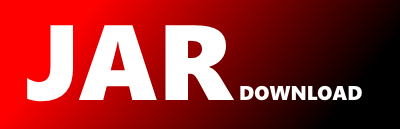
io.axual.connect.plugins.http.headerselection.BasicHeaderSelectorConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of http-sink-connector Show documentation
Show all versions of http-sink-connector Show documentation
This connector is used to call an HTTP service for each record on a topic
package io.axual.connect.plugins.http.headerselection;
/*-
* ========================LICENSE_START=================================
* HTTP Sink Connector for Kafka Connect
* %%
* Copyright (C) 2020 Axual B.V.
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* =========================LICENSE_END==================================
*/
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import org.apache.kafka.common.config.AbstractConfig;
import org.apache.kafka.common.config.ConfigDef;
import org.apache.kafka.common.config.ConfigDef.Importance;
import org.apache.kafka.common.config.ConfigDef.NonEmptyStringWithoutControlChars;
import org.apache.kafka.common.config.ConfigDef.Type;
import org.apache.kafka.common.config.ConfigException;
/**
* Provides the configuration and validation definitions for the {@link BasicHeaderSelector}
* Because of the dynamic nature of the configuration properties the definitions are created
* with
* the {@link #enrichConfig(Map)} method.
*/
public class BasicHeaderSelectorConfig extends AbstractConfig {
public static final String HEADER_ALIAS_CONFIG = "headers";
private static final String HEADER_ALIAS_DEFAULT = "";
private static final String HEADER_ALIAS_DOC = "This is a comma separated list of header alias for the header. Example headers=ab,cd would need configurations headers.ab.key=Custom-Header1 and headers.cd=Another-Header";
public static final String HEADER_KEY_CONFIG_FORMAT = HEADER_ALIAS_CONFIG + ".%s.key";
private static final String HEADER_KEY_DOC = "The actual Kafka Header name to use";
/**
* Creates the Configuration Definition based on the configuration properties provided.
When
* the {@link #HEADER_ALIAS_CONFIG} is set it will use the aliases to create the additional
* configuration definitions per alias
*
* @param configs The map with configuration properties
* @return The configuration definition enriched according to the contents of the provided
* properties
*/
public static ConfigDef enrichConfig(Map, ?> configs) {
Object headerAliases = ConfigDef
.parseType(HEADER_ALIAS_CONFIG, configs.get(HEADER_ALIAS_CONFIG), Type.LIST);
ConfigDef newDef = new ConfigDef()
.define(HEADER_ALIAS_CONFIG, Type.LIST, HEADER_ALIAS_DEFAULT, Importance.HIGH,
HEADER_ALIAS_DOC);
if (!(headerAliases instanceof List)) {
// happens when HEADER_ALIAS_CONFIG is not set
return newDef;
}
LinkedHashSet> uniqueHeaderAliases = new LinkedHashSet<>((List>) headerAliases);
for (Object aliasElement : uniqueHeaderAliases) {
if (!(aliasElement instanceof String)) {
throw new ConfigException(
"All elements of " + HEADER_ALIAS_CONFIG + " property should be of type String");
}
final String alias = ((String) aliasElement).trim();
if (((String) aliasElement).isEmpty()) {
throw new ConfigException(
"An element of " + HEADER_ALIAS_CONFIG + " property is an empty String");
}
final String keyConfig = String.format(HEADER_KEY_CONFIG_FORMAT, alias);
newDef.define(keyConfig, Type.STRING, ConfigDef.NO_DEFAULT_VALUE,
new NonEmptyStringWithoutControlChars(), Importance.HIGH, HEADER_KEY_DOC);
}
return newDef;
}
public BasicHeaderSelectorConfig(Map, ?> originals) {
super(enrichConfig(originals), originals);
}
/**
* @return Returns the list of aliases defined in the properties
*/
public List getHeaderAliases() {
return getList(HEADER_ALIAS_CONFIG);
}
/**
* Returns the header key for a specific alias.
This key is the exact name of the required
* Kafka Header
*
* @param alias the alias to retrieve the Kafka Header key for
* @return the exact name of the required Kafka Header the alias refers to
*/
public String getHeaderKey(String alias) {
return getString(String.format(HEADER_KEY_CONFIG_FORMAT, alias));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy