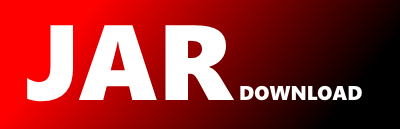
io.axual.connect.plugins.http.HttpSinkConnector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of http-sink-connector Show documentation
Show all versions of http-sink-connector Show documentation
This connector is used to call an HTTP service for each record on a topic
package io.axual.connect.plugins.http;
/*-
* ========================LICENSE_START=================================
* HTTP Sink Connector for Kafka Connect
* %%
* Copyright (C) 2020 Axual B.V.
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* =========================LICENSE_END==================================
*/
import static io.axual.connect.plugins.http.HttpSinkConnectorConfig.ENDPOINT_CONFIG;
import io.axual.connect.plugins.http.exceptions.HttpSinkConnectorException;
import io.axual.connect.plugins.http.helpers.SslHelper;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.net.SocketFactory;
import javax.net.ssl.SSLContext;
import javax.net.ssl.SSLHandshakeException;
import javax.net.ssl.SSLSocket;
import javax.net.ssl.SSLSocketFactory;
import org.apache.kafka.common.config.Config;
import org.apache.kafka.common.config.ConfigDef;
import org.apache.kafka.common.config.ConfigValue;
import org.apache.kafka.connect.connector.Task;
import org.apache.kafka.connect.sink.SinkConnector;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* The HTTP Sink Connector class is used for sending records to a specific HTTP endpoint.
* It accepts the configuration options defined in {@link HttpSinkConnectorConfig}.
The
* corresponding task class for this connector is {@link HttpSinkTask}.
*
* It will always create the maximum number of task configurations, just passing all
* configuration properties on to the task.
When {@link #validate(Map)} is called it will create
* an enriched configuration definition using {@link HttpSinkConnectorConfig#getConfigurationDefinition(Map)}
* and validate the supplied properties.
If no validation errors are found, the connector will
* try to open a socket on the target host to verify the endpoint and SSL configuration.
*
*/
public class HttpSinkConnector extends SinkConnector {
private static final Logger LOG = LoggerFactory.getLogger(HttpSinkConnector.class);
Map connectorConfig = new HashMap<>();
final SslHelper sslHelper;
/**
* Constructor to inject sslHelper for testing
*
* @param sslHelper
*/
HttpSinkConnector(SslHelper sslHelper) {
this.sslHelper = sslHelper;
}
/**
* Default constructor required for instantiation of connector
*/
public HttpSinkConnector() {
this.sslHelper = SslHelper.INSTANCE;
}
@Override
public void start(Map config) {
LOG.info("Starting connector");
if (!connectorConfig.isEmpty()) {
this.context.raiseError(
new HttpSinkConnectorException("Trying to start a connector which is not stopped"));
return;
}
connectorConfig.putAll(config);
}
@Override
public Class extends Task> taskClass() {
return HttpSinkTask.class;
}
@Override
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy