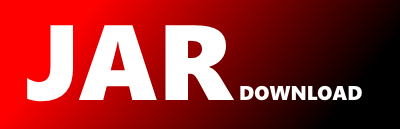
io.aubay.fase.maven.plugin.FaseTable Maven / Gradle / Ivy
package io.aubay.fase.maven.plugin;
/*-
* #%L
* fase-maven-plugin Maven Plugin
* %%
* Copyright (C) 2018 - 2019 Aubay
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import java.util.ArrayList;
import java.util.List;
import java.util.stream.IntStream;
import gherkin.ast.Examples;
import gherkin.ast.TableCell;
import gherkin.ast.TableRow;
/**
*
* Classe representant la FaseTable tel que definit comme ci-dessous dans les Examples :
*
* | faseId | prefix | dataFile | dataFileFormat | dataFileTransformer | dataFileTransformerParams |
* | testExamples | examples | src/test/resources/testing-good-file.csv | CSV | CSV | , |
*
* @author elegeleux
*
*/
public class FaseTable {
/**
* Enum permettant de connaitre les colonnes autorises dans une FaseTable
*
* @author elegeleux
*
*/
public enum ColumnName {
/**
* Identifiant pour FASe
*/
FASE_ID("faseId"),
/**
* Prefix pour l'utilisation dans la feature finale
* Permet de disposer de plusieurs FaseTable dans une meme fichier
*/
PREFIX("prefix"),
/**
* Chemin vers le fichier a utiliser pour le chargement
*/
DATA_FILE("dataFile"),
/**
* Format du fichier
* Permet de verifier que le transformateur associe est compatible avec ce format
*/
DATA_FILE_FORMAT("dataFileFormat"),
/**
* Id du transformateur a utiliser
*/
DATA_FILE_TRANSFORMER("dataFileTransformer"),
/**
* Parametre pour le transformateur (format libre de la donnee dependant du transformateur)
*/
DATA_FILE_TRANSFORMER_PARAMS("dataFileTransformerParams");
private String key;
private ColumnName(String key) {
this.key = key;
}
/**
* Renvoie le nom de la colonne a utiliser dans la FaseTable
* @return : le nom de la colonne a utiliser dans la FaseTable
*/
public String getKey() {
return this.key;
}
/**
* Permet de recuperer l'enum d'une colonne en fonction de son nom
*
* @param key : nom de la colonne dans la FaseTable
* @return enum de la colonne concernee
*/
public static ColumnName parse(String key) {
for(ColumnName columnName : ColumnName.values()) {
if(columnName.getKey().equals(key)) {
return columnName;
}
}
return null;
}
}
private Examples examples;
private List rows;
/**
* Constructeur permettant de concevoir la FaseTable en fonction de l'Examples original
*
* @param examples : Examples a transformer
*/
public FaseTable(Examples examples) {
this.examples = examples;
loadTable();
}
/**
* Renvoie la liste de FaseRow de la table
*
* @return : la liste de FaseRow de la table
*/
public List getRows() {
return rows;
}
private void loadTable() {
validateExamples(examples);
TableRow header = this.examples.getTableHeader();
validateHeader(header);
List headerCells = header.getCells();
rows = new ArrayList<>();
List body = this.examples.getTableBody();
body.stream().forEach(row ->
this.loadRow(row, headerCells)
);
}
private void validateExamples(Examples examples) {
if(examples==null) {
throw new IllegalArgumentException("examples cannot be null");
}
}
private void validateHeader(TableRow header) {
if(header==null) {
throw new IllegalArgumentException("header cannot be null");
}
List cells = header.getCells();
if(cells==null || cells.isEmpty()) {
throw new IllegalArgumentException("cells cannot be null or empty");
}
if(cells.size()<2) {
throw new IllegalArgumentException("cells should contains at least 2 cells");
}
if(!cells.get(0).getValue().equals(FaseTable.ColumnName.FASE_ID.getKey())) {
throw new IllegalArgumentException("content of first cell should be equals to " + FaseTable.ColumnName.FASE_ID.getKey());
}
for(TableCell cell : cells) {
if(ColumnName.parse(cell.getValue())==null) {
throw new IllegalArgumentException("content for cell with value=\"" + cell.getValue() + "\" is INVALID");
}
}
}
private void loadRow(TableRow row, List headerCells) {
FaseRow faseRow = new FaseRow();
IntStream.range(0, headerCells.size())
.forEach(index ->
faseRow.addValue(ColumnName.parse(headerCells.get(index).getValue()), row.getCells().get(index).getValue())
);
this.rows.add(faseRow);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy