersionUID = 1L;
@XmlAttribute(name = "booleanField", required = true)
protected boolean booleanField;
@XmlAttribute(name = "byteField", required = true)
protected byte byteField;
@XmlAttribute(name = "charField", required = true)
protected String charField;
@XmlAttribute(name = "doubleField", required = true)
protected double doubleField;
@XmlAttribute(name = "floatField", required = true)
protected float floatField;
@XmlAttribute(name = "intField", required = true)
protected int intField;
@XmlAttribute(name = "longField", required = true)
protected long longField;
@XmlAttribute(name = "shortField", required = true)
protected short shortField;
/**
* Gets the value of the booleanField property.
*
*/
public boolean isBooleanField() {
return booleanField;
}
/**
* Sets the value of the booleanField property.
*
*/
public void setBooleanField(boolean value) {
this.booleanField = value;
}
/**
* Gets the value of the byteField property.
*
*/
public byte getByteField() {
return byteField;
}
/**
* Sets the value of the byteField property.
*
*/
public void setByteField(byte value) {
this.byteField = value;
}
/**
* Gets the value of the charField property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCharField() {
return charField;
}
/**
* Sets the value of the charField property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCharField(String value) {
this.charField = value;
}
/**
* Gets the value of the doubleField property.
*
*/
public double getDoubleField() {
return doubleField;
}
/**
* Sets the value of the doubleField property.
*
*/
public void setDoubleField(double value) {
this.doubleField = value;
}
/**
* Gets the value of the floatField property.
*
*/
public float getFloatField() {
return floatField;
}
/**
* Sets the value of the floatField property.
*
*/
public void setFloatField(float value) {
this.floatField = value;
}
/**
* Gets the value of the intField property.
*
*/
public int getIntField() {
return intField;
}
/**
* Sets the value of the intField property.
*
*/
public void setIntField(int value) {
this.intField = value;
}
/**
* Gets the value of the longField property.
*
*/
public long getLongField() {
return longField;
}
/**
* Sets the value of the longField property.
*
*/
public void setLongField(long value) {
this.longField = value;
}
/**
* Gets the value of the shortField property.
*
*/
public short getShortField() {
return shortField;
}
/**
* Sets the value of the shortField property.
*
*/
public void setShortField(short value) {
this.shortField = value;
}
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final XmlFlatPrimitiveAttribute that = ((XmlFlatPrimitiveAttribute) object);
{
boolean leftBooleanField;
leftBooleanField = this.isBooleanField();
boolean rightBooleanField;
rightBooleanField = that.isBooleanField();
if (leftBooleanField!= rightBooleanField) {
return false;
}
}
{
byte leftByteField;
leftByteField = this.getByteField();
byte rightByteField;
rightByteField = that.getByteField();
if (leftByteField!= rightByteField) {
return false;
}
}
{
String leftCharField;
leftCharField = this.getCharField();
String rightCharField;
rightCharField = that.getCharField();
if (this.charField!= null) {
if (that.charField!= null) {
if (!leftCharField.equals(rightCharField)) {
return false;
}
} else {
return false;
}
} else {
if (that.charField!= null) {
return false;
}
}
}
{
double leftDoubleField;
leftDoubleField = this.getDoubleField();
double rightDoubleField;
rightDoubleField = that.getDoubleField();
if (Double.doubleToLongBits(leftDoubleField)!= Double.doubleToLongBits(rightDoubleField)) {
return false;
}
}
{
float leftFloatField;
leftFloatField = this.getFloatField();
float rightFloatField;
rightFloatField = that.getFloatField();
if (leftFloatField!= rightFloatField) {
return false;
}
}
{
int leftIntField;
leftIntField = this.getIntField();
int rightIntField;
rightIntField = that.getIntField();
if (leftIntField!= rightIntField) {
return false;
}
}
{
long leftLongField;
leftLongField = this.getLongField();
long rightLongField;
rightLongField = that.getLongField();
if (leftLongField!= rightLongField) {
return false;
}
}
{
short leftShortField;
leftShortField = this.getShortField();
short rightShortField;
rightShortField = that.getShortField();
if (leftShortField!= rightShortField) {
return false;
}
}
return true;
}
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
boolean theBooleanField;
theBooleanField = this.isBooleanField();
currentHashCode += (theBooleanField? 1231 : 1237);
}
{
currentHashCode = (currentHashCode* 31);
byte theByteField;
theByteField = this.getByteField();
currentHashCode += ((int) theByteField);
}
{
currentHashCode = (currentHashCode* 31);
String theCharField;
theCharField = this.getCharField();
if (this.charField!= null) {
currentHashCode += theCharField.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
double theDoubleField;
theDoubleField = this.getDoubleField();
final long theDoubleFieldBits = Double.doubleToLongBits(theDoubleField);
currentHashCode += ((int)(theDoubleFieldBits^(theDoubleFieldBits >>> 32)));
}
{
currentHashCode = (currentHashCode* 31);
float theFloatField;
theFloatField = this.getFloatField();
currentHashCode += ((int) theFloatField);
}
{
currentHashCode = (currentHashCode* 31);
int theIntField;
theIntField = this.getIntField();
currentHashCode += theIntField;
}
{
currentHashCode = (currentHashCode* 31);
long theLongField;
theLongField = this.getLongField();
currentHashCode += ((int)(theLongField^(theLongField >>> 32)));
}
{
currentHashCode = (currentHashCode* 31);
short theShortField;
theShortField = this.getShortField();
currentHashCode += ((int) theShortField);
}
return currentHashCode;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy