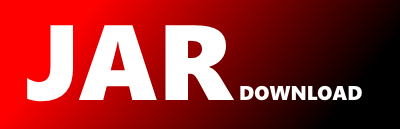
io.axual.utilities.config.providers.VaultKeyStoreProviderConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vault-keystore-provider Show documentation
Show all versions of vault-keystore-provider Show documentation
Provides an implementation of the Kafka ConfigProvider to retrieve private keys and
certificate chains from HashiCorp Vault and create files for keystores
The newest version!
package io.axual.utilities.config.providers;
/*-
* ========================LICENSE_START=================================
* Keystore Generation Configuration Provider
* %%
* Copyright (C) 2020 Axual B.V.
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* =========================LICENSE_END==================================
*/
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
import org.apache.kafka.common.config.ConfigDef;
import org.apache.kafka.common.config.types.Password;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class VaultKeyStoreProviderConfig extends VaultHelperConfig {
public static final Logger LOG = LoggerFactory.getLogger(VaultKeyStoreProviderConfig.class);
public static final String KEYSTORE_PRIVATE_KEY_KEYNAME_CONFIG =
"private.key.keyname";
static final String KEYSTORE_PRIVATE_KEY_KEYNAME_DEFAULT = "private.key";
private static final String KEYSTORE_PRIVATE_KEY_KEYNAME_DOC = "The name of the key used to store the private key PEM contents in Vault";
public static final String KEYSTORE_CERTIFICATE_CHAIN_KEYNAME_CONFIG =
"certificate.chain.keyname";
static final String KEYSTORE_CERTIFICATE_CHAIN_KEYNAME_DEFAULT = "certificate.chain";
private static final String KEYSTORE_CERTIFICATE_CHAIN_KEYNAME_DOC = "The name of the key used to store the certificate chain as PEM in Vault";
public static final String KEYSTORE_TEMPORARY_STORAGE_DIRECTORY_CONFIG =
"temporary.storage.dir";
static final String KEYSTORE_TEMPORARY_STORAGE_DIRECTORY_DEFAULT = System
.getProperty("java.io.tmpdir");
private static final String KEYSTORE_TEMPORARY_STORAGE_DIRECTORY_DOC = "The directory to store temporary files. If not set it will default to the JVM Temporary Directory";
public static final String KEYSTORE_TRUSTSTORE_LOCATION_CONFIG =
"truststore.location";
static final String KEYSTORE_TRUSTSTORE_LOCATION_DEFAULT = null;
private static final String KEYSTORE_TRUSTSTORE_LOCATION_DOC = "The path to the truststore file";
public static final String KEYSTORE_TRUSTSTORE_PASSWORD_CONFIG =
"truststore.password";
static final String KEYSTORE_TRUSTSTORE_PASSWORD_DEFAULT = null;
private static final String KEYSTORE_TRUSTSTORE_PASSWORD_DOC = "The password of the truststore file";
protected static final ConfigDef CONFIG_DEF = addVaultKeystoreProviderConfigDefinitions(
new ConfigDef());
private final Optional privateKeyKeyName;
private final Optional certificateChainKeyName;
private final Optional temporaryStorageDirectory;
private final Optional trustStoreLocation;
private final Optional trustStorePassword;
public VaultKeyStoreProviderConfig(Map, ?> originals) {
this(CONFIG_DEF, originals, false);
}
public VaultKeyStoreProviderConfig(Map, ?> originals, boolean doLog) {
this(CONFIG_DEF, originals, doLog);
}
public VaultKeyStoreProviderConfig(ConfigDef definition, Map, ?> originals) {
this(definition, originals, false);
}
public VaultKeyStoreProviderConfig(ConfigDef definition, Map, ?> originals, boolean doLog) {
super(verifyVaultKeystoreConfigProviderConfigDefinition(definition), originals, doLog);
privateKeyKeyName = getOptionalString(KEYSTORE_PRIVATE_KEY_KEYNAME_CONFIG);
certificateChainKeyName = getOptionalString(KEYSTORE_CERTIFICATE_CHAIN_KEYNAME_CONFIG);
temporaryStorageDirectory = getOptionalString(KEYSTORE_TEMPORARY_STORAGE_DIRECTORY_CONFIG);
trustStoreLocation = getOptionalString(KEYSTORE_TRUSTSTORE_LOCATION_CONFIG);
trustStorePassword = getOptionalPassword(KEYSTORE_TRUSTSTORE_PASSWORD_CONFIG);
}
public static ConfigDef addVaultKeystoreProviderConfigDefinitions(ConfigDef configDef) {
addVaultHelperConfigDefinitions(configDef)
.define(KEYSTORE_PRIVATE_KEY_KEYNAME_CONFIG, ConfigDef.Type.STRING,
KEYSTORE_PRIVATE_KEY_KEYNAME_DEFAULT, ConfigDef.Importance.HIGH,
KEYSTORE_PRIVATE_KEY_KEYNAME_DOC)
.define(KEYSTORE_CERTIFICATE_CHAIN_KEYNAME_CONFIG, ConfigDef.Type.STRING,
KEYSTORE_CERTIFICATE_CHAIN_KEYNAME_DEFAULT, ConfigDef.Importance.HIGH,
KEYSTORE_CERTIFICATE_CHAIN_KEYNAME_DOC)
.define(KEYSTORE_TEMPORARY_STORAGE_DIRECTORY_CONFIG, ConfigDef.Type.STRING,
KEYSTORE_TEMPORARY_STORAGE_DIRECTORY_DEFAULT, ConfigDef.Importance.LOW,
KEYSTORE_TEMPORARY_STORAGE_DIRECTORY_DOC)
.define(KEYSTORE_TRUSTSTORE_LOCATION_CONFIG, ConfigDef.Type.STRING,
KEYSTORE_TRUSTSTORE_LOCATION_DEFAULT, ConfigDef.Importance.HIGH,
KEYSTORE_TRUSTSTORE_LOCATION_DOC)
.define(KEYSTORE_TRUSTSTORE_PASSWORD_CONFIG, ConfigDef.Type.PASSWORD,
KEYSTORE_TRUSTSTORE_PASSWORD_DEFAULT, ConfigDef.Importance.HIGH,
KEYSTORE_TRUSTSTORE_PASSWORD_DOC);
return configDef;
}
protected static ConfigDef verifyVaultKeystoreConfigProviderConfigDefinition(
ConfigDef definition) {
Set configKeys = definition.names();
Set missing = CONFIG_DEF.names().stream()
.filter(name -> !configKeys.contains(name))
.collect(Collectors.toSet());
if (!missing.isEmpty()) {
throw new IllegalArgumentException(
"ConfigDef is missing configurations : " + String.join(", ", missing));
}
return definition;
}
public Optional getPrivateKeyKeyName() {
return privateKeyKeyName;
}
public Optional getCertificateChainKeyName() {
return certificateChainKeyName;
}
public Optional getTemporaryStorageDirectory() {
return temporaryStorageDirectory;
}
public Optional getTrustStoreLocation() {
return trustStoreLocation;
}
public Optional getTrustStorePassword() {
return trustStorePassword;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof VaultKeyStoreProviderConfig)) {
return false;
}
if (!super.equals(o)) {
return false;
}
VaultKeyStoreProviderConfig that = (VaultKeyStoreProviderConfig) o;
return privateKeyKeyName.equals(that.privateKeyKeyName) &&
certificateChainKeyName.equals(that.certificateChainKeyName) &&
temporaryStorageDirectory.equals(that.temporaryStorageDirectory) &&
trustStoreLocation.equals(that.trustStoreLocation) &&
trustStorePassword.equals(that.trustStorePassword);
}
@Override
public int hashCode() {
return Objects.hash(super.hashCode(), privateKeyKeyName, certificateChainKeyName,
temporaryStorageDirectory, trustStoreLocation, trustStorePassword);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy