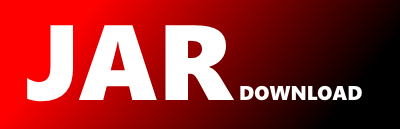
io.baltoro.service.ServiceImpl Maven / Gradle / Ivy
package io.baltoro.service;
import java.io.ByteArrayInputStream;
import java.io.InputStream;
import java.security.KeyFactory;
import java.security.cert.CertificateFactory;
import java.security.cert.X509Certificate;
import java.security.interfaces.RSAPrivateKey;
import java.security.spec.PKCS8EncodedKeySpec;
import java.sql.Timestamp;
import java.util.List;
import java.util.logging.Logger;
import io.baltoro.domain.Alias;
import io.baltoro.domain.App;
import io.baltoro.domain.BO;
import io.baltoro.domain.BODefaults;
import io.baltoro.domain.BaltoroInstance;
import io.baltoro.domain.Container;
import io.baltoro.domain.ObjectTypeEnum;
import io.baltoro.domain.Permission;
import io.baltoro.domain.PermissionTypeEnum;
import io.baltoro.domain.PrivateData;
import io.baltoro.domain.StateTypeEnum;
import io.baltoro.domain.User;
import io.baltoro.exception.NotFoundException;
import io.baltoro.exception.ObjectExistsException;
import io.baltoro.exception.ReadNotAllowedException;
import io.baltoro.exception.ServiceException;
import io.baltoro.to.APIError;
import io.baltoro.to.Keys;
import io.baltoro.util.CryptoUtil;
import io.baltoro.util.ObjectUtil;
import io.baltoro.util.StringUtil;
import io.baltoro.util.UUIDGenerator;
public class ServiceImpl implements Service
{
protected static final Logger log = Logger.getLogger(ServiceImpl.class.getName());
public T get(String baseUuid, Class type)
throws ServiceException,NotFoundException,ReadNotAllowedException
{
BO obj = BOCache.instance().get(baseUuid);
if(obj != null)
{
Permission p = obj.getPermission(Ctx.getUserUuid());
if(p == null)
{
Dao dao = DaoFactory.getInstance();
p = dao.getPermission(baseUuid);
obj.addPermission(Ctx.getUserUuid(), p);
if(!p.isRead() || (p instanceof NullPermission))
{
throw new ReadNotAllowedException(baseUuid, Ctx.getUserUuid());
}
}
System.out.println("!!!!!!["+baseUuid+"]!!!!!!! from cache");
return type.cast(obj);
}
Dao dao = DaoFactory.getInstance();
BO bo = dao.getBOByBaseUuid(baseUuid);
BOCache.instance().add(baseUuid, bo);
return type.cast(bo);
}
public BO get(String baseUuid)
throws ServiceException,NotFoundException,ReadNotAllowedException
{
BO obj = BOCache.instance().get(baseUuid);
if(obj != null)
{
Permission p = obj.getPermission(Ctx.getUserUuid());
if(p == null)
{
Dao dao = DaoFactory.getInstance();
p = dao.getPermission(baseUuid);
obj.addPermission(Ctx.getUserUuid(), p);
if(!p.isRead() || (p instanceof NullPermission))
{
throw new ReadNotAllowedException(baseUuid, Ctx.getUserUuid());
}
}
return obj;
}
Dao dao = DaoFactory.getInstance();
BO bo = dao.getBOByBaseUuid(baseUuid);
BOCache.instance().add(baseUuid, bo);
return bo;
}
public List getBOs(List uuids)
throws ServiceException,NotFoundException,ReadNotAllowedException
{
Dao dao = DaoFactory.getInstance();
List bos = dao.getBOs(uuids);
return bos;
}
public List findBOByName(String name, ObjectTypeEnum type, String containerUuid)
throws ServiceException
{
Dao dao = DaoFactory.getInstance();
return dao.findBOByName(name, type, containerUuid);
}
public List find(String name, ObjectTypeEnum type)
throws ServiceException
{
Dao dao = DaoFactory.getInstance();
return dao.find(name, type);
}
/*
public BO getByName(String name, ObjectTypeEnum type, String containerUuid)
throws ServiceException,NotFoundException
{
Dao dao = DaoFactory.getInstance();
List list = dao.findBOByName(name, type, containerUuid);
if(list == null || list.isEmpty())
{
throw new NotFoundException("record not found");
}
else
{
return list.get(0);
}
}
*/
public BO getByName(String name, ObjectTypeEnum type)
{
BO bo = BOCache.instance().getByName(name, type);
try
{
if(bo != null)
{
Permission p = bo.getPermission(Ctx.getUserUuid());
if(p == null)
{
Dao dao = DaoFactory.getInstance();
p = dao.getPermission(bo.getBaseUuid());
bo.addPermission(Ctx.getUserUuid(), p);
if(!p.isRead() || (p instanceof NullPermission))
{
throw new ReadNotAllowedException(bo.getBaseUuid(), Ctx.getUserUuid());
}
}
System.out.println(" ))))))))))))))))))) from cache by name : "+name);
return bo;
}
Dao dao = DaoFactory.getInstance();
List list = dao.find(name, type);
if(list == null || list.isEmpty())
{
list = dao.find(name, ObjectTypeEnum.ALIS);
if(list == null || list.isEmpty())
{
throw new NotFoundException("record not found");
}
else
{
Alias alias = (Alias) list.get(0);
BOCache.instance().add(alias.getBaseUuid(), alias);
String baseUuid = alias.getSourceUuid();
bo = get(baseUuid);
}
}
else
{
bo = (BO)list.get(0);
}
BOCache.instance().add(bo.getBaseUuid(), bo);
return bo;
}
catch (Exception e)
{
e.printStackTrace();
}
return null;
}
public List findBOByMetadata(String defUuid, String mdName, String value)
throws ServiceException,NotFoundException,ReadNotAllowedException
{
Dao dao = DaoFactory.getInstance();
//BODef def = dao.getDefByBaseUuid(defUuid);
//MetadataMap map = def.getMetadata(mdName);
return null;// dao.findBOByMetadata(map.getColumnName(), value);
}
public void saveBODef(BODefaults def)
throws ServiceException
{
Dao dao = DaoFactory.getInstance();
//dao.saveObjectDef(def);
}
public T createBO(String name, ObjectTypeEnum type)
throws ServiceException
{
//PermissionResolver.checkCreateAllowed(def.getBaseUuid());
BO bo = null;
//ObjectTypeEnum type = ObjectTypeEnum.getInstanceType(def.getObjectType());
String baseUuid = UUIDGenerator.uuid(type);
String versionUuid = UUIDGenerator.uuid(type);
String containerUuid = BODefaults.BASE_CONTAINER;
boolean checkUniqueName = false;
bo = ObjectUtil.initBOByType(type);
if(bo == null)
{
throw new ServiceException(type+" not supported");
}
if(bo instanceof Container)
{
containerUuid = baseUuid;
}
else if(bo instanceof User || bo instanceof App)
{
containerUuid = BODefaults.BASE_CONTAINER;
checkUniqueName = true;
}
else if(type == ObjectTypeEnum.APPW)
{
}
if(checkUniqueName)
{
Dao dao = DaoFactory.getInstance();
boolean exits = dao.objectExists(name, containerUuid, type);
if(exits)
{
throw new ObjectExistsException(name+" object exsits already");
}
}
bo.setBaseUuid(baseUuid);
bo.setVersionUuid(versionUuid);
bo.setVersionNumber(1);
bo.setLatestVersionUuid(versionUuid);
bo.setObjectType(type.toString());
bo.setName(name);
bo.setState(StateTypeEnum.LIVE.toString());
bo.setContainerUuid(containerUuid);
bo.setPermissionType(PermissionTypeEnum.CONT.toString());
bo.setCreatedBy(Ctx.getUserUuid());
bo.setCreatedOn(new Timestamp(System.currentTimeMillis()));
return (T)bo;
}
public void deleteBO(String baseUuid)
{
Dao dao = DaoFactory.getInstance();
try
{
dao.deleteBO(baseUuid);
}
catch (ServiceException e)
{
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public void saveBO(BO bo)
throws ServiceException
{
User user = Ctx.getUser();
String domain = null;
if(bo instanceof Container)
{
//domain = StringUtil.getDomainFromEmail(user.getName());
}
Dao dao = DaoFactory.getInstance();
dao.save(bo);
Permission permission = Permission.initOwnerPermission(bo.getBaseUuid(), user.getBaseUuid());
dao.savePermissions(permission);
if(bo.getBaseUuid().equals(BODefaults.BASE_CONTAINER) || bo.getBaseUuid().equals(BODefaults.BASE_USER))
{
System.out.println(bo.getBaseUuid()+" permission for base objects : do nothing ......");
}
else
{
//permission = Permission.initReadPermission(bo.getBaseUuid(), BODefaults.BASE_USER);
//dao.savePermissions(permission);
}
dao.saveMetadata(bo);
if(bo instanceof Container)
{
permission = Permission.initReadPermission(bo.getBaseUuid(), bo.getBaseUuid());
dao.savePermissions(permission);
dao.saveRelationship(bo.getBaseUuid(), Ctx.getUserUuid());
}
}
public void updateBO(BO bo)
throws ServiceException
{
//signBO(bo);
Dao dao = DaoFactory.getInstance();
dao.save(bo);
dao.deleteMetadata(bo);
dao.saveMetadata(bo);
}
public void savePermissions(Permission permission)
throws ServiceException
{
Dao dao = DaoFactory.getInstance();
dao.savePermissions(permission);
}
private void createRelation(String pBaseUuid, String cBaseUuid)
throws ServiceException
{
Dao dao = DaoFactory.getInstance();
dao.saveRelationship(pBaseUuid, cBaseUuid);
}
public List findChildrenByType(String baseUuid, ObjectTypeEnum type)
throws ServiceException
{
Dao dao = DaoFactory.getInstance();
List children = dao.findChildrenByType(baseUuid, type);
return children;
}
public List findByStateType(String state, ObjectTypeEnum type, String containerUuid)
throws ServiceException
{
Dao dao = DaoFactory.getInstance();
List list = dao.findByStateType(state, type, containerUuid);
return list;
}
public int countByStateType(String state, ObjectTypeEnum type, String containerUuid)
throws ServiceException
{
Dao dao = DaoFactory.getInstance();
int count = dao.countByStateType(state, type, containerUuid);
return count;
}
public Container createContainer(String name) throws ServiceException
{
try
{
Container baseContainer = get(BODefaults.BASE_CONTAINER, Container.class);
byte[] caBytes = StringUtil.decode(baseContainer.getCa());
InputStream in = new ByteArrayInputStream(caBytes);
CertificateFactory fact = CertificateFactory.getInstance("X.509","BC");
X509Certificate rootCert = (X509Certificate)fact.generateCertificate(in);
byte[] keyBytes = StringUtil.decode(baseContainer.getPrivateKey());
PKCS8EncodedKeySpec spec = new PKCS8EncodedKeySpec(keyBytes);
KeyFactory rsaFact = KeyFactory.getInstance("RSA","BC");
RSAPrivateKey caKey = (RSAPrivateKey) rsaFact.generatePrivate(spec);
Keys keys = CryptoUtil.generateKeys();
//X509Certificate containerCert = CryptoUtil.signCert(keys.keypair, name, rootCert, caKey);
Container container = (Container) createBO(name, ObjectTypeEnum.CONT);
container.setPublicKey(keys.getPublicKey());
container.setPrivateKey(keys.getPrivateKey());
//container.setCa(StringUtil.encode(containerCert.getEncoded()));
saveBO(container);
return container;
}
catch (Exception e)
{
throw new ServiceException(e);
}
}
public Alias createAlias(String name, BO bo) throws ServiceException
{
ObjectTypeEnum type = ObjectTypeEnum.valueOf(bo.getObjectType());
List apps = (List)find(name, type);
if(apps != null && apps.size() > 0)
{
throw new ServiceException(name+" already exists");
}
List aliass = (List)find(name, ObjectTypeEnum.ALIS);
for (Alias alias : aliass)
{
if(alias.getSourceType().equals(bo.getObjectType()))
{
throw new ServiceException(name+" already exists");
}
}
Alias alias = (Alias) createBO(name, ObjectTypeEnum.ALIS);
alias.setSourceUuid(bo.getBaseUuid());
alias.setSourceType(bo.getObjectType());
saveBO(alias);
Permission p = Permission.initReadPermission(alias.getBaseUuid(), BODefaults.BASE_USER);
savePermissions(p);
createRelation(bo.getBaseUuid(), alias.getBaseUuid());
//BOCache.instance().add(alias.getBaseUuid(), alias);
return alias;
}
public App createApp(String name) throws ServiceException
{
try
{
App app = null;
try
{
app = (App) getByName(name, ObjectTypeEnum.APPW);
String error = name+" already exists";
List aliass = (List)find(name, ObjectTypeEnum.ALIS);
for (Alias alias : aliass)
{
if(alias.getSourceType().equals(ObjectTypeEnum.APPW))
{
error = name+" already exists";
break;
}
}
log.info(error);
throw new APIError(error);
}
catch (NotFoundException e)
{
log.info("app with name : "+name+" doesn't exists");
}
/*
Container baseContainer = get(BODefaults.BASE_CONTAINER, Container.class);
byte[] caBytes = StringUtil.decode(baseContainer.getCa());
InputStream in = new ByteArrayInputStream(caBytes);
CertificateFactory fact = CertificateFactory.getInstance("X.509","BC");
X509Certificate rootCert = (X509Certificate)fact.generateCertificate(in);
byte[] keyBytes = StringUtil.decode(baseContainer.getPrivateKey());
PKCS8EncodedKeySpec spec = new PKCS8EncodedKeySpec(keyBytes);
KeyFactory rsaFact = KeyFactory.getInstance("RSA","BC");
RSAPrivateKey caKey = (RSAPrivateKey) rsaFact.generatePrivate(spec);
X509Certificate appCert = CryptoUtil.signCert(keys.keypair, name, rootCert, caKey);
*/
app = (App) createBO(name, ObjectTypeEnum.APPW);
Keys keys = CryptoUtil.generateKeys();
app.setPublicKey(keys.getPublicKey());
//app.setPrivateKey(keys.getPrivateKey());
PrivateData pd = createBO(app.getName()+"-private data", ObjectTypeEnum.PRVD);
pd.setPrivateKey(keys.getPrivateKey());
pd.setPassword("password");
app.setPrivateDataUuid(pd.getBaseUuid());
saveBO(app);
saveBO(pd);
User user = Ctx.getUser();
createRelation(user.getBaseUuid(), app.getBaseUuid());
savePermissions(Permission.initReadPermission(app.getBaseUuid(), BODefaults.BASE_USER));
return app;
}
catch (Exception e)
{
throw new ServiceException(e);
}
}
public User createUser(String email, String password)
throws ServiceException
{
User user = (User) getByName(email, ObjectTypeEnum.USER);
if(user != null)
{
String error = email+" user already exists";
log.info(error);
throw new APIError(error);
}
user = (User) createBO(email, ObjectTypeEnum.USER);
user.setEmail(email);
String salt = UUIDGenerator.randomString(5);
String passwordHash = CryptoUtil.hash(salt+password);
user.setPasswordSalt(salt);
user.setPasswordHash(passwordHash);
user.setContainerUuid(BODefaults.BASE_CONTAINER);
saveBO(user);
System.out.println(user.getBaseUuid()+", "+user.getCreatedBy());
return user;
}
public BaltoroInstance getInstance(String uuid) throws ServiceException
{
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy