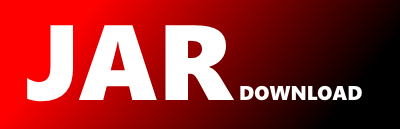
io.aubay.fase.maven.plugin.util.FileUtils Maven / Gradle / Ivy
package io.aubay.fase.maven.plugin.util;
/*-
* #%L
* fase-maven-plugin Maven Plugin
* %%
* Copyright (C) 2018 - 2019 Aubay
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardOpenOption;
import java.nio.file.attribute.PosixFileAttributeView;
import java.nio.file.attribute.PosixFileAttributes;
import java.nio.file.attribute.PosixFilePermission;
import java.util.Set;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class FileUtils {
private static final Logger LOG = LogManager.getLogger();
public static String getMavenRelativePath(String path) {
if (path == null) {
throw new IllegalArgumentException("path CANNOT BE NULL");
}
File pathFile = new File(path);
File parentFile = pathFile.getParentFile();
File mavenSourceFile = null;
StringBuilder relativePath = new StringBuilder(pathFile.getName());
while (parentFile != null && mavenSourceFile == null) {
String directoryName = parentFile.getName();
if (!directoryName.equals("java") && !directoryName.equals("resources")) {
relativePath.insert(0, File.separator);
relativePath.insert(0, parentFile.getName());
parentFile = parentFile.getParentFile();
} else {
mavenSourceFile = parentFile;
}
}
return relativePath.toString();
}
public static String sourceUriToOutputDirectoryUri(String sourceUri, String outputDirectory) {
StringBuilder result = new StringBuilder();
if (outputDirectory != null && !"".equals(outputDirectory)) {
result.append(outputDirectory).append(File.separator);
}
result.append(getMavenRelativePath(sourceUri));
return result.toString();
}
public static boolean writeDataInFile(String data, File file) throws IOException {
if (file == null) {
throw new IllegalArgumentException("file CANNOT BE NULL");
}
LOG.info("Checking and creating directories for file={}", file);
checkAndCreateDirectories(file);
Files.write(file.toPath(), data.getBytes(), StandardOpenOption.CREATE, StandardOpenOption.WRITE);
return true;
}
public static boolean checkAndCreateDirectories(File file) throws IOException {
// Control input param
if (file == null) {
throw new IllegalArgumentException("file CANNOT BE NULL");
}
// Control that input param isAbsolute
if (!file.isAbsolute()) {
throw new IllegalArgumentException("file SHOULD BE ABSOLUTE");
}
// Control parent directories
File parentFile = file.getParentFile();
// Control if directories for parent exists and try to create if not
if (!parentFile.exists()) {
Files.createDirectories(Paths.get(parentFile.getAbsolutePath()));
}
// Control if parent can be write
if (isFileReadOnly(parentFile)) {
throw new IllegalArgumentException("parentFile SHOULD BE WRITABLE");
}
return true;
}
protected static boolean isFileReadOnly(File file) {
boolean isReadOnly = false;
if (System.getProperty("os.name").startsWith("Windows")) {
// All Windows versions
isReadOnly = !file.canWrite();
} else {
// All Unix-like OSes.
Path path = file.toPath();
PosixFileAttributes attributes = null;
try {
attributes = Files.getFileAttributeView(path, PosixFileAttributeView.class).readAttributes();
} catch (java.io.IOException e) {
// File presence is guaranteed. Ignore
LOG.error("Cannot getFileAttributeView for path={}", path, e);
}
if (attributes != null) {
// A file is read-only in Linux only when it has 0444 permissions.
Set permissions = attributes.permissions();
if (!permissions.contains(PosixFilePermission.OWNER_WRITE)
&& !permissions.contains(PosixFilePermission.GROUP_WRITE)
&& !permissions.contains(PosixFilePermission.OTHERS_WRITE)) {
isReadOnly = true;
}
}
}
return isReadOnly;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy