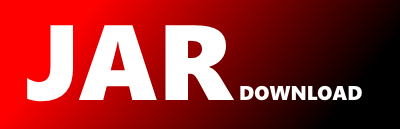
io.aubay.fase.maven.plugin.util.GherkinUtils Maven / Gradle / Ivy
package io.aubay.fase.maven.plugin.util;
/*-
* #%L
* fase-maven-plugin Maven Plugin
* %%
* Copyright (C) 2018 - 2019 Aubay
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import gherkin.ast.Examples;
import gherkin.ast.Feature;
import gherkin.ast.GherkinDocument;
import gherkin.ast.ScenarioDefinition;
import gherkin.ast.ScenarioOutline;
import gherkin.events.CucumberEvent;
import gherkin.events.GherkinDocumentEvent;
import gherkin.events.SourceEvent;
import gherkin.stream.GherkinEvents;
import gherkin.stream.SourceEvents;
import io.aubay.fase.maven.plugin.FaseRow;
import io.aubay.fase.maven.plugin.FaseTable;
import io.cucumber.datatable.DataTable;
public class GherkinUtils {
private static final Logger LOG = LogManager.getLogger();
public static Optional examplesToDatatable(Examples examples) {
if (examples == null) {
return Optional.empty();
}
FaseTable faseTable = new FaseTable(examples);
List faseRows = faseTable.getRows();
List> data = new ArrayList<>();
faseRows.stream().forEach(faseRow -> mergeRows(data, faseRow));
return Optional.of(DataTable.create(data));
}
public static List> mergeRows(List> data, FaseRow faseRow) {
if(faseRow==null) {
throw new IllegalArgumentException("faseRow parameter CANNOT BE NULL");
}
if(!faseRow.isValid()) {
throw new IllegalArgumentException("faseRow parameter IS NOT VALID");
}
if(!faseRow.isDataLoaded()) {
faseRow.loadData();
if(!faseRow.isDataLoaded()) {
throw new IllegalArgumentException("faseRow parameter IS UNABLE TO LOAD DATA");
}
}
if(data==null) {
data = new ArrayList<>();
}
if(data.isEmpty()) {
data.add(faseRow.getHeader());
data.addAll(faseRow.getRows());
} else {
List dataHeaders = data.get(0);
List faseHeaders = faseRow.getHeader();
List> faseRows = faseRow.getRows();
dataHeaders.addAll(faseHeaders);
for(int i=1;i row = data.get(i);
if(row!=null) {
if(i-1row.add(""));
}
}
}
}
return data;
}
public static SourceEvent getSourceEvent(String path) {
if(path==null) {
throw new IllegalArgumentException("path parameter CANNOT BE NULL");
}
List paths = new ArrayList<>();
paths.add(path);
SourceEvents sourceEvents = new SourceEvents(paths);
return sourceEvents.iterator().next();
}
public static List getCucumberEvents(List paths, boolean printSource, boolean printAst,
boolean printPickles) {
if (paths == null || paths.isEmpty()) {
return Collections.emptyList();
}
List cucumberEvents = new ArrayList<>();
SourceEvents sourceEvents = new SourceEvents(paths);
GherkinEvents gherkinEvents = new GherkinEvents(printSource, printAst, printPickles);
for (SourceEvent sourceEvent : sourceEvents) {
for (CucumberEvent cucumberEvent : gherkinEvents.iterable(sourceEvent)) {
cucumberEvents.add(cucumberEvent);
}
}
return cucumberEvents;
}
public static List getFeatures(List cucumberEvents) {
if (cucumberEvents == null || cucumberEvents.isEmpty()) {
return new ArrayList<>();
}
return cucumberEvents.stream().filter(ce -> ce instanceof GherkinDocumentEvent)
.map(ce -> (GherkinDocumentEvent) ce).map(gde -> gde.document).map(GherkinDocument::getFeature)
.collect(Collectors.toList());
}
public static Map> getScenarioOutlinesByFeature(List cucumberEvents) {
if (cucumberEvents == null || cucumberEvents.isEmpty()) {
return new HashMap<>();
}
List features = getFeatures(cucumberEvents);
Map> scenarioDefinitionsByFeature = features.stream()
.filter(f -> f.getChildren() != null).collect(Collectors.toMap(f -> f, f -> f.getChildren()));
return scenarioDefinitionsByFeature.entrySet().stream()
.collect(Collectors.toMap(Map.Entry::getKey,
e -> e.getValue().stream().filter(sd -> sd instanceof ScenarioOutline)
.map(sd -> (ScenarioOutline) sd).collect(Collectors.toList())));
}
public static Map>> getExamplesByFeatureAndScenarioOutline(
List cucumberEvents) {
if (cucumberEvents == null || cucumberEvents.isEmpty()) {
return new HashMap<>();
}
Map> scenarioOutlinesByFeature = getScenarioOutlinesByFeature(cucumberEvents);
return scenarioOutlinesByFeature.entrySet().stream().collect(
Collectors.toMap(Map.Entry::getKey, e -> e.getValue().stream().filter(so -> so.getExamples() != null)
.collect(Collectors.toMap(Function.identity(), ScenarioOutline::getExamples))));
}
public static Map>> getFaseTablesByFeatureAndScenarioOutline(
List paths) {
if (paths == null || paths.isEmpty()) {
return Collections.emptyMap();
}
return getExamplesByFeatureAndScenarioOutline(getCucumberEvents(paths, false, true, false)).entrySet().stream()
.collect(Collectors.toMap(Map.Entry::getKey,
entry -> entry.getValue().entrySet().stream()
.collect(Collectors.toMap(Map.Entry::getKey, entry2 -> entry2.getValue().stream()
.map(FaseTable::new).collect(Collectors.toList())))));
}
private GherkinUtils() {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy