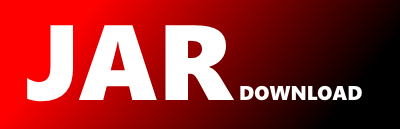
io.axual.connect.plugins.http.authentication.BasicAuthenticationProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of http-sink-connector Show documentation
Show all versions of http-sink-connector Show documentation
This connector is used to call an HTTP service for each record on a topic
package io.axual.connect.plugins.http.authentication;
/*-
* ========================LICENSE_START=================================
* HTTP Sink Connector for Kafka Connect
* %%
* Copyright (C) 2020 Axual B.V.
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* =========================LICENSE_END==================================
*/
import io.axual.connect.plugins.http.exceptions.ConfigurationException;
import io.axual.connect.plugins.http.sender.IAuthenticationProvider;
import java.io.IOException;
import java.util.Map;
import org.apache.http.HttpException;
import org.apache.http.HttpRequest;
import org.apache.http.HttpRequestInterceptor;
import org.apache.http.auth.AuthScope;
import org.apache.http.auth.AuthState;
import org.apache.http.auth.Credentials;
import org.apache.http.auth.UsernamePasswordCredentials;
import org.apache.http.client.CredentialsProvider;
import org.apache.http.client.protocol.HttpClientContext;
import org.apache.http.impl.auth.BasicScheme;
import org.apache.http.impl.client.BasicCredentialsProvider;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.protocol.HttpContext;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* This class provides Basic Authentication support for the HTTP Sink Connector. This provider
* supports Preemptive Authentication, which can be enabled to force the connector to always send
* the Basic Authentication header. The provider is configured with the options found in {@link
* BasicAuthenticationProviderConfig}.
*
* To use the Basic Authentication Provider the setting {@link io.axual.connect.plugins.http.HttpSinkConnectorConfig#AUTHENTICATION_PROVIDER_CLASS_CONFIG}
* must be set to this class, and the configuration options of {@link BasicAuthenticationProviderConfig}
* must be prefixed with {@link io.axual.connect.plugins.http.HttpSinkConnectorConfig#AUTHENTICATION_PROVIDER_CLASS_PARAM_PREFIX}
*/
public class BasicAuthenticationProvider implements IAuthenticationProvider {
private static final Logger LOG = LoggerFactory.getLogger(BasicAuthenticationProvider.class);
BasicCredentialsProvider credentialsProvider;
PreemptiveInterceptor preemptiveInterceptor = null;
@Override
public void configure(Map configs) {
LOG.debug("Configuring for basic authentication");
BasicAuthenticationProviderConfig config = new BasicAuthenticationProviderConfig(configs);
credentialsProvider = new BasicCredentialsProvider();
Credentials credentials = new UsernamePasswordCredentials(config.getUsername(),
config.getPassword() == null ? null : config.getPassword().value());
LOG.debug("Creating credentials for ANY AuthScope");
credentialsProvider.setCredentials(AuthScope.ANY, credentials);
if (config.isPreemptiveAuthenticationEnabled()) {
LOG.debug("Creating Preemptive Interceptor");
preemptiveInterceptor = new PreemptiveInterceptor();
} else {
preemptiveInterceptor = null;
}
}
@Override
public HttpClientBuilder addAuthentication(HttpClientBuilder clientBuilder) {
LOG.debug("Adding authentication");
if (credentialsProvider == null) {
throw new ConfigurationException("Provider not yet configured");
}
if (preemptiveInterceptor != null) {
LOG.debug("Adding Preemptive Interceptor");
clientBuilder.addInterceptorFirst(preemptiveInterceptor);
}
LOG.debug("Setting default credentials provider");
return clientBuilder.setDefaultCredentialsProvider(credentialsProvider);
}
static class PreemptiveInterceptor implements HttpRequestInterceptor {
private static final Logger LOG = LoggerFactory.getLogger(PreemptiveInterceptor.class);
@Override
public void process(HttpRequest request, HttpContext context)
throws HttpException, IOException {
LOG.debug("Processing intercepted message");
AuthState authState = (AuthState) context.getAttribute(HttpClientContext.TARGET_AUTH_STATE);
if (authState.getAuthScheme() == null) {
LOG.debug("No AuthScheme found in AuthState");
CredentialsProvider credsProvider = (CredentialsProvider)
context.getAttribute(HttpClientContext.CREDS_PROVIDER);
Credentials creds = credsProvider.getCredentials(AuthScope.ANY);
if (creds != null) {
LOG.debug("Updating AuthState with BasicScheme");
authState.update(new BasicScheme(), creds);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy