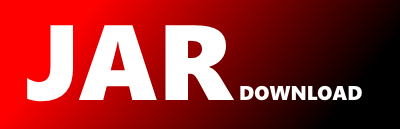
io.axual.connect.plugins.http.authentication.AuthenticationProviderConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of http-sink-connector Show documentation
Show all versions of http-sink-connector Show documentation
This connector is used to call an HTTP service for each record on a topic
package io.axual.connect.plugins.http.authentication;
/*-
* ========================LICENSE_START=================================
* HTTP Sink Connector for Kafka Connect
* %%
* Copyright (C) 2020 Axual B.V.
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* =========================LICENSE_END==================================
*/
import static io.axual.connect.plugins.http.HttpSinkConnectorConfig.ENDPOINT_CONFIG;
import io.axual.connect.plugins.http.HttpSinkConnectorConfig;
import java.net.URI;
import java.util.Map;
import java.util.Objects;
import org.apache.kafka.common.config.AbstractConfig;
import org.apache.kafka.common.config.ConfigDef;
/**
* The AuthenticationProviderConfig is a base class for AuthenticationProviders. It adds the
* ENDPOINT_CONFIG definition to provide the authorisation provider implementations with the target
* URI containing hostname and port. These are often required to set up a correct authorisation
* scope
*/
public class AuthenticationProviderConfig extends AbstractConfig {
/**
* Enriched the provided definition with the endpoint definition
*
* @param original the provided, original configuration definition
* @return A new configuration definition enriched with the endpoint definition
*/
private static ConfigDef enrichConfigDef(ConfigDef original) {
return new ConfigDef(original).define(HttpSinkConnectorConfig.ENDPOINT_CONFIG_KEY);
}
private final URI endpointUri;
/**
* Enriches the provided configuration definition and creates the configuration object It will add
* the endpoint configuration of HttpSinkConnectorConfig to the Authentication Provider property
*
* @param configDef The configuration definition which should be expanded with the endpoint
* @param originals the configuration properties to use for constructing the Config object
*/
public AuthenticationProviderConfig(ConfigDef configDef,
Map, ?> originals) {
super(enrichConfigDef(configDef), originals);
endpointUri = URI.create(getString(ENDPOINT_CONFIG));
}
/**
* The full URI which was set as endpoint for the Sink connector
*
* @return the endpoint as URI
*/
public URI getEndpointUri() {
return endpointUri;
}
/**
* Returns the URI scheme defined for the URI
*
* @return the scheme used
*/
public String getEndpointScheme() {
return endpointUri.getScheme();
}
/**
* Returns the specified host or the URI
*
* @return the host or null if none set
*/
public String getEndpointHost() {
return endpointUri.getHost();
}
/**
* Return the port number set for the URI. If no port number, or a negative port number was used
* it will return 80 if the HTTP scheme is used, 443 is HTTPS scheme is used, or else -1
*
* @return The port number for this connection
*/
public int getEndpointPort() {
int port = endpointUri.getPort();
if (port < 0) {
// get default port
switch (getEndpointScheme().toLowerCase()) {
case "http":
port = 80;
break;
case "https":
port = 443;
break;
default:
break;
}
}
return port;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof AuthenticationProviderConfig)) {
return false;
}
if (!super.equals(o)) {
return false;
}
AuthenticationProviderConfig that = (AuthenticationProviderConfig) o;
return endpointUri.equals(that.endpointUri);
}
@Override
public int hashCode() {
return Objects.hash(super.hashCode(), endpointUri);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy