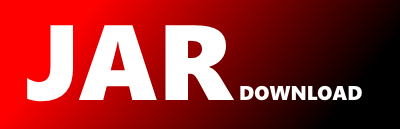
io.axual.connect.plugins.http.headerselection.BasicHeaderSelector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of http-sink-connector Show documentation
Show all versions of http-sink-connector Show documentation
This connector is used to call an HTTP service for each record on a topic
package io.axual.connect.plugins.http.headerselection;
/*-
* ========================LICENSE_START=================================
* HTTP Sink Connector for Kafka Connect
* %%
* Copyright (C) 2020 Axual B.V.
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* =========================LICENSE_END==================================
*/
import io.axual.connect.plugins.http.sender.HttpSenderRequest;
import io.axual.connect.plugins.http.sender.IHeaderSelector;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.http.Header;
import org.apache.http.message.BasicHeader;
import org.apache.kafka.common.config.ConfigDef;
import org.apache.kafka.connect.sink.SinkRecord;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* The Basic Header Selector is used to select headers from the SinkRecord that is processed, and
* send it in the HTTP request as a HTTP header.
* The selector is configured with the options found in {@link BasicHeaderSelectorConfig}
*
*
* To use the Basic Authentication Provider the setting {@link io.axual.connect.plugins.http.HttpSinkConnectorConfig#HEADER_SELECTOR_CLASS_CONFIG}
* must be set to this class, and the configuration options of {@link BasicHeaderSelectorConfig}
* must be prefixed with {@link io.axual.connect.plugins.http.HttpSinkConnectorConfig#HEADER_SELECTOR_CLASS_PARAM_PREFIX}
*
* Currently only String headers are supported by this selectors
*/
public class BasicHeaderSelector implements IHeaderSelector {
private static final Logger LOG = LoggerFactory.getLogger(BasicHeaderSelector.class);
private Set stringHeaderKeys = new HashSet<>();
@Override
public ConfigDef configDefinition(Map configs) {
LOG.debug("Enriching configuration definition for configuration");
return BasicHeaderSelectorConfig.enrichConfig(configs);
}
@Override
public void configure(Map configs) {
LOG.debug("Configuring header selector");
BasicHeaderSelectorConfig config = new BasicHeaderSelectorConfig(configs);
stringHeaderKeys.clear();
for (String alias : config.getHeaderAliases()) {
stringHeaderKeys.add(config.getHeaderKey(alias));
}
LOG.debug("Using header keys {}", stringHeaderKeys);
}
@Override
public HttpSenderRequest selectHeaders(HttpSenderRequest request) {
LOG.debug("Selecting header for request");
List headers = request.getSelectedHeaders();
SinkRecord record = request.getSinkRecord();
final String topic = record.topic();
final Integer partition = record.kafkaPartition();
final long offset = record.kafkaOffset();
for (String headerKey : stringHeaderKeys) {
org.apache.kafka.connect.header.Header connectHeader = record.headers()
.lastWithName(headerKey);
if (connectHeader != null) {
if (connectHeader.value() instanceof CharSequence) {
BasicHeader newHeader = new BasicHeader(headerKey, connectHeader.value().toString());
headers.add(newHeader);
LOG.debug("Adding header {} from record from topic-partition {}-{} and offset {}",
newHeader, topic, partition, offset);
} else {
LOG.debug(
"Value of header {} is not a String. Record metadata topic-partition {}-{} and offset {}",
headerKey, topic, partition, offset);
}
} else {
LOG.debug("Could not find key {} in record from topic-partition {}-{} and offset {}",
headerKey, topic, partition, offset);
}
}
return request;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy