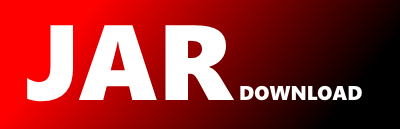
io.axual.connect.plugins.http.sender.HttpSenderRetryStrategy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of http-sink-connector Show documentation
Show all versions of http-sink-connector Show documentation
This connector is used to call an HTTP service for each record on a topic
package io.axual.connect.plugins.http.sender;
/*-
* ========================LICENSE_START=================================
* HTTP Sink Connector for Kafka Connect
* %%
* Copyright (C) 2020 Axual B.V.
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* =========================LICENSE_END==================================
*/
import java.io.IOException;
import java.util.Arrays;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.apache.http.HttpResponse;
import org.apache.http.HttpStatus;
import org.apache.http.client.HttpRequestRetryHandler;
import org.apache.http.client.ServiceUnavailableRetryStrategy;
import org.apache.http.protocol.HttpContext;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* This Retry Strategy combines the Apache Http Client {@code ServiceUnavailableRetryStrategy} and
*
* {@code HttpRequestRetryHandler} to handle both Exceptions and invalid status codes.
The class
* accepts a list of HTTP status codes considered to indicate a successful send, a maximum retry
* count and a wait time between retries.
If an invalid status code is received the client will
* wait for the set wait time, but when Exceptions occur it will retry immediately.
*/
public class HttpSenderRetryStrategy implements
ServiceUnavailableRetryStrategy, HttpRequestRetryHandler {
private static final Logger LOG = LoggerFactory.getLogger(HttpSenderRetryStrategy.class);
public static final int SC_PERMANENT_REDIRECT = 308;
private static final List REDIRECT_CODES = Arrays.asList(
HttpStatus.SC_MOVED_PERMANENTLY,
HttpStatus.SC_MOVED_TEMPORARILY,
HttpStatus.SC_TEMPORARY_REDIRECT,
HttpStatus.SC_TEMPORARY_REDIRECT,
//Permanent Redirect
SC_PERMANENT_REDIRECT,
HttpStatus.SC_SEE_OTHER
);
final Set validStatusCodes;
final int maximumRetries;
final int retryWaitMs;
/**
* Create the Retry Strategy
*
* @param validStatusCodes A list of HTTP status codes which indicate a successful send request
* @param maximumRetries The maximum amount of retries before failing the send request
* @param retryWaitMs the number of milliseconds to wait between retries when an invalid
* status code was received
*/
public HttpSenderRetryStrategy(List validStatusCodes, int maximumRetries,
int retryWaitMs) {
LOG.debug("Creating retry strategy, maximum retries {}, retry wait ms {}, valid codes {}",
maximumRetries, retryWaitMs, validStatusCodes);
if (validStatusCodes == null || validStatusCodes.isEmpty()) {
throw new IllegalArgumentException("No valid status codes supplied");
}
if (maximumRetries < 0) {
throw new IllegalArgumentException("Negative maximum retries not allowed");
}
if (retryWaitMs < 0) {
throw new IllegalArgumentException("Negative retry wait period not allowed");
}
this.validStatusCodes = new HashSet<>(REDIRECT_CODES);
this.validStatusCodes.addAll(validStatusCodes);
this.maximumRetries = maximumRetries;
this.retryWaitMs = retryWaitMs;
}
@Override
public boolean retryRequest(HttpResponse response, int executionCount, HttpContext context) {
int statusCode = response.getStatusLine().getStatusCode();
LOG.debug("RetryRequest nr {} for status {}", executionCount, statusCode);
if (executionCount > maximumRetries) {
return false;
}
return !validStatusCodes.contains(statusCode);
}
@Override
public long getRetryInterval() {
return retryWaitMs;
}
@Override
public boolean retryRequest(IOException exception, int executionCount, HttpContext context) {
LOG.debug("RetryRequest nr {} for exception {}", executionCount,
exception.getClass().getName());
return executionCount <= maximumRetries;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy