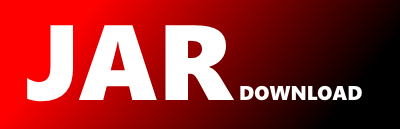
io.aubay.fase.maven.plugin.FaseMojo Maven / Gradle / Ivy
package io.aubay.fase.maven.plugin;
/*-
* #%L
* fase-maven-plugin Maven Plugin
* %%
* Copyright (C) 2018 - 2019 Aubay
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import java.io.File;
import java.util.Arrays;
import java.util.stream.Collectors;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.shared.model.fileset.FileSet;
import org.apache.maven.shared.model.fileset.util.FileSetManager;
import io.aubay.fase.maven.plugin.util.CucumberUtils;
/**
* Mojo pour la transformation de fichier de feature FASe
* en feature Cucumber
*
* Un fichier de feature FASe contient des "Examples" du type :
*
* | faseId | prefix | dataFile | dataFileFormat | dataFileTransformer | dataFileTransformerParams |
* | testExamples | examples | src/test/resources/testing-good-file.csv | CSV | CSV | , |
*
* et devient
*
* | examples.id | examples.numeroDossier | examples.description |
* | 1 | 12345 | Description de mon dossier |
*
*
* avec un fichier src/test/resources/testing-good-file.csv contenant :
*
* id,numeroDossier,description
* 1,12345,Description de mon dossier
*
*
* Les extensions par defaut recherche sont celles de CucumberUtils.FEATURE_FASE_EXTENSION
* Les inclusions se font depuis tous les repertoires et sous-repertoires de src/test
* Aucune exclusions par defaut.
* Le repertoire de sortie "outputDirectory" par defaut est : ${project.build.directory}/fase
*
*/
@Mojo(name = "process-cucumber-features", defaultPhase = LifecyclePhase.PROCESS_TEST_SOURCES)
public class FaseMojo extends AbstractMojo {
private static final ThreadLocal MOJO = new ThreadLocal<>();
private static final String[] DEFAULT_EXCLUDES = new String[] {};
private static final String[] DEFAULT_INCLUDES = new String[] { "src/test/**/**" };
/**
* List of files to include. Specified as fileset patterns which are relative to
* the input directory.
*/
@Parameter
private String[] includes;
/**
* List of files to exclude. Specified as fileset patterns which are relative to
* the input directory.
*/
@Parameter
private String[] excludes;
/**
* Location of the output file.
*/
@Parameter(defaultValue = "${project.build.directory}/fase", property = "outputDir", required = true)
private File outputDirectory;
/**
* Base dir of Maven Project
*/
@Parameter(defaultValue = "${project.basedir}", readonly = true, required = true)
private File basedir;
/**
* File extension of feature's file to look for
*/
@Parameter(defaultValue = CucumberUtils.FEATURE_FASE_EXTENSION, readonly = true, required = true)
private String featureExtension;
public void execute() throws MojoExecutionException {
MOJO.set(this);
getLog().info("Executing FaseMojo to transform Fase Example into a Cucumber Example");
ExamplesReplacer replacer = new ExamplesReplacer();
replacer.setFeaturesPaths(getFeaturesPaths());
replacer.setOutputDirectory(outputDirectory);
replacer.setFeatureExtension(featureExtension);
replacer.executeReplacement();
MOJO.remove();
}
private String[] getIncludes() {
if (includes != null && includes.length > 0) {
return includes;
}
return DEFAULT_INCLUDES;
}
private String[] getExcludes() {
if (excludes != null && excludes.length > 0) {
return excludes;
}
return DEFAULT_EXCLUDES;
}
private String[] getFeaturesPaths() {
FileSetManager fileSetManager = new FileSetManager();
FileSet featuresContentFileSet = new FileSet();
featuresContentFileSet.setDirectory(basedir.getAbsolutePath());
featuresContentFileSet.setIncludes(Arrays.asList(getIncludes()));
featuresContentFileSet.setExcludes(Arrays.asList(getExcludes()));
String[] relativeFeaturesPaths = fileSetManager.getIncludedFiles(featuresContentFileSet);
return Arrays.stream(relativeFeaturesPaths).map(p -> basedir.getAbsolutePath() + File.separator + p).collect(Collectors.toList()).toArray(new String[0]);
}
/**
* Methode permettant de recuperer le repertoire de travail par defaut
* Utile pour les AbstractDataTransformer pour recuperer les fichiers utilises pour les transformations
*
* @return : basedir de ce FaseMojo ou new File(".") si pas de FaseMojo dans le ThreadLocal
*/
public static File getBasedir() {
FaseMojo mojo = MOJO.get();
if(mojo==null) {
return new File(".");
}
return mojo.basedir;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy