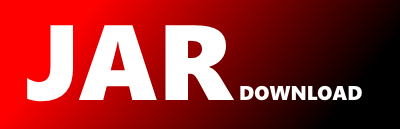
io.avaje.validation.core.ResourceBundleManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of avaje-validator Show documentation
Show all versions of avaje-validator Show documentation
validator for annotated pojos using constraint annotations and source code generation
package io.avaje.validation.core;
import static java.lang.System.Logger.Level.ERROR;
import static java.util.ResourceBundle.getBundle;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.MissingResourceException;
import java.util.ResourceBundle;
import org.jspecify.annotations.Nullable;
import io.avaje.applog.AppLog;
final class ResourceBundleManager {
private static final System.Logger logger = AppLog.getLogger(ResourceBundleManager.class);
private final Map> map = new HashMap<>();
private static final List EMPTY = List.of();
private static final String DEFAULT_BUNDLE = "io.avaje.validation.Messages";
ResourceBundleManager(List names, List providedBundles, LocaleResolver localeResolver) {
for (final var name : names) {
addBundle(name, localeResolver.defaultLocale());
for (final Locale locale : localeResolver.otherLocales()) {
addBundle(name, locale);
}
}
for (final var bundle : providedBundles) {
map.computeIfAbsent(bundle.getLocale(), key -> new ArrayList<>()).add(bundle);
}
// since default is added last, it will be the last place messages will be resolved
addBundle(DEFAULT_BUNDLE, localeResolver.defaultLocale());
for (final Locale locale : localeResolver.otherLocales()) {
addBundle(DEFAULT_BUNDLE, locale);
}
}
private void addBundle(final String name, final Locale locale) {
try {
map.computeIfAbsent(locale, key -> new ArrayList<>()).add(getBundle(name, locale));
} catch (MissingResourceException e) {
logger.log(ERROR, "failed to load " + name + " with locale " + locale);
}
}
@Nullable
public String message(String template, Locale resolvedLocale) {
for (final var bundle : map.getOrDefault(resolvedLocale, EMPTY)) {
if (bundle.containsKey(template)) {
return bundle.getString(template);
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy