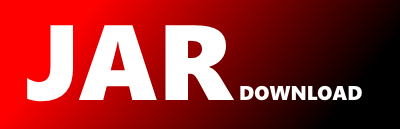
io.atomix.copycat.client.session.ClientSessionState Maven / Gradle / Ivy
/*
* Copyright 2015 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License
*/
package io.atomix.copycat.client.session;
import io.atomix.catalyst.concurrent.Listener;
import io.atomix.catalyst.util.Assert;
import io.atomix.copycat.session.Session;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Set;
import java.util.concurrent.CopyOnWriteArraySet;
import java.util.function.Consumer;
/**
* Client state.
*
* @author l.accept(state));
}
return this;
}
/**
* Registers a state change listener on the session manager.
*
* @param callback The state change listener callback.
* @return The state change listener.
*/
public Listener onStateChange(Consumer callback) {
Listener listener = new Listener() {
@Override
public void accept(Session.State state) {
callback.accept(state);
}
@Override
public void close() {
changeListeners.remove(this);
}
};
changeListeners.add(listener);
return listener;
}
/**
* Sets the last command request sequence number.
*
* @param commandRequest The last command request sequence number.
* @return The client session state.
*/
public ClientSessionState setCommandRequest(long commandRequest) {
this.commandRequest = commandRequest;
return this;
}
/**
* Returns the last command request sequence number for the session.
*
* @return The last command request sequence number for the session.
*/
public long getCommandRequest() {
return commandRequest;
}
/**
* Returns the next command request sequence number for the session.
*
* @return The next command request sequence number for the session.
*/
public long nextCommandRequest() {
return ++commandRequest;
}
/**
* Sets the last command sequence number for which a response has been received.
*
* @param commandResponse The last command sequence number for which a response has been received.
* @return The client session state.
*/
public ClientSessionState setCommandResponse(long commandResponse) {
this.commandResponse = commandResponse;
return this;
}
/**
* Returns the last command sequence number for which a response has been received.
*
* @return The last command sequence number for which a response has been received.
*/
public long getCommandResponse() {
return commandResponse;
}
/**
* Sets the highest index for which a response has been received.
*
* @param responseIndex The highest index for which a command or query response has been received.
* @return The client session state.
*/
public ClientSessionState setResponseIndex(long responseIndex) {
this.responseIndex = Math.max(this.responseIndex, responseIndex);
return this;
}
/**
* Returns the highest index for which a response has been received.
*
* @return The highest index for which a command or query response has been received.
*/
public long getResponseIndex() {
return responseIndex;
}
/**
* Sets the highest index for which an event has been received in sequence.
*
* @param eventIndex The highest index for which an event has been received in sequence.
* @return The client session state.
*/
public ClientSessionState setEventIndex(long eventIndex) {
this.eventIndex = eventIndex;
return this;
}
/**
* Returns the highest index for which an event has been received in sequence.
*
* @return The highest index for which an event has been received in sequence.
*/
public long getEventIndex() {
return eventIndex;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy