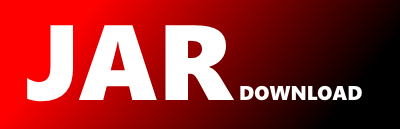
io.baltoro.remote.InstancePoller Maven / Gradle / Ivy
package io.baltoro.remote;
import java.util.concurrent.ConcurrentLinkedQueue;
import io.baltoro.exception.NoRunningInstanceException;
import io.baltoro.to.WSTO;
public class InstancePoller extends Thread
{
private String appUuid;
boolean run = true;
private String sync;
private long t;
ConcurrentLinkedQueue queue;
static int count;
public InstancePoller(String appUuid)
{
count++;
this.appUuid = appUuid;
sync = appUuid+"-queue";
queue = new ConcurrentLinkedQueue<>();
System.out.println("Starting Poller For "+appUuid+", count ("+count+")");
}
void add(WSTO to)
{
queue.add(to);
synchronized (sync.intern())
{
sync.intern().notify();
}
}
@Override
public void run()
{
while(run)
{
t = System.currentTimeMillis();
if(!WSSessions.get().isRunning(appUuid))
{
System.out.println("1 No running instance for "+appUuid+", closing the polling thread ");
break;
}
if(queue == null || queue.size() == 0)
{
//wait(appUuid+" queue is empty !");
wait(null);
continue;
}
WSTO to = queue.peek();
if(to == null)
{
wait(appUuid+" no items in queue !");
continue;
}
SessionInstance session;
try
{
session = WSSessions.get().getSessionForWorker(appUuid, to.instanceUuid);
}
catch (NoRunningInstanceException e)
{
System.out.println("2 No running instance for "+appUuid+", closing the polling thread ");
break;
}
if(session == null)
{
String apiPath = null;
if(to.webSocketContext != null)
{
apiPath = to.webSocketContext.getApiPath();
}
else
{
apiPath = to.requestContext.getApiPath();
}
wait(appUuid+" >>>>> NO free ws session ! waiting .... "+apiPath);
continue;
}
to = queue.poll();
/*
if(to.webSocketContext != null)
{
byte[] data = to.webSocketContext.getData();
if(data != null)
{
int f = data[0];
int s = data[1];
System.out.println(" >>> ====> frame ("+f+") , seg("+s+") len("+data.length+") "+this);
}
}
*/
//System.out.println(" WorkerPool : "+WorkerPool.info());
RequestWorker worker = WorkerPool.get();
if(worker == null)
{
worker = new RequestWorker();
worker.start();
}
worker.set(to, session);
}
System.out.println("Poller Thread stoped for ("+appUuid+"), count("+count+")");
run = false;
}
private void wait(String text)
{
try
{
long t0 = System.currentTimeMillis();
synchronized (sync.intern())
{
//System.out.println(text);
sync.intern().wait(50000);
long t1 = System.currentTimeMillis();
long t = t1-t0;
if(text != null && t > 0)
{
System.out.println("Server waited : "+t+" : "+text);
}
}
}
catch (Exception e)
{
e.printStackTrace();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy