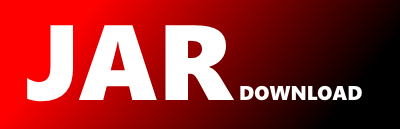
io.atomix.copycat.client.session.ClientSession Maven / Gradle / Ivy
/*
* Copyright 2015 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License
*/
package io.atomix.copycat.client.session;
import io.atomix.catalyst.concurrent.Futures;
import io.atomix.catalyst.concurrent.Listener;
import io.atomix.catalyst.concurrent.ThreadContext;
import io.atomix.catalyst.transport.Client;
import io.atomix.catalyst.util.Assert;
import io.atomix.copycat.Command;
import io.atomix.copycat.Operation;
import io.atomix.copycat.Query;
import io.atomix.copycat.client.ConnectionStrategy;
import io.atomix.copycat.client.util.AddressSelector;
import io.atomix.copycat.client.util.ClientConnection;
import io.atomix.copycat.session.ClosedSessionException;
import io.atomix.copycat.session.Session;
import java.time.Duration;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
/**
* Handles submitting state machine {@link Command commands} and {@link Query queries} to the Copycat cluster.
*
* The client session is responsible for maintaining a client's connection to a Copycat cluster and coordinating
* the submission of {@link Command commands} and {@link Query queries} to various nodes in the cluster. Client
* sessions are single-use objects that represent the context within which a cluster can guarantee linearizable
* semantics for state machine operations. When a session is {@link #register() opened}, the session will register
* itself with the cluster by attempting to contact each of the known servers. Once the session has been successfully
* registered, kee-alive requests will be periodically sent to keep the session alive.
*
* Sessions are responsible for sequencing concurrent operations to ensure they're applied to the system state
* in the order in which they were submitted by the client. To do so, the session coordinates with its server-side
* counterpart using unique per-operation sequence numbers.
*