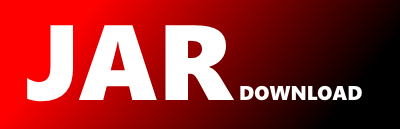
io.aubay.fase.maven.plugin.SourceData Maven / Gradle / Ivy
package io.aubay.fase.maven.plugin;
/*-
* #%L
* fase-maven-plugin Maven Plugin
* %%
* Copyright (C) 2018 - 2019 Aubay
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.apache.logging.log4j.message.Message;
import org.apache.logging.log4j.message.ParameterizedMessage;
import gherkin.ast.Examples;
import gherkin.events.SourceEvent;
import io.aubay.fase.maven.plugin.util.CucumberUtils;
import io.aubay.fase.maven.plugin.util.FileUtils;
import io.aubay.fase.maven.plugin.util.GherkinUtils;
import io.cucumber.datatable.DataTable;
public class SourceData {
private static final Logger LOG = LogManager.getLogger();
private static final String LINE_SEPARATOR_REPLACER = "";
private static final String WINDOWS_LINE_SEPARATOR = "\\r\\n";
private static final String LINUX_MAC_LINE_SEPARATOR = "\\n";
private SourceEvent sourceEvent;
private List newData = new ArrayList<>();
private List originalData = new ArrayList<>();
private int lastReplacementIndex = 0;
public SourceData(SourceEvent sourceEvent) {
this.sourceEvent = sourceEvent;
loadOriginalData();
}
public void loadExamples(Examples examples) {
if(originalData.size() dataTable = GherkinUtils.examplesToDatatable(examples);
if (dataTable.isPresent()) {
newData.add(dataTable.get().toString());
}
lastReplacementIndex = examples.getLocation().getLine() + examples.getTableBody().size() + 1;
}
public List getNewData(){
return this.newData;
}
public boolean saveNewData(File outputDirectory) {
if(outputDirectory==null) {
return false;
}
String sourceUriToOutputUri = FileUtils.sourceUriToOutputDirectoryUri(sourceEvent.uri,
outputDirectory.getAbsolutePath());
sourceUriToOutputUri = sourceUriToOutputUri.replace(CucumberUtils.FEATURE_FASE_EXTENSION, CucumberUtils.FEATURE_EXTENSION);
LOG.info("sourceUriToOutputUri={}", sourceUriToOutputUri);
String data = String.join(System.getProperty(SystemProperties.LINE_SEPARATOR.getKey()), this.newData);
File fileToWrite = new File(sourceUriToOutputUri);
try {
FileUtils.writeDataInFile(data, fileToWrite);
} catch (IOException e) {
Message msg = new ParameterizedMessage("Cannot write data={} to file={}", data, fileToWrite, e);
LOG.error(msg);
}
return true;
}
private void loadOriginalData() {
String tempData = sourceEvent.data.replaceAll(WINDOWS_LINE_SEPARATOR, LINE_SEPARATOR_REPLACER);
tempData = tempData.replaceAll(LINUX_MAC_LINE_SEPARATOR, LINE_SEPARATOR_REPLACER);
LOG.info("tempData={}", tempData);
this.originalData.addAll(Arrays.asList(tempData.split(LINE_SEPARATOR_REPLACER)));
//this.originalData.addAll(Arrays.asList(sourceEvent.data.split(System.getProperty(SystemProperties.LINE_SEPARATOR.getKey()))));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy