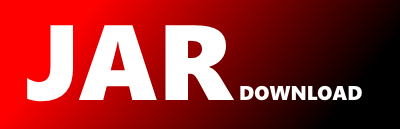
io.avaje.json.simple.SimpleMapper Maven / Gradle / Ivy
package io.avaje.json.simple;
import io.avaje.json.JsonAdapter;
import io.avaje.json.JsonReader;
import io.avaje.json.JsonWriter;
import io.avaje.json.PropertyNames;
import io.avaje.json.stream.JsonStream;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.Reader;
import java.io.Writer;
import java.util.List;
import java.util.Map;
/**
* A mapper for mapping to basic Java types.
*
* This supports the basic Java types of String, Boolean, Integer, Long, Double and
* Maps and List of these.
*
* For full support with more types and binding to custom types use avaje-jsonb instead.
*
*
Example
* {@code
*
* static final SimpleMapper simpleMapper = SimpleMapper.builder().build();
*
* Map map = new LinkedHashMap<>();
* map.put("one", 45L);
* map.put("two", 93L);
*
* String asJson = simpleMapper.toJson(map);
*
* }
*/
public interface SimpleMapper {
/**
* Create a new builder for SimpleMapper.
*/
static Builder builder() {
return new DSimpleMapperBuilder();
}
/**
* Return a mapper for any json content.
*/
Type
© 2015 - 2024 Weber Informatics LLC | Privacy Policy