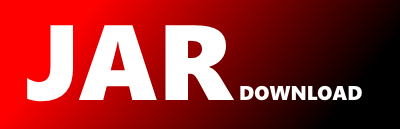
io.baltoro.remote.WSProbe1 Maven / Gradle / Ivy
package io.baltoro.remote;
import java.io.ByteArrayOutputStream;
import java.nio.ByteBuffer;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardOpenOption;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import java.util.logging.Logger;
import javax.servlet.http.HttpSession;
import javax.websocket.EndpointConfig;
import javax.websocket.OnClose;
import javax.websocket.OnMessage;
import javax.websocket.OnOpen;
import javax.websocket.Session;
import javax.websocket.server.ServerEndpoint;
import org.apache.tomcat.websocket.WsSession;
@ServerEndpoint(value = "/probe1")
public class WSProbe1
{
static Logger log = Logger.getLogger(WSProbe1.class.getSimpleName());
private static int limit = 10;
private int index;
String deviceUuid;
Path path;
byte[] spacerBytes = {100,100,100,100,100,100,100,100};
byte[] lastFrameBytes;
int lastFrame = -1;
int _lastFrame = -1;
int currentFrame = -1;
int _lastFrameCount = 0;
static Map probeMap = new HashMap<>(100);
static Map examMap = new HashMap<>(100);
//List list = new ArrayList<>(limit);
private Map> frameMap = new HashMap<>(limit);
WsSession session;
byte[] segs;
int segDataPos = 0;
@OnOpen
public void onOpen(Session _session, EndpointConfig config)
{
this.session = (WsSession) _session;
HttpSession httpSession = HTTPSessions.getSession(session.getHttpSessionId());
if(httpSession == null)
{
System.out.println("restart browser ... httpsession is null");
}
deviceUuid = (String) httpSession.getAttribute("deviceUuid");
lastFrame = -1;
System.out.println("Open Probe WS Connection ... deviceUuid : "+deviceUuid);
if(deviceUuid != null)
{
probeMap.put(deviceUuid, this);
}
}
private void checkFile(String examUuid)
{
if(path != null && path.endsWith(examUuid+".dat"))
{
return;
}
String dir = System.getProperty("user.home")+"/exams";
path = Paths.get(dir+"/"+examUuid+".dat");
try
{
if (!Files.exists(path))
{
Files.createFile(path);
}
else
{
Files.delete(path);
Files.createFile(path);
}
}
catch (Exception e)
{
e.printStackTrace();
}
}
void sendMessage(String text)
{
if(session == null)
{
System.out.println(" live session is null");
return;
}
if(text==null || text.equals("stop"))
{
//WSProbe1.liveSession.close();
return;
}
session.getAsyncRemote().sendText(text);
}
@OnClose
public void onClose(Session session)
{
System.out.println("Close Probe WS Connection ... ");
index = -1;
}
int dr = 0;
long t0 = System.currentTimeMillis();
@OnMessage
public void onMessage(ByteBuffer buffer, Session _session) throws Exception
{
byte[] data = buffer.array();
String examUuid = examMap.get(deviceUuid);
if(examUuid == null)
{
System.out.println("EXAM UUID NULL.................... data received index("+index+") len("+data.length+")");
//int f = data[0] & 0xFF;
//int s = data[1] & 0xFF;
//System.out.println("adding data to frame("+f+"), seg("+s+"), index("+index+") len("+data.length+")");
//System.out.println(" no exmaUUId >>>>>>>>>>>>>>>>> "+data.length+"- "+this+" ,,, "+deviceUuid);
//session.send(data);
//session.getBasicRemote().sendBinary(ByteBuffer.wrap(new byte[]{1}));
return;
}
int segs = data.length/525;
for (int i = 0; i < segs; i++)
{
int index = (i*525)+8;
byte[] segment = Arrays.copyOfRange(data, index, index+514);
int f = segment[0] & 0xFF;
int s = segment[1] & 0xFF;
//System.out.println("len="+segment.length+",index="+index+" frame="+f+", seg="+s+", l1="+l1+",l2="+l2+",l3="+l3);
//System.out.println("frame="+f+", seg="+s);
index = f % limit;
Map segMap = frameMap.get(index);
if(segMap == null)
{
String key = f+"-segment-map";
synchronized (key.intern())
{
segMap = frameMap.get(index);
if(segMap == null)
{
segMap = new HashMap<>(160);
frameMap.put(index, segMap);
}
}
}
segMap.put(s, segment);
if(_lastFrame == -1)
{
_lastFrame = f;
_lastFrameCount = 0;
}
if(f != _lastFrame && _lastFrameCount < 4)
{
_lastFrameCount++;
//System.out.println(">>>>>>>>>>>> _lastFrame="+_lastFrame+", count="+_lastFrameCount);
}
if(f == _lastFrame)
{
_lastFrameCount = 0;
}
if(_lastFrameCount > 3)
{
//System.out.println("*********** processing frame ="+_lastFrame+", count="+_lastFrameCount);
processFrame(_lastFrame, examUuid);
_lastFrame = f;
_lastFrameCount = 0;
}
}
//System.out.println("adding data to frame("+f+"), seg("+s+"), index("+index+") len("+data.length+")");
}
void processFrame(int frameNumber, String examUuid) throws Exception
{
frameNumber = frameNumber % limit;
ByteArrayOutputStream out = new ByteArrayOutputStream(85*1000);
Map segMap = frameMap.get(frameNumber);
if(segMap == null)
{
System.err.println(" >>>>>>>>>>>>>>>>>>>> &&&&&&&&&&&&&&& ********************* segMap NULL : "+frameNumber);
return;
}
for (int i = 0; i < segMap.keySet().size(); i++)
{
byte[] segBytes = segMap.get(i);
if(segBytes != null)
{
out.write(segBytes);
}
}
this.lastFrameBytes = out.toByteArray();
this.lastFrame = frameNumber;
System.err.println(" >>>>>>>>>>>>>>>>>>>> &&&&&&&&&&&&&&& ********************* frame : "+frameNumber+", bytes : "+lastFrameBytes.length);
String key = examUuid+"-sync";
synchronized (key.intern())
{
key.intern().notify();
}
///*
checkFile(examUuid);
if(path != null)
{
Files.write(path, lastFrameBytes, StandardOpenOption.APPEND);
Files.write(path, spacerBytes, StandardOpenOption.APPEND);
}
//*/
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy