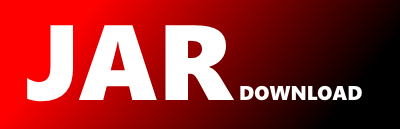
io.baltoro.remote.BaltoroReqProcessor Maven / Gradle / Ivy
package io.baltoro.remote;
import java.util.HashMap;
import java.util.Map;
import java.util.Queue;
import java.util.Timer;
import java.util.TimerTask;
import java.util.concurrent.ConcurrentLinkedDeque;
import io.baltoro.domain.BaltoroInstanceRequest;
import io.baltoro.service.InstanceService;
import io.baltoro.service.ServiceFactory;
public class BaltoroReqProcessor
{
private static BaltoroReqProcessor processor;
private Queue queue = new ConcurrentLinkedDeque();
private Queue updateQueue = new ConcurrentLinkedDeque();
private Map map = new HashMap<>(2000);
private InstanceService service = ServiceFactory.get(InstanceService.class);
private BaltoroReqProcessor()
{
Timer insertTimer = new Timer();
insertTimer.schedule(new TimerTask()
{
@Override
public void run()
{
try
{
BaltoroInstanceRequest req;
while((req = queue.poll()) != null)
{
service.touchAppUserSession(req.getBltSessionId());
service.insert(req);
map.put(req.getUuid(), req.getMillisTaken());
}
}
catch (Exception e)
{
e.printStackTrace();
}
}
}, 0,4000);
Timer updateTimer = new Timer();
updateTimer.schedule(new TimerTask()
{
@Override
public void run()
{
try
{
BaltoroInstanceRequest req;
while((req = updateQueue.poll()) != null)
{
service.updateRequestTime(req.getUuid(), req.getMillisTaken(), req.getSizeKB(), req.getError());
}
}
catch (Exception e)
{
e.printStackTrace();
}
}
}, 0,4000);
}
static BaltoroReqProcessor get()
{
if(processor == null)
{
processor = new BaltoroReqProcessor();
}
return processor;
}
public void add(BaltoroInstanceRequest req)
{
queue.add(req);
}
public void update(BaltoroInstanceRequest req)
{
Integer millis = map.get(req.getUuid());
if(millis == null)
{
// do nothing
}
else if(millis.intValue() == req.getMillisTaken())
{
map.remove(req.getUuid());
}
else
{
map.remove(req.getUuid());
updateQueue.add(req);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy