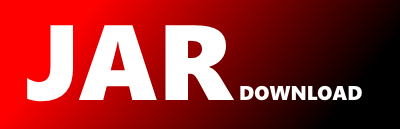
io.atomix.copycat.client.session.ClientSession Maven / Gradle / Ivy
/*
* Copyright 2015 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License
*/
package io.atomix.copycat.client.session;
import io.atomix.catalyst.transport.Client;
import io.atomix.catalyst.util.Assert;
import io.atomix.catalyst.util.Listener;
import io.atomix.catalyst.util.Managed;
import io.atomix.catalyst.util.concurrent.ThreadContext;
import io.atomix.copycat.client.*;
import io.atomix.copycat.client.util.AddressSelector;
import io.atomix.copycat.client.util.ClientConnection;
import java.util.UUID;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
/**
* Client session.
*
* @author onStateChange(Consumer callback) {
return state.onStateChange(callback);
}
/**
* Submits an operation to the session.
*
* @param operation The operation to submit.
* @param The operation result type.
* @return A completable future to be completed with the operation result.
*/
public CompletableFuture submit(Operation operation) {
if (operation instanceof Query) {
return submit((Query) operation);
} else if (operation instanceof Command) {
return submit((Command) operation);
} else {
throw new UnsupportedOperationException("unknown operation type: " + operation.getClass());
}
}
/**
* Submits a command to the session.
*
* @param command The command to submit.
* @param The command result type.
* @return A completable future to be completed with the command result.
*/
public CompletableFuture submit(Command command) {
return submitter.submit(command);
}
/**
* Submits a query to the session.
*
* @param query The query to submit.
* @param The query result type.
* @return A completable future to be completed with the query result.
*/
public CompletableFuture submit(Query query) {
return submitter.submit(query);
}
/**
* Opens the session.
*
* @return A completable future to be completed once the session is opened.
*/
public CompletableFuture open() {
return manager.open().thenApply(v -> this);
}
@Override
public boolean isOpen() {
return state.getState() == State.OPEN || state.getState() == State.UNSTABLE;
}
@Override
public Session publish(String event) {
listener.publish(event);
return this;
}
@Override
public Session publish(String event, Object message) {
listener.publish(event, message);
return this;
}
@Override
public Listener onEvent(String event, Runnable callback) {
return listener.onEvent(event, callback);
}
@Override
public Listener onEvent(String event, Consumer callback) {
return listener.onEvent(event, callback);
}
/**
* Closes the session.
*
* @return A completable future to be completed once the session is closed.
*/
public CompletableFuture close() {
ThreadContext context = ThreadContext.currentContext();
if (context != null) {
return submitter.close()
.thenCompose(v -> listener.close())
.thenCompose(v -> manager.close())
.thenCompose(v -> connection.close())
.whenCompleteAsync((result, error) -> this.context.close(), context.executor());
} else {
return submitter.close()
.thenCompose(v -> listener.close())
.thenCompose(v -> manager.close())
.thenCompose(v -> connection.close())
.whenCompleteAsync((result, error) -> this.context.close());
}
}
/**
* Kills the session.
*
* @return A completable future to be completed once the session has been killed.
*/
public CompletableFuture kill() {
ThreadContext context = ThreadContext.currentContext();
if (context != null) {
return submitter.close()
.thenCompose(v -> listener.close())
.thenCompose(v -> manager.kill())
.thenCompose(v -> connection.close())
.whenCompleteAsync((result, error) -> this.context.close(), context.executor());
} else {
return submitter.close()
.thenCompose(v -> listener.close())
.thenCompose(v -> manager.kill())
.thenCompose(v -> connection.close())
.whenCompleteAsync((result, error) -> this.context.close());
}
}
@Override
public boolean isClosed() {
return state.getState() == State.EXPIRED || state.getState() == State.CLOSED;
}
@Override
public int hashCode() {
int hashCode = 31;
long id = id();
hashCode = 37 * hashCode + (int)(id ^ (id >>> 32));
return hashCode;
}
@Override
public boolean equals(Object object) {
return object instanceof ClientSession && ((ClientSession) object).id() == id();
}
@Override
public String toString() {
return String.format("%s[id=%d]", getClass().getSimpleName(), id());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy