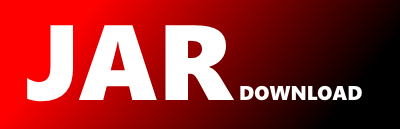
io.baltoro.remote.api.APIAuth Maven / Gradle / Ivy
package io.baltoro.remote.api;
import java.io.IOException;
import java.util.logging.Logger;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import javax.ws.rs.Consumes;
import javax.ws.rs.FormParam;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonMappingException;
import io.baltoro.domain.ObjectTypeEnum;
import io.baltoro.domain.User;
import io.baltoro.exception.NotFoundException;
import io.baltoro.exception.ServiceException;
import io.baltoro.service.Service;
import io.baltoro.service.ServiceFactory;
import io.baltoro.service.TOFactory;
import io.baltoro.to.APIError;
import io.baltoro.to.UserTO;
import io.baltoro.util.CryptoUtil;
@Path("/auth")
public class APIAuth
{
static Logger log = Logger.getLogger(APIAuth.class.getName());
void init()
{
}
@Path("/login")
@POST
@Produces(MediaType.APPLICATION_JSON)
@Consumes(MediaType.APPLICATION_FORM_URLENCODED)
public UserTO login(@FormParam("email") String email, @FormParam("password") String password,
@javax.ws.rs.core.Context HttpServletRequest request)
throws APIError,ServiceException, JsonParseException, JsonMappingException, IOException
{
Service service = ServiceFactory.getInstance();
User user = null;
try
{
user = (User) service.getByName(email, ObjectTypeEnum.USER);
String hash = user.getPasswordHash();
String salt = user.getPasswordSalt();
String inputHash = CryptoUtil.hash(salt+password);
if(!inputHash.equals(hash))
{
throw new APIError("email password not correct");
}
HttpSession session = request.getSession(true);
session.setAttribute("auth-user", user);
System.out.println(" login >>"+user.getBaseUuid()+" >> "+session.getId());
UserTO to = TOFactory.getTO(user, UserTO.class);
return to;
}
catch(APIError e)
{
throw e;
}
catch(NotFoundException e)
{
throw new APIError("email not correct");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy